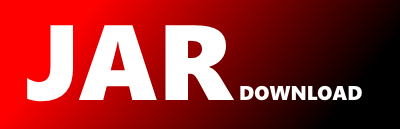
com.pulumi.azurenative.migrate.inputs.ProjectPropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.migrate.inputs;
import com.pulumi.azurenative.migrate.enums.ProjectStatus;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Properties of a project.
*
*/
public final class ProjectPropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final ProjectPropertiesArgs Empty = new ProjectPropertiesArgs();
/**
* Assessment solution ARM id tracked by Microsoft.Migrate/migrateProjects.
*
*/
@Import(name="assessmentSolutionId")
private @Nullable Output assessmentSolutionId;
/**
* @return Assessment solution ARM id tracked by Microsoft.Migrate/migrateProjects.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy