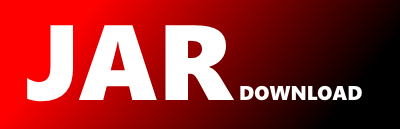
com.pulumi.azurenative.media.outputs.GetLiveEventResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media.outputs;
import com.pulumi.azurenative.media.outputs.CrossSiteAccessPoliciesResponse;
import com.pulumi.azurenative.media.outputs.LiveEventEncodingResponse;
import com.pulumi.azurenative.media.outputs.LiveEventInputResponse;
import com.pulumi.azurenative.media.outputs.LiveEventPreviewResponse;
import com.pulumi.azurenative.media.outputs.LiveEventTranscriptionResponse;
import com.pulumi.azurenative.media.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetLiveEventResult {
/**
* @return The creation time for the live event
*
*/
private String created;
/**
* @return Live event cross site access policies.
*
*/
private @Nullable CrossSiteAccessPoliciesResponse crossSiteAccessPolicies;
/**
* @return A description for the live event.
*
*/
private @Nullable String description;
/**
* @return Encoding settings for the live event. It configures whether a live encoder is used for the live event and settings for the live encoder if it is used.
*
*/
private @Nullable LiveEventEncodingResponse encoding;
/**
* @return When useStaticHostname is set to true, the hostnamePrefix specifies the first part of the hostname assigned to the live event preview and ingest endpoints. The final hostname would be a combination of this prefix, the media service account name and a short code for the Azure Media Services data center.
*
*/
private @Nullable String hostnamePrefix;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return Live event input settings. It defines how the live event receives input from a contribution encoder.
*
*/
private LiveEventInputResponse input;
/**
* @return The last modified time of the live event.
*
*/
private String lastModified;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Live event preview settings. Preview allows live event producers to preview the live streaming content without creating any live output.
*
*/
private @Nullable LiveEventPreviewResponse preview;
/**
* @return The provisioning state of the live event.
*
*/
private String provisioningState;
/**
* @return The resource state of the live event. See https://go.microsoft.com/fwlink/?linkid=2139012 for more information.
*
*/
private String resourceState;
/**
* @return The options to use for the LiveEvent. This value is specified at creation time and cannot be updated. The valid values for the array entry values are 'Default' and 'LowLatency'.
*
*/
private @Nullable List streamOptions;
/**
* @return The system metadata relating to this resource.
*
*/
private SystemDataResponse systemData;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Live transcription settings for the live event. See https://go.microsoft.com/fwlink/?linkid=2133742 for more information about the live transcription feature.
*
*/
private @Nullable List transcriptions;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
/**
* @return Specifies whether a static hostname would be assigned to the live event preview and ingest endpoints. This value can only be updated if the live event is in Standby state
*
*/
private @Nullable Boolean useStaticHostname;
private GetLiveEventResult() {}
/**
* @return The creation time for the live event
*
*/
public String created() {
return this.created;
}
/**
* @return Live event cross site access policies.
*
*/
public Optional crossSiteAccessPolicies() {
return Optional.ofNullable(this.crossSiteAccessPolicies);
}
/**
* @return A description for the live event.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return Encoding settings for the live event. It configures whether a live encoder is used for the live event and settings for the live encoder if it is used.
*
*/
public Optional encoding() {
return Optional.ofNullable(this.encoding);
}
/**
* @return When useStaticHostname is set to true, the hostnamePrefix specifies the first part of the hostname assigned to the live event preview and ingest endpoints. The final hostname would be a combination of this prefix, the media service account name and a short code for the Azure Media Services data center.
*
*/
public Optional hostnamePrefix() {
return Optional.ofNullable(this.hostnamePrefix);
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return Live event input settings. It defines how the live event receives input from a contribution encoder.
*
*/
public LiveEventInputResponse input() {
return this.input;
}
/**
* @return The last modified time of the live event.
*
*/
public String lastModified() {
return this.lastModified;
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Live event preview settings. Preview allows live event producers to preview the live streaming content without creating any live output.
*
*/
public Optional preview() {
return Optional.ofNullable(this.preview);
}
/**
* @return The provisioning state of the live event.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The resource state of the live event. See https://go.microsoft.com/fwlink/?linkid=2139012 for more information.
*
*/
public String resourceState() {
return this.resourceState;
}
/**
* @return The options to use for the LiveEvent. This value is specified at creation time and cannot be updated. The valid values for the array entry values are 'Default' and 'LowLatency'.
*
*/
public List streamOptions() {
return this.streamOptions == null ? List.of() : this.streamOptions;
}
/**
* @return The system metadata relating to this resource.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Live transcription settings for the live event. See https://go.microsoft.com/fwlink/?linkid=2133742 for more information about the live transcription feature.
*
*/
public List transcriptions() {
return this.transcriptions == null ? List.of() : this.transcriptions;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
/**
* @return Specifies whether a static hostname would be assigned to the live event preview and ingest endpoints. This value can only be updated if the live event is in Standby state
*
*/
public Optional useStaticHostname() {
return Optional.ofNullable(this.useStaticHostname);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetLiveEventResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String created;
private @Nullable CrossSiteAccessPoliciesResponse crossSiteAccessPolicies;
private @Nullable String description;
private @Nullable LiveEventEncodingResponse encoding;
private @Nullable String hostnamePrefix;
private String id;
private LiveEventInputResponse input;
private String lastModified;
private String location;
private String name;
private @Nullable LiveEventPreviewResponse preview;
private String provisioningState;
private String resourceState;
private @Nullable List streamOptions;
private SystemDataResponse systemData;
private @Nullable Map tags;
private @Nullable List transcriptions;
private String type;
private @Nullable Boolean useStaticHostname;
public Builder() {}
public Builder(GetLiveEventResult defaults) {
Objects.requireNonNull(defaults);
this.created = defaults.created;
this.crossSiteAccessPolicies = defaults.crossSiteAccessPolicies;
this.description = defaults.description;
this.encoding = defaults.encoding;
this.hostnamePrefix = defaults.hostnamePrefix;
this.id = defaults.id;
this.input = defaults.input;
this.lastModified = defaults.lastModified;
this.location = defaults.location;
this.name = defaults.name;
this.preview = defaults.preview;
this.provisioningState = defaults.provisioningState;
this.resourceState = defaults.resourceState;
this.streamOptions = defaults.streamOptions;
this.systemData = defaults.systemData;
this.tags = defaults.tags;
this.transcriptions = defaults.transcriptions;
this.type = defaults.type;
this.useStaticHostname = defaults.useStaticHostname;
}
@CustomType.Setter
public Builder created(String created) {
if (created == null) {
throw new MissingRequiredPropertyException("GetLiveEventResult", "created");
}
this.created = created;
return this;
}
@CustomType.Setter
public Builder crossSiteAccessPolicies(@Nullable CrossSiteAccessPoliciesResponse crossSiteAccessPolicies) {
this.crossSiteAccessPolicies = crossSiteAccessPolicies;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder encoding(@Nullable LiveEventEncodingResponse encoding) {
this.encoding = encoding;
return this;
}
@CustomType.Setter
public Builder hostnamePrefix(@Nullable String hostnamePrefix) {
this.hostnamePrefix = hostnamePrefix;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetLiveEventResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder input(LiveEventInputResponse input) {
if (input == null) {
throw new MissingRequiredPropertyException("GetLiveEventResult", "input");
}
this.input = input;
return this;
}
@CustomType.Setter
public Builder lastModified(String lastModified) {
if (lastModified == null) {
throw new MissingRequiredPropertyException("GetLiveEventResult", "lastModified");
}
this.lastModified = lastModified;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetLiveEventResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetLiveEventResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder preview(@Nullable LiveEventPreviewResponse preview) {
this.preview = preview;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetLiveEventResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder resourceState(String resourceState) {
if (resourceState == null) {
throw new MissingRequiredPropertyException("GetLiveEventResult", "resourceState");
}
this.resourceState = resourceState;
return this;
}
@CustomType.Setter
public Builder streamOptions(@Nullable List streamOptions) {
this.streamOptions = streamOptions;
return this;
}
public Builder streamOptions(String... streamOptions) {
return streamOptions(List.of(streamOptions));
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetLiveEventResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder transcriptions(@Nullable List transcriptions) {
this.transcriptions = transcriptions;
return this;
}
public Builder transcriptions(LiveEventTranscriptionResponse... transcriptions) {
return transcriptions(List.of(transcriptions));
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetLiveEventResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder useStaticHostname(@Nullable Boolean useStaticHostname) {
this.useStaticHostname = useStaticHostname;
return this;
}
public GetLiveEventResult build() {
final var _resultValue = new GetLiveEventResult();
_resultValue.created = created;
_resultValue.crossSiteAccessPolicies = crossSiteAccessPolicies;
_resultValue.description = description;
_resultValue.encoding = encoding;
_resultValue.hostnamePrefix = hostnamePrefix;
_resultValue.id = id;
_resultValue.input = input;
_resultValue.lastModified = lastModified;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.preview = preview;
_resultValue.provisioningState = provisioningState;
_resultValue.resourceState = resourceState;
_resultValue.streamOptions = streamOptions;
_resultValue.systemData = systemData;
_resultValue.tags = tags;
_resultValue.transcriptions = transcriptions;
_resultValue.type = type;
_resultValue.useStaticHostname = useStaticHostname;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy