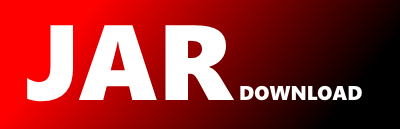
com.pulumi.azurenative.media.LiveOutput Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.media.LiveOutputArgs;
import com.pulumi.azurenative.media.outputs.HlsResponse;
import com.pulumi.azurenative.media.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The Live Output.
* Azure REST API version: 2022-11-01. Prior API version in Azure Native 1.x: 2020-05-01.
*
* ## Example Usage
* ### Create a LiveOutput
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.media.LiveOutput;
* import com.pulumi.azurenative.media.LiveOutputArgs;
* import com.pulumi.azurenative.media.inputs.HlsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var liveOutput = new LiveOutput("liveOutput", LiveOutputArgs.builder()
* .accountName("slitestmedia10")
* .archiveWindowLength("PT5M")
* .assetName("6f3264f5-a189-48b4-a29a-a40f22575212")
* .description("test live output 1")
* .hls(HlsArgs.builder()
* .fragmentsPerTsSegment(5)
* .build())
* .liveEventName("myLiveEvent1")
* .liveOutputName("myLiveOutput1")
* .manifestName("testmanifest")
* .resourceGroupName("mediaresources")
* .rewindWindowLength("PT4M")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:media:LiveOutput myLiveOutput1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Media/mediaservices/{accountName}/liveEvents/{liveEventName}/liveOutputs/{liveOutputName}
* ```
*
*/
@ResourceType(type="azure-native:media:LiveOutput")
public class LiveOutput extends com.pulumi.resources.CustomResource {
/**
* ISO 8601 time between 1 minute to 25 hours to indicate the maximum content length that can be archived in the asset for this live output. This also sets the maximum content length for the rewind window. For example, use PT1H30M to indicate 1 hour and 30 minutes of archive window.
*
*/
@Export(name="archiveWindowLength", refs={String.class}, tree="[0]")
private Output archiveWindowLength;
/**
* @return ISO 8601 time between 1 minute to 25 hours to indicate the maximum content length that can be archived in the asset for this live output. This also sets the maximum content length for the rewind window. For example, use PT1H30M to indicate 1 hour and 30 minutes of archive window.
*
*/
public Output archiveWindowLength() {
return this.archiveWindowLength;
}
/**
* The asset that the live output will write to.
*
*/
@Export(name="assetName", refs={String.class}, tree="[0]")
private Output assetName;
/**
* @return The asset that the live output will write to.
*
*/
public Output assetName() {
return this.assetName;
}
/**
* The creation time the live output.
*
*/
@Export(name="created", refs={String.class}, tree="[0]")
private Output created;
/**
* @return The creation time the live output.
*
*/
public Output created() {
return this.created;
}
/**
* The description of the live output.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return The description of the live output.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* HTTP Live Streaming (HLS) packing setting for the live output.
*
*/
@Export(name="hls", refs={HlsResponse.class}, tree="[0]")
private Output* @Nullable */ HlsResponse> hls;
/**
* @return HTTP Live Streaming (HLS) packing setting for the live output.
*
*/
public Output> hls() {
return Codegen.optional(this.hls);
}
/**
* The time the live output was last modified.
*
*/
@Export(name="lastModified", refs={String.class}, tree="[0]")
private Output lastModified;
/**
* @return The time the live output was last modified.
*
*/
public Output lastModified() {
return this.lastModified;
}
/**
* The manifest file name. If not provided, the service will generate one automatically.
*
*/
@Export(name="manifestName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> manifestName;
/**
* @return The manifest file name. If not provided, the service will generate one automatically.
*
*/
public Output> manifestName() {
return Codegen.optional(this.manifestName);
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* The initial timestamp that the live output will start at, any content before this value will not be archived.
*
*/
@Export(name="outputSnapTime", refs={Double.class}, tree="[0]")
private Output* @Nullable */ Double> outputSnapTime;
/**
* @return The initial timestamp that the live output will start at, any content before this value will not be archived.
*
*/
public Output> outputSnapTime() {
return Codegen.optional(this.outputSnapTime);
}
/**
* The provisioning state of the live output.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The provisioning state of the live output.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* The resource state of the live output.
*
*/
@Export(name="resourceState", refs={String.class}, tree="[0]")
private Output resourceState;
/**
* @return The resource state of the live output.
*
*/
public Output resourceState() {
return this.resourceState;
}
/**
* ISO 8601 time between 1 minute to the duration of archiveWindowLength to control seek-able window length during Live. The service won't use this property once LiveOutput stops. The archived VOD will have full content with original ArchiveWindowLength. For example, use PT1H30M to indicate 1 hour and 30 minutes of rewind window length. Service will use implicit default value 30m only if Live Event enables LL.
*
*/
@Export(name="rewindWindowLength", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> rewindWindowLength;
/**
* @return ISO 8601 time between 1 minute to the duration of archiveWindowLength to control seek-able window length during Live. The service won't use this property once LiveOutput stops. The archived VOD will have full content with original ArchiveWindowLength. For example, use PT1H30M to indicate 1 hour and 30 minutes of rewind window length. Service will use implicit default value 30m only if Live Event enables LL.
*
*/
public Output> rewindWindowLength() {
return Codegen.optional(this.rewindWindowLength);
}
/**
* The system metadata relating to this resource.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return The system metadata relating to this resource.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public LiveOutput(java.lang.String name) {
this(name, LiveOutputArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public LiveOutput(java.lang.String name, LiveOutputArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public LiveOutput(java.lang.String name, LiveOutputArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:media:LiveOutput", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private LiveOutput(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:media:LiveOutput", name, null, makeResourceOptions(options, id), false);
}
private static LiveOutputArgs makeArgs(LiveOutputArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? LiveOutputArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:media/v20180330preview:LiveOutput").build()),
Output.of(Alias.builder().type("azure-native:media/v20180601preview:LiveOutput").build()),
Output.of(Alias.builder().type("azure-native:media/v20180701:LiveOutput").build()),
Output.of(Alias.builder().type("azure-native:media/v20190501preview:LiveOutput").build()),
Output.of(Alias.builder().type("azure-native:media/v20200501:LiveOutput").build()),
Output.of(Alias.builder().type("azure-native:media/v20210601:LiveOutput").build()),
Output.of(Alias.builder().type("azure-native:media/v20211101:LiveOutput").build()),
Output.of(Alias.builder().type("azure-native:media/v20220801:LiveOutput").build()),
Output.of(Alias.builder().type("azure-native:media/v20221101:LiveOutput").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static LiveOutput get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new LiveOutput(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy