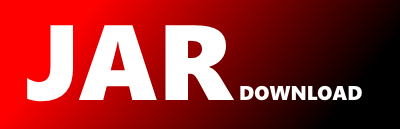
com.pulumi.azurenative.migrate.outputs.GmsaAuthenticationPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.migrate.outputs;
import com.pulumi.azurenative.migrate.outputs.KeyVaultSecretStorePropertiesResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GmsaAuthenticationPropertiesResponse {
/**
* @return Gets or sets the list of dns server that can resolve the Active Directory Domain Name/Address.
*
*/
private @Nullable String adDomainControllerDns;
/**
* @return Gets or sets the FQDN of the Active Directory Domain. For e.g. 'contoso.local', 'fareast.corp.microsoft.com' etc.
*
*/
private @Nullable String adDomainFqdn;
private @Nullable KeyVaultSecretStorePropertiesResponse akvProperties;
/**
* @return Gets or sets the current state of GMSA configuration.
*
*/
private String configurationState;
/**
* @return Gets or sets the password of the user specified by RestApi.Controllers.V2022_05_01_preview.Models.WorkloadDeployment.Gmsa.GmsaAuthenticationProperties.DomainAdminUsername.
*
*/
private @Nullable String domainAdminPassword;
/**
* @return Gets or sets the name of the user having admin rights on the Active Directory Domain Controller.
*
*/
private @Nullable String domainAdminUsername;
/**
* @return Gets or sets the address of the Active Directory Domain Controller running Domain Services.
*
*/
private @Nullable String domainControllerAddress;
/**
* @return Gets or sets the name to be used for GMSA.
*
*/
private @Nullable String gmsaAccountName;
/**
* @return Gets Cred Spec Name to be used.
*
*/
private String gmsaCredSpecName;
/**
* @return Gets name of the secret where GMSA secret is stored in the KeyVault.
*
*/
private String gmsaSecretName;
/**
* @return Gets or sets the password of the user specified by RestApi.Controllers.V2022_05_01_preview.Models.WorkloadDeployment.Gmsa.GmsaAuthenticationProperties.GmsaUsername.
*
*/
private @Nullable String gmsaUserPassword;
/**
* @return Gets or sets username of the user having authorization to access GMSA on Active Directory.
*
*/
private @Nullable String gmsaUsername;
private GmsaAuthenticationPropertiesResponse() {}
/**
* @return Gets or sets the list of dns server that can resolve the Active Directory Domain Name/Address.
*
*/
public Optional adDomainControllerDns() {
return Optional.ofNullable(this.adDomainControllerDns);
}
/**
* @return Gets or sets the FQDN of the Active Directory Domain. For e.g. 'contoso.local', 'fareast.corp.microsoft.com' etc.
*
*/
public Optional adDomainFqdn() {
return Optional.ofNullable(this.adDomainFqdn);
}
public Optional akvProperties() {
return Optional.ofNullable(this.akvProperties);
}
/**
* @return Gets or sets the current state of GMSA configuration.
*
*/
public String configurationState() {
return this.configurationState;
}
/**
* @return Gets or sets the password of the user specified by RestApi.Controllers.V2022_05_01_preview.Models.WorkloadDeployment.Gmsa.GmsaAuthenticationProperties.DomainAdminUsername.
*
*/
public Optional domainAdminPassword() {
return Optional.ofNullable(this.domainAdminPassword);
}
/**
* @return Gets or sets the name of the user having admin rights on the Active Directory Domain Controller.
*
*/
public Optional domainAdminUsername() {
return Optional.ofNullable(this.domainAdminUsername);
}
/**
* @return Gets or sets the address of the Active Directory Domain Controller running Domain Services.
*
*/
public Optional domainControllerAddress() {
return Optional.ofNullable(this.domainControllerAddress);
}
/**
* @return Gets or sets the name to be used for GMSA.
*
*/
public Optional gmsaAccountName() {
return Optional.ofNullable(this.gmsaAccountName);
}
/**
* @return Gets Cred Spec Name to be used.
*
*/
public String gmsaCredSpecName() {
return this.gmsaCredSpecName;
}
/**
* @return Gets name of the secret where GMSA secret is stored in the KeyVault.
*
*/
public String gmsaSecretName() {
return this.gmsaSecretName;
}
/**
* @return Gets or sets the password of the user specified by RestApi.Controllers.V2022_05_01_preview.Models.WorkloadDeployment.Gmsa.GmsaAuthenticationProperties.GmsaUsername.
*
*/
public Optional gmsaUserPassword() {
return Optional.ofNullable(this.gmsaUserPassword);
}
/**
* @return Gets or sets username of the user having authorization to access GMSA on Active Directory.
*
*/
public Optional gmsaUsername() {
return Optional.ofNullable(this.gmsaUsername);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GmsaAuthenticationPropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String adDomainControllerDns;
private @Nullable String adDomainFqdn;
private @Nullable KeyVaultSecretStorePropertiesResponse akvProperties;
private String configurationState;
private @Nullable String domainAdminPassword;
private @Nullable String domainAdminUsername;
private @Nullable String domainControllerAddress;
private @Nullable String gmsaAccountName;
private String gmsaCredSpecName;
private String gmsaSecretName;
private @Nullable String gmsaUserPassword;
private @Nullable String gmsaUsername;
public Builder() {}
public Builder(GmsaAuthenticationPropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.adDomainControllerDns = defaults.adDomainControllerDns;
this.adDomainFqdn = defaults.adDomainFqdn;
this.akvProperties = defaults.akvProperties;
this.configurationState = defaults.configurationState;
this.domainAdminPassword = defaults.domainAdminPassword;
this.domainAdminUsername = defaults.domainAdminUsername;
this.domainControllerAddress = defaults.domainControllerAddress;
this.gmsaAccountName = defaults.gmsaAccountName;
this.gmsaCredSpecName = defaults.gmsaCredSpecName;
this.gmsaSecretName = defaults.gmsaSecretName;
this.gmsaUserPassword = defaults.gmsaUserPassword;
this.gmsaUsername = defaults.gmsaUsername;
}
@CustomType.Setter
public Builder adDomainControllerDns(@Nullable String adDomainControllerDns) {
this.adDomainControllerDns = adDomainControllerDns;
return this;
}
@CustomType.Setter
public Builder adDomainFqdn(@Nullable String adDomainFqdn) {
this.adDomainFqdn = adDomainFqdn;
return this;
}
@CustomType.Setter
public Builder akvProperties(@Nullable KeyVaultSecretStorePropertiesResponse akvProperties) {
this.akvProperties = akvProperties;
return this;
}
@CustomType.Setter
public Builder configurationState(String configurationState) {
if (configurationState == null) {
throw new MissingRequiredPropertyException("GmsaAuthenticationPropertiesResponse", "configurationState");
}
this.configurationState = configurationState;
return this;
}
@CustomType.Setter
public Builder domainAdminPassword(@Nullable String domainAdminPassword) {
this.domainAdminPassword = domainAdminPassword;
return this;
}
@CustomType.Setter
public Builder domainAdminUsername(@Nullable String domainAdminUsername) {
this.domainAdminUsername = domainAdminUsername;
return this;
}
@CustomType.Setter
public Builder domainControllerAddress(@Nullable String domainControllerAddress) {
this.domainControllerAddress = domainControllerAddress;
return this;
}
@CustomType.Setter
public Builder gmsaAccountName(@Nullable String gmsaAccountName) {
this.gmsaAccountName = gmsaAccountName;
return this;
}
@CustomType.Setter
public Builder gmsaCredSpecName(String gmsaCredSpecName) {
if (gmsaCredSpecName == null) {
throw new MissingRequiredPropertyException("GmsaAuthenticationPropertiesResponse", "gmsaCredSpecName");
}
this.gmsaCredSpecName = gmsaCredSpecName;
return this;
}
@CustomType.Setter
public Builder gmsaSecretName(String gmsaSecretName) {
if (gmsaSecretName == null) {
throw new MissingRequiredPropertyException("GmsaAuthenticationPropertiesResponse", "gmsaSecretName");
}
this.gmsaSecretName = gmsaSecretName;
return this;
}
@CustomType.Setter
public Builder gmsaUserPassword(@Nullable String gmsaUserPassword) {
this.gmsaUserPassword = gmsaUserPassword;
return this;
}
@CustomType.Setter
public Builder gmsaUsername(@Nullable String gmsaUsername) {
this.gmsaUsername = gmsaUsername;
return this;
}
public GmsaAuthenticationPropertiesResponse build() {
final var _resultValue = new GmsaAuthenticationPropertiesResponse();
_resultValue.adDomainControllerDns = adDomainControllerDns;
_resultValue.adDomainFqdn = adDomainFqdn;
_resultValue.akvProperties = akvProperties;
_resultValue.configurationState = configurationState;
_resultValue.domainAdminPassword = domainAdminPassword;
_resultValue.domainAdminUsername = domainAdminUsername;
_resultValue.domainControllerAddress = domainControllerAddress;
_resultValue.gmsaAccountName = gmsaAccountName;
_resultValue.gmsaCredSpecName = gmsaCredSpecName;
_resultValue.gmsaSecretName = gmsaSecretName;
_resultValue.gmsaUserPassword = gmsaUserPassword;
_resultValue.gmsaUsername = gmsaUsername;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy