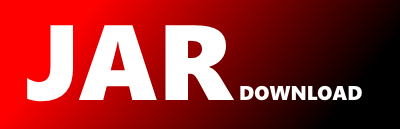
com.pulumi.azurenative.media.outputs.ContentKeyPolicyFairPlayConfigurationResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media.outputs;
import com.pulumi.azurenative.media.outputs.ContentKeyPolicyFairPlayOfflineRentalConfigurationResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ContentKeyPolicyFairPlayConfigurationResponse {
/**
* @return The key that must be used as FairPlay Application Secret key. This needs to be base64 encoded.
*
*/
private String ask;
/**
* @return The Base64 representation of FairPlay certificate in PKCS 12 (pfx) format (including private key).
*
*/
private String fairPlayPfx;
/**
* @return The password encrypting FairPlay certificate in PKCS 12 (pfx) format.
*
*/
private String fairPlayPfxPassword;
/**
* @return The discriminator for derived types.
* Expected value is '#Microsoft.Media.ContentKeyPolicyFairPlayConfiguration'.
*
*/
private String odataType;
/**
* @return Offline rental policy
*
*/
private @Nullable ContentKeyPolicyFairPlayOfflineRentalConfigurationResponse offlineRentalConfiguration;
/**
* @return The rental and lease key type.
*
*/
private String rentalAndLeaseKeyType;
/**
* @return The rental duration. Must be greater than or equal to 0.
*
*/
private Double rentalDuration;
private ContentKeyPolicyFairPlayConfigurationResponse() {}
/**
* @return The key that must be used as FairPlay Application Secret key. This needs to be base64 encoded.
*
*/
public String ask() {
return this.ask;
}
/**
* @return The Base64 representation of FairPlay certificate in PKCS 12 (pfx) format (including private key).
*
*/
public String fairPlayPfx() {
return this.fairPlayPfx;
}
/**
* @return The password encrypting FairPlay certificate in PKCS 12 (pfx) format.
*
*/
public String fairPlayPfxPassword() {
return this.fairPlayPfxPassword;
}
/**
* @return The discriminator for derived types.
* Expected value is '#Microsoft.Media.ContentKeyPolicyFairPlayConfiguration'.
*
*/
public String odataType() {
return this.odataType;
}
/**
* @return Offline rental policy
*
*/
public Optional offlineRentalConfiguration() {
return Optional.ofNullable(this.offlineRentalConfiguration);
}
/**
* @return The rental and lease key type.
*
*/
public String rentalAndLeaseKeyType() {
return this.rentalAndLeaseKeyType;
}
/**
* @return The rental duration. Must be greater than or equal to 0.
*
*/
public Double rentalDuration() {
return this.rentalDuration;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ContentKeyPolicyFairPlayConfigurationResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String ask;
private String fairPlayPfx;
private String fairPlayPfxPassword;
private String odataType;
private @Nullable ContentKeyPolicyFairPlayOfflineRentalConfigurationResponse offlineRentalConfiguration;
private String rentalAndLeaseKeyType;
private Double rentalDuration;
public Builder() {}
public Builder(ContentKeyPolicyFairPlayConfigurationResponse defaults) {
Objects.requireNonNull(defaults);
this.ask = defaults.ask;
this.fairPlayPfx = defaults.fairPlayPfx;
this.fairPlayPfxPassword = defaults.fairPlayPfxPassword;
this.odataType = defaults.odataType;
this.offlineRentalConfiguration = defaults.offlineRentalConfiguration;
this.rentalAndLeaseKeyType = defaults.rentalAndLeaseKeyType;
this.rentalDuration = defaults.rentalDuration;
}
@CustomType.Setter
public Builder ask(String ask) {
if (ask == null) {
throw new MissingRequiredPropertyException("ContentKeyPolicyFairPlayConfigurationResponse", "ask");
}
this.ask = ask;
return this;
}
@CustomType.Setter
public Builder fairPlayPfx(String fairPlayPfx) {
if (fairPlayPfx == null) {
throw new MissingRequiredPropertyException("ContentKeyPolicyFairPlayConfigurationResponse", "fairPlayPfx");
}
this.fairPlayPfx = fairPlayPfx;
return this;
}
@CustomType.Setter
public Builder fairPlayPfxPassword(String fairPlayPfxPassword) {
if (fairPlayPfxPassword == null) {
throw new MissingRequiredPropertyException("ContentKeyPolicyFairPlayConfigurationResponse", "fairPlayPfxPassword");
}
this.fairPlayPfxPassword = fairPlayPfxPassword;
return this;
}
@CustomType.Setter
public Builder odataType(String odataType) {
if (odataType == null) {
throw new MissingRequiredPropertyException("ContentKeyPolicyFairPlayConfigurationResponse", "odataType");
}
this.odataType = odataType;
return this;
}
@CustomType.Setter
public Builder offlineRentalConfiguration(@Nullable ContentKeyPolicyFairPlayOfflineRentalConfigurationResponse offlineRentalConfiguration) {
this.offlineRentalConfiguration = offlineRentalConfiguration;
return this;
}
@CustomType.Setter
public Builder rentalAndLeaseKeyType(String rentalAndLeaseKeyType) {
if (rentalAndLeaseKeyType == null) {
throw new MissingRequiredPropertyException("ContentKeyPolicyFairPlayConfigurationResponse", "rentalAndLeaseKeyType");
}
this.rentalAndLeaseKeyType = rentalAndLeaseKeyType;
return this;
}
@CustomType.Setter
public Builder rentalDuration(Double rentalDuration) {
if (rentalDuration == null) {
throw new MissingRequiredPropertyException("ContentKeyPolicyFairPlayConfigurationResponse", "rentalDuration");
}
this.rentalDuration = rentalDuration;
return this;
}
public ContentKeyPolicyFairPlayConfigurationResponse build() {
final var _resultValue = new ContentKeyPolicyFairPlayConfigurationResponse();
_resultValue.ask = ask;
_resultValue.fairPlayPfx = fairPlayPfx;
_resultValue.fairPlayPfxPassword = fairPlayPfxPassword;
_resultValue.odataType = odataType;
_resultValue.offlineRentalConfiguration = offlineRentalConfiguration;
_resultValue.rentalAndLeaseKeyType = rentalAndLeaseKeyType;
_resultValue.rentalDuration = rentalDuration;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy