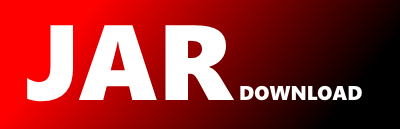
com.pulumi.azurenative.mobilenetwork.SimArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.mobilenetwork;
import com.pulumi.azurenative.mobilenetwork.inputs.SimPolicyResourceIdArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.SimStaticIpPropertiesArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class SimArgs extends com.pulumi.resources.ResourceArgs {
public static final SimArgs Empty = new SimArgs();
/**
* The Ki value for the SIM.
*
*/
@Import(name="authenticationKey")
private @Nullable Output authenticationKey;
/**
* @return The Ki value for the SIM.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy