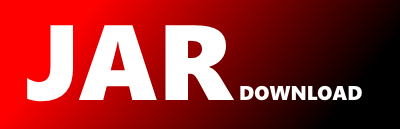
com.pulumi.azurenative.media.inputs.ContentKeyPolicyPlayReadyPlayRightArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media.inputs;
import com.pulumi.azurenative.media.enums.ContentKeyPolicyPlayReadyUnknownOutputPassingOption;
import com.pulumi.azurenative.media.inputs.ContentKeyPolicyPlayReadyExplicitAnalogTelevisionRestrictionArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Configures the Play Right in the PlayReady license.
*
*/
public final class ContentKeyPolicyPlayReadyPlayRightArgs extends com.pulumi.resources.ResourceArgs {
public static final ContentKeyPolicyPlayReadyPlayRightArgs Empty = new ContentKeyPolicyPlayReadyPlayRightArgs();
/**
* Configures Automatic Gain Control (AGC) and Color Stripe in the license. Must be between 0 and 3 inclusive.
*
*/
@Import(name="agcAndColorStripeRestriction")
private @Nullable Output agcAndColorStripeRestriction;
/**
* @return Configures Automatic Gain Control (AGC) and Color Stripe in the license. Must be between 0 and 3 inclusive.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy