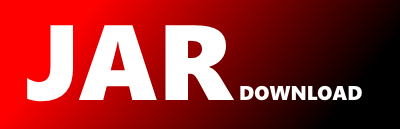
com.pulumi.azurenative.migrate.inputs.AzureSettingsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.migrate.inputs;
import com.pulumi.azurenative.migrate.enums.BusinessCaseCurrency;
import com.pulumi.azurenative.migrate.enums.DiscoverySource;
import com.pulumi.azurenative.migrate.enums.MigrationStrategy;
import com.pulumi.azurenative.migrate.enums.SavingsOption;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Azure settings for a business case.
*
*/
public final class AzureSettingsArgs extends com.pulumi.resources.ResourceArgs {
public static final AzureSettingsArgs Empty = new AzureSettingsArgs();
/**
* Gets Avs labour cost percentage.
*
*/
@Import(name="avsLaborCostPercentage")
private @Nullable Output avsLaborCostPercentage;
/**
* @return Gets Avs labour cost percentage.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy