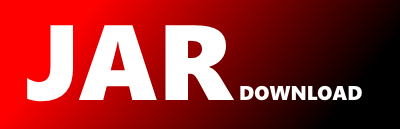
com.pulumi.azurenative.network.AdminRuleArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network;
import com.pulumi.azurenative.network.enums.SecurityConfigurationRuleAccess;
import com.pulumi.azurenative.network.enums.SecurityConfigurationRuleDirection;
import com.pulumi.azurenative.network.enums.SecurityConfigurationRuleProtocol;
import com.pulumi.azurenative.network.inputs.AddressPrefixItemArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class AdminRuleArgs extends com.pulumi.resources.ResourceArgs {
public static final AdminRuleArgs Empty = new AdminRuleArgs();
/**
* Indicates the access allowed for this particular rule
*
*/
@Import(name="access", required=true)
private Output> access;
/**
* @return Indicates the access allowed for this particular rule
*
*/
public Output> access() {
return this.access;
}
/**
* The name of the network manager Security Configuration.
*
*/
@Import(name="configurationName", required=true)
private Output configurationName;
/**
* @return The name of the network manager Security Configuration.
*
*/
public Output configurationName() {
return this.configurationName;
}
/**
* A description for this rule. Restricted to 140 chars.
*
*/
@Import(name="description")
private @Nullable Output description;
/**
* @return A description for this rule. Restricted to 140 chars.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy