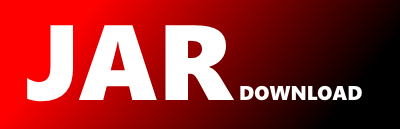
com.pulumi.azurenative.media.inputs.SelectAudioTrackByAttributeArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media.inputs;
import com.pulumi.azurenative.media.enums.AttributeFilter;
import com.pulumi.azurenative.media.enums.ChannelMapping;
import com.pulumi.azurenative.media.enums.TrackAttribute;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Select audio tracks from the input by specifying an attribute and an attribute filter.
*
*/
public final class SelectAudioTrackByAttributeArgs extends com.pulumi.resources.ResourceArgs {
public static final SelectAudioTrackByAttributeArgs Empty = new SelectAudioTrackByAttributeArgs();
/**
* The TrackAttribute to filter the tracks by.
*
*/
@Import(name="attribute", required=true)
private Output> attribute;
/**
* @return The TrackAttribute to filter the tracks by.
*
*/
public Output> attribute() {
return this.attribute;
}
/**
* Optional designation for single channel audio tracks. Can be used to combine the tracks into stereo or multi-channel audio tracks.
*
*/
@Import(name="channelMapping")
private @Nullable Output> channelMapping;
/**
* @return Optional designation for single channel audio tracks. Can be used to combine the tracks into stereo or multi-channel audio tracks.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy