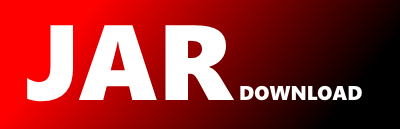
com.pulumi.azurenative.network.DscpConfigurationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network;
import com.pulumi.azurenative.network.enums.ProtocolType;
import com.pulumi.azurenative.network.inputs.QosDefinitionArgs;
import com.pulumi.azurenative.network.inputs.QosIpRangeArgs;
import com.pulumi.azurenative.network.inputs.QosPortRangeArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DscpConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final DscpConfigurationArgs Empty = new DscpConfigurationArgs();
/**
* Destination IP ranges.
*
*/
@Import(name="destinationIpRanges")
private @Nullable Output> destinationIpRanges;
/**
* @return Destination IP ranges.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy