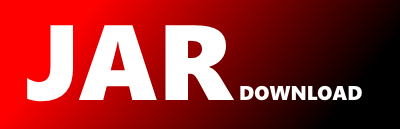
com.pulumi.azurenative.mobilenetwork.PacketCoreControlPlane Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.mobilenetwork;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.mobilenetwork.PacketCoreControlPlaneArgs;
import com.pulumi.azurenative.mobilenetwork.outputs.DiagnosticsUploadConfigurationResponse;
import com.pulumi.azurenative.mobilenetwork.outputs.InstallationResponse;
import com.pulumi.azurenative.mobilenetwork.outputs.InterfacePropertiesResponse;
import com.pulumi.azurenative.mobilenetwork.outputs.LocalDiagnosticsAccessConfigurationResponse;
import com.pulumi.azurenative.mobilenetwork.outputs.ManagedServiceIdentityResponse;
import com.pulumi.azurenative.mobilenetwork.outputs.PlatformConfigurationResponse;
import com.pulumi.azurenative.mobilenetwork.outputs.SiteResourceIdResponse;
import com.pulumi.azurenative.mobilenetwork.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Integer;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Packet core control plane resource.
* Azure REST API version: 2023-06-01. Prior API version in Azure Native 1.x: 2022-04-01-preview.
*
* Other available API versions: 2022-03-01-preview, 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
* ## Example Usage
* ### Create packet core control plane
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.mobilenetwork.PacketCoreControlPlane;
* import com.pulumi.azurenative.mobilenetwork.PacketCoreControlPlaneArgs;
* import com.pulumi.azurenative.mobilenetwork.inputs.InterfacePropertiesArgs;
* import com.pulumi.azurenative.mobilenetwork.inputs.InstallationArgs;
* import com.pulumi.azurenative.mobilenetwork.inputs.LocalDiagnosticsAccessConfigurationArgs;
* import com.pulumi.azurenative.mobilenetwork.inputs.HttpsServerCertificateArgs;
* import com.pulumi.azurenative.mobilenetwork.inputs.PlatformConfigurationArgs;
* import com.pulumi.azurenative.mobilenetwork.inputs.AzureStackEdgeDeviceResourceIdArgs;
* import com.pulumi.azurenative.mobilenetwork.inputs.ConnectedClusterResourceIdArgs;
* import com.pulumi.azurenative.mobilenetwork.inputs.CustomLocationResourceIdArgs;
* import com.pulumi.azurenative.mobilenetwork.inputs.SiteResourceIdArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var packetCoreControlPlane = new PacketCoreControlPlane("packetCoreControlPlane", PacketCoreControlPlaneArgs.builder()
* .controlPlaneAccessInterface(InterfacePropertiesArgs.builder()
* .name("N2")
* .build())
* .coreNetworkTechnology("5GC")
* .installation(InstallationArgs.builder()
* .desiredState("Installed")
* .build())
* .localDiagnosticsAccess(LocalDiagnosticsAccessConfigurationArgs.builder()
* .authenticationType("AAD")
* .httpsServerCertificate(HttpsServerCertificateArgs.builder()
* .certificateUrl("https://contosovault.vault.azure.net/certificates/ingress")
* .build())
* .build())
* .location("eastus")
* .packetCoreControlPlaneName("TestPacketCoreCP")
* .platform(PlatformConfigurationArgs.builder()
* .azureStackEdgeDevice(AzureStackEdgeDeviceResourceIdArgs.builder()
* .id("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.DataBoxEdge/dataBoxEdgeDevices/TestAzureStackEdgeDevice")
* .build())
* .connectedCluster(ConnectedClusterResourceIdArgs.builder()
* .id("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.Kubernetes/connectedClusters/TestConnectedCluster")
* .build())
* .customLocation(CustomLocationResourceIdArgs.builder()
* .id("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.ExtendedLocation/customLocations/TestCustomLocation")
* .build())
* .type("AKS-HCI")
* .build())
* .resourceGroupName("rg1")
* .sites(SiteResourceIdArgs.builder()
* .id("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.MobileNetwork/mobileNetworks/testMobileNetwork/sites/testSite")
* .build())
* .sku("G0")
* .ueMtu(1600)
* .version("0.2.0")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:mobilenetwork:PacketCoreControlPlane TestPacketCoreCP /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.MobileNetwork/packetCoreControlPlanes/{packetCoreControlPlaneName}
* ```
*
*/
@ResourceType(type="azure-native:mobilenetwork:PacketCoreControlPlane")
public class PacketCoreControlPlane extends com.pulumi.resources.CustomResource {
/**
* The control plane interface on the access network. For 5G networks, this is the N2 interface. For 4G networks, this is the S1-MME interface.
*
*/
@Export(name="controlPlaneAccessInterface", refs={InterfacePropertiesResponse.class}, tree="[0]")
private Output controlPlaneAccessInterface;
/**
* @return The control plane interface on the access network. For 5G networks, this is the N2 interface. For 4G networks, this is the S1-MME interface.
*
*/
public Output controlPlaneAccessInterface() {
return this.controlPlaneAccessInterface;
}
/**
* The core network technology generation (5G core or EPC / 4G core).
*
*/
@Export(name="coreNetworkTechnology", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> coreNetworkTechnology;
/**
* @return The core network technology generation (5G core or EPC / 4G core).
*
*/
public Output> coreNetworkTechnology() {
return Codegen.optional(this.coreNetworkTechnology);
}
/**
* Configuration for uploading packet core diagnostics
*
*/
@Export(name="diagnosticsUpload", refs={DiagnosticsUploadConfigurationResponse.class}, tree="[0]")
private Output* @Nullable */ DiagnosticsUploadConfigurationResponse> diagnosticsUpload;
/**
* @return Configuration for uploading packet core diagnostics
*
*/
public Output> diagnosticsUpload() {
return Codegen.optional(this.diagnosticsUpload);
}
/**
* The identity used to retrieve the ingress certificate from Azure key vault.
*
*/
@Export(name="identity", refs={ManagedServiceIdentityResponse.class}, tree="[0]")
private Output* @Nullable */ ManagedServiceIdentityResponse> identity;
/**
* @return The identity used to retrieve the ingress certificate from Azure key vault.
*
*/
public Output> identity() {
return Codegen.optional(this.identity);
}
/**
* The installation state of the packet core control plane resource.
*
*/
@Export(name="installation", refs={InstallationResponse.class}, tree="[0]")
private Output* @Nullable */ InstallationResponse> installation;
/**
* @return The installation state of the packet core control plane resource.
*
*/
public Output> installation() {
return Codegen.optional(this.installation);
}
/**
* The currently installed version of the packet core software.
*
*/
@Export(name="installedVersion", refs={String.class}, tree="[0]")
private Output installedVersion;
/**
* @return The currently installed version of the packet core software.
*
*/
public Output installedVersion() {
return this.installedVersion;
}
/**
* Settings to allow interoperability with third party components e.g. RANs and UEs.
*
*/
@Export(name="interopSettings", refs={Object.class}, tree="[0]")
private Output* @Nullable */ Object> interopSettings;
/**
* @return Settings to allow interoperability with third party components e.g. RANs and UEs.
*
*/
public Output> interopSettings() {
return Codegen.optional(this.interopSettings);
}
/**
* The kubernetes ingress configuration to control access to packet core diagnostics over local APIs.
*
*/
@Export(name="localDiagnosticsAccess", refs={LocalDiagnosticsAccessConfigurationResponse.class}, tree="[0]")
private Output localDiagnosticsAccess;
/**
* @return The kubernetes ingress configuration to control access to packet core diagnostics over local APIs.
*
*/
public Output localDiagnosticsAccess() {
return this.localDiagnosticsAccess;
}
/**
* The geo-location where the resource lives
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return The geo-location where the resource lives
*
*/
public Output location() {
return this.location;
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* The platform where the packet core is deployed.
*
*/
@Export(name="platform", refs={PlatformConfigurationResponse.class}, tree="[0]")
private Output platform;
/**
* @return The platform where the packet core is deployed.
*
*/
public Output platform() {
return this.platform;
}
/**
* The provisioning state of the packet core control plane resource.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The provisioning state of the packet core control plane resource.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* The previous version of the packet core software that was deployed. Used when performing the rollback action.
*
*/
@Export(name="rollbackVersion", refs={String.class}, tree="[0]")
private Output rollbackVersion;
/**
* @return The previous version of the packet core software that was deployed. Used when performing the rollback action.
*
*/
public Output rollbackVersion() {
return this.rollbackVersion;
}
/**
* Site(s) under which this packet core control plane should be deployed. The sites must be in the same location as the packet core control plane.
*
*/
@Export(name="sites", refs={List.class,SiteResourceIdResponse.class}, tree="[0,1]")
private Output> sites;
/**
* @return Site(s) under which this packet core control plane should be deployed. The sites must be in the same location as the packet core control plane.
*
*/
public Output> sites() {
return this.sites;
}
/**
* The SKU defining the throughput and SIM allowances for this packet core control plane deployment.
*
*/
@Export(name="sku", refs={String.class}, tree="[0]")
private Output sku;
/**
* @return The SKU defining the throughput and SIM allowances for this packet core control plane deployment.
*
*/
public Output sku() {
return this.sku;
}
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* Resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
* The MTU (in bytes) signaled to the UE. The same MTU is set on the user plane data links for all data networks. The MTU set on the user plane access link is calculated to be 60 bytes greater than this value to allow for GTP encapsulation.
*
*/
@Export(name="ueMtu", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> ueMtu;
/**
* @return The MTU (in bytes) signaled to the UE. The same MTU is set on the user plane data links for all data networks. The MTU set on the user plane access link is calculated to be 60 bytes greater than this value to allow for GTP encapsulation.
*
*/
public Output> ueMtu() {
return Codegen.optional(this.ueMtu);
}
/**
* The desired version of the packet core software.
*
*/
@Export(name="version", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> version;
/**
* @return The desired version of the packet core software.
*
*/
public Output> version() {
return Codegen.optional(this.version);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public PacketCoreControlPlane(java.lang.String name) {
this(name, PacketCoreControlPlaneArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public PacketCoreControlPlane(java.lang.String name, PacketCoreControlPlaneArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public PacketCoreControlPlane(java.lang.String name, PacketCoreControlPlaneArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:mobilenetwork:PacketCoreControlPlane", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private PacketCoreControlPlane(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:mobilenetwork:PacketCoreControlPlane", name, null, makeResourceOptions(options, id), false);
}
private static PacketCoreControlPlaneArgs makeArgs(PacketCoreControlPlaneArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? PacketCoreControlPlaneArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:mobilenetwork/v20220301preview:PacketCoreControlPlane").build()),
Output.of(Alias.builder().type("azure-native:mobilenetwork/v20220401preview:PacketCoreControlPlane").build()),
Output.of(Alias.builder().type("azure-native:mobilenetwork/v20221101:PacketCoreControlPlane").build()),
Output.of(Alias.builder().type("azure-native:mobilenetwork/v20230601:PacketCoreControlPlane").build()),
Output.of(Alias.builder().type("azure-native:mobilenetwork/v20230901:PacketCoreControlPlane").build()),
Output.of(Alias.builder().type("azure-native:mobilenetwork/v20240201:PacketCoreControlPlane").build()),
Output.of(Alias.builder().type("azure-native:mobilenetwork/v20240401:PacketCoreControlPlane").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static PacketCoreControlPlane get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new PacketCoreControlPlane(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy