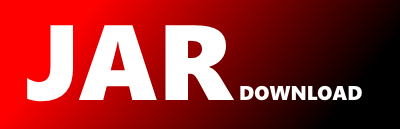
com.pulumi.azurenative.migrate.outputs.IISAKSWorkloadDeploymentResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.migrate.outputs;
import com.pulumi.azurenative.migrate.outputs.AKSDeploymentPropertiesResponse;
import com.pulumi.azurenative.migrate.outputs.AKSDeploymentSpecificationResponse;
import com.pulumi.azurenative.migrate.outputs.AppInsightMonitoringPropertiesResponse;
import com.pulumi.azurenative.migrate.outputs.AutomationArtifactResponse;
import com.pulumi.azurenative.migrate.outputs.BindingResponse;
import com.pulumi.azurenative.migrate.outputs.ContainerImagePropertiesResponse;
import com.pulumi.azurenative.migrate.outputs.DeployedResourcesPropertiesResponse;
import com.pulumi.azurenative.migrate.outputs.GmsaAuthenticationPropertiesResponse;
import com.pulumi.azurenative.migrate.outputs.ResourceRequirementsResponse;
import com.pulumi.azurenative.migrate.outputs.WebApplicationConfigurationResponse;
import com.pulumi.azurenative.migrate.outputs.WebApplicationDirectoryResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class IISAKSWorkloadDeploymentResponse {
/**
* @return Class for GMSA authentication details to configure Active Directory connectivity.
*
*/
private @Nullable GmsaAuthenticationPropertiesResponse authenticationProperties;
/**
* @return Class for automation artifact.
*
*/
private @Nullable AutomationArtifactResponse automationArtifactProperties;
/**
* @return Gets or sets the bindings for the application.
*
*/
private @Nullable List bindings;
/**
* @return Gets or sets the build container images.
*
*/
private @Nullable List buildContainerImages;
/**
* @return Class for AKSDeployment Properties.
*
*/
private @Nullable AKSDeploymentPropertiesResponse clusterProperties;
/**
* @return Gets or sets application configuration.
*
*/
private @Nullable List configurations;
/**
* @return Class for container image properties.
*
*/
private @Nullable ContainerImagePropertiesResponse containerImageProperties;
/**
* @return Gets or sets the deployment history.
*
*/
private List deploymentHistory;
/**
* @return Gets or sets the deployment name prefix.
*
*/
private @Nullable String deploymentNamePrefix;
/**
* @return AKS Deployment Specification.
*
*/
private @Nullable AKSDeploymentSpecificationResponse deploymentSpec;
/**
* @return Gets or sets application directories.
*
*/
private @Nullable List directories;
/**
* @return Resource Requirements.
*
*/
private @Nullable ResourceRequirementsResponse limits;
/**
* @return Class for app insight monitoring properties.
*
*/
private @Nullable AppInsightMonitoringPropertiesResponse monitoringProperties;
/**
* @return Resource Requirements.
*
*/
private @Nullable ResourceRequirementsResponse requests;
/**
* @return Gets or sets the target platform managed identity.
*
*/
private @Nullable String targetPlatformIdentity;
private IISAKSWorkloadDeploymentResponse() {}
/**
* @return Class for GMSA authentication details to configure Active Directory connectivity.
*
*/
public Optional authenticationProperties() {
return Optional.ofNullable(this.authenticationProperties);
}
/**
* @return Class for automation artifact.
*
*/
public Optional automationArtifactProperties() {
return Optional.ofNullable(this.automationArtifactProperties);
}
/**
* @return Gets or sets the bindings for the application.
*
*/
public List bindings() {
return this.bindings == null ? List.of() : this.bindings;
}
/**
* @return Gets or sets the build container images.
*
*/
public List buildContainerImages() {
return this.buildContainerImages == null ? List.of() : this.buildContainerImages;
}
/**
* @return Class for AKSDeployment Properties.
*
*/
public Optional clusterProperties() {
return Optional.ofNullable(this.clusterProperties);
}
/**
* @return Gets or sets application configuration.
*
*/
public List configurations() {
return this.configurations == null ? List.of() : this.configurations;
}
/**
* @return Class for container image properties.
*
*/
public Optional containerImageProperties() {
return Optional.ofNullable(this.containerImageProperties);
}
/**
* @return Gets or sets the deployment history.
*
*/
public List deploymentHistory() {
return this.deploymentHistory;
}
/**
* @return Gets or sets the deployment name prefix.
*
*/
public Optional deploymentNamePrefix() {
return Optional.ofNullable(this.deploymentNamePrefix);
}
/**
* @return AKS Deployment Specification.
*
*/
public Optional deploymentSpec() {
return Optional.ofNullable(this.deploymentSpec);
}
/**
* @return Gets or sets application directories.
*
*/
public List directories() {
return this.directories == null ? List.of() : this.directories;
}
/**
* @return Resource Requirements.
*
*/
public Optional limits() {
return Optional.ofNullable(this.limits);
}
/**
* @return Class for app insight monitoring properties.
*
*/
public Optional monitoringProperties() {
return Optional.ofNullable(this.monitoringProperties);
}
/**
* @return Resource Requirements.
*
*/
public Optional requests() {
return Optional.ofNullable(this.requests);
}
/**
* @return Gets or sets the target platform managed identity.
*
*/
public Optional targetPlatformIdentity() {
return Optional.ofNullable(this.targetPlatformIdentity);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(IISAKSWorkloadDeploymentResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable GmsaAuthenticationPropertiesResponse authenticationProperties;
private @Nullable AutomationArtifactResponse automationArtifactProperties;
private @Nullable List bindings;
private @Nullable List buildContainerImages;
private @Nullable AKSDeploymentPropertiesResponse clusterProperties;
private @Nullable List configurations;
private @Nullable ContainerImagePropertiesResponse containerImageProperties;
private List deploymentHistory;
private @Nullable String deploymentNamePrefix;
private @Nullable AKSDeploymentSpecificationResponse deploymentSpec;
private @Nullable List directories;
private @Nullable ResourceRequirementsResponse limits;
private @Nullable AppInsightMonitoringPropertiesResponse monitoringProperties;
private @Nullable ResourceRequirementsResponse requests;
private @Nullable String targetPlatformIdentity;
public Builder() {}
public Builder(IISAKSWorkloadDeploymentResponse defaults) {
Objects.requireNonNull(defaults);
this.authenticationProperties = defaults.authenticationProperties;
this.automationArtifactProperties = defaults.automationArtifactProperties;
this.bindings = defaults.bindings;
this.buildContainerImages = defaults.buildContainerImages;
this.clusterProperties = defaults.clusterProperties;
this.configurations = defaults.configurations;
this.containerImageProperties = defaults.containerImageProperties;
this.deploymentHistory = defaults.deploymentHistory;
this.deploymentNamePrefix = defaults.deploymentNamePrefix;
this.deploymentSpec = defaults.deploymentSpec;
this.directories = defaults.directories;
this.limits = defaults.limits;
this.monitoringProperties = defaults.monitoringProperties;
this.requests = defaults.requests;
this.targetPlatformIdentity = defaults.targetPlatformIdentity;
}
@CustomType.Setter
public Builder authenticationProperties(@Nullable GmsaAuthenticationPropertiesResponse authenticationProperties) {
this.authenticationProperties = authenticationProperties;
return this;
}
@CustomType.Setter
public Builder automationArtifactProperties(@Nullable AutomationArtifactResponse automationArtifactProperties) {
this.automationArtifactProperties = automationArtifactProperties;
return this;
}
@CustomType.Setter
public Builder bindings(@Nullable List bindings) {
this.bindings = bindings;
return this;
}
public Builder bindings(BindingResponse... bindings) {
return bindings(List.of(bindings));
}
@CustomType.Setter
public Builder buildContainerImages(@Nullable List buildContainerImages) {
this.buildContainerImages = buildContainerImages;
return this;
}
public Builder buildContainerImages(ContainerImagePropertiesResponse... buildContainerImages) {
return buildContainerImages(List.of(buildContainerImages));
}
@CustomType.Setter
public Builder clusterProperties(@Nullable AKSDeploymentPropertiesResponse clusterProperties) {
this.clusterProperties = clusterProperties;
return this;
}
@CustomType.Setter
public Builder configurations(@Nullable List configurations) {
this.configurations = configurations;
return this;
}
public Builder configurations(WebApplicationConfigurationResponse... configurations) {
return configurations(List.of(configurations));
}
@CustomType.Setter
public Builder containerImageProperties(@Nullable ContainerImagePropertiesResponse containerImageProperties) {
this.containerImageProperties = containerImageProperties;
return this;
}
@CustomType.Setter
public Builder deploymentHistory(List deploymentHistory) {
if (deploymentHistory == null) {
throw new MissingRequiredPropertyException("IISAKSWorkloadDeploymentResponse", "deploymentHistory");
}
this.deploymentHistory = deploymentHistory;
return this;
}
public Builder deploymentHistory(DeployedResourcesPropertiesResponse... deploymentHistory) {
return deploymentHistory(List.of(deploymentHistory));
}
@CustomType.Setter
public Builder deploymentNamePrefix(@Nullable String deploymentNamePrefix) {
this.deploymentNamePrefix = deploymentNamePrefix;
return this;
}
@CustomType.Setter
public Builder deploymentSpec(@Nullable AKSDeploymentSpecificationResponse deploymentSpec) {
this.deploymentSpec = deploymentSpec;
return this;
}
@CustomType.Setter
public Builder directories(@Nullable List directories) {
this.directories = directories;
return this;
}
public Builder directories(WebApplicationDirectoryResponse... directories) {
return directories(List.of(directories));
}
@CustomType.Setter
public Builder limits(@Nullable ResourceRequirementsResponse limits) {
this.limits = limits;
return this;
}
@CustomType.Setter
public Builder monitoringProperties(@Nullable AppInsightMonitoringPropertiesResponse monitoringProperties) {
this.monitoringProperties = monitoringProperties;
return this;
}
@CustomType.Setter
public Builder requests(@Nullable ResourceRequirementsResponse requests) {
this.requests = requests;
return this;
}
@CustomType.Setter
public Builder targetPlatformIdentity(@Nullable String targetPlatformIdentity) {
this.targetPlatformIdentity = targetPlatformIdentity;
return this;
}
public IISAKSWorkloadDeploymentResponse build() {
final var _resultValue = new IISAKSWorkloadDeploymentResponse();
_resultValue.authenticationProperties = authenticationProperties;
_resultValue.automationArtifactProperties = automationArtifactProperties;
_resultValue.bindings = bindings;
_resultValue.buildContainerImages = buildContainerImages;
_resultValue.clusterProperties = clusterProperties;
_resultValue.configurations = configurations;
_resultValue.containerImageProperties = containerImageProperties;
_resultValue.deploymentHistory = deploymentHistory;
_resultValue.deploymentNamePrefix = deploymentNamePrefix;
_resultValue.deploymentSpec = deploymentSpec;
_resultValue.directories = directories;
_resultValue.limits = limits;
_resultValue.monitoringProperties = monitoringProperties;
_resultValue.requests = requests;
_resultValue.targetPlatformIdentity = targetPlatformIdentity;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy