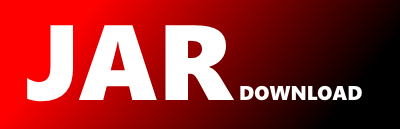
com.pulumi.azurenative.network.FirewallPolicyArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network;
import com.pulumi.azurenative.network.enums.AzureFirewallThreatIntelMode;
import com.pulumi.azurenative.network.inputs.DnsSettingsArgs;
import com.pulumi.azurenative.network.inputs.ExplicitProxyArgs;
import com.pulumi.azurenative.network.inputs.FirewallPolicyInsightsArgs;
import com.pulumi.azurenative.network.inputs.FirewallPolicyIntrusionDetectionArgs;
import com.pulumi.azurenative.network.inputs.FirewallPolicySNATArgs;
import com.pulumi.azurenative.network.inputs.FirewallPolicySQLArgs;
import com.pulumi.azurenative.network.inputs.FirewallPolicySkuArgs;
import com.pulumi.azurenative.network.inputs.FirewallPolicyThreatIntelWhitelistArgs;
import com.pulumi.azurenative.network.inputs.FirewallPolicyTransportSecurityArgs;
import com.pulumi.azurenative.network.inputs.ManagedServiceIdentityArgs;
import com.pulumi.azurenative.network.inputs.SubResourceArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class FirewallPolicyArgs extends com.pulumi.resources.ResourceArgs {
public static final FirewallPolicyArgs Empty = new FirewallPolicyArgs();
/**
* The parent firewall policy from which rules are inherited.
*
*/
@Import(name="basePolicy")
private @Nullable Output basePolicy;
/**
* @return The parent firewall policy from which rules are inherited.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy