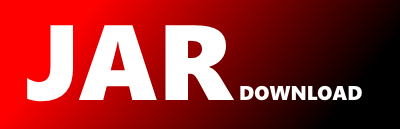
com.pulumi.azurenative.netapp.inputs.ExportPolicyRuleArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.netapp.inputs;
import com.pulumi.azurenative.netapp.enums.ChownMode;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Volume Export Policy Rule
*
*/
public final class ExportPolicyRuleArgs extends com.pulumi.resources.ResourceArgs {
public static final ExportPolicyRuleArgs Empty = new ExportPolicyRuleArgs();
/**
* Client ingress specification as comma separated string with IPv4 CIDRs, IPv4 host addresses and host names
*
*/
@Import(name="allowedClients")
private @Nullable Output allowedClients;
/**
* @return Client ingress specification as comma separated string with IPv4 CIDRs, IPv4 host addresses and host names
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy