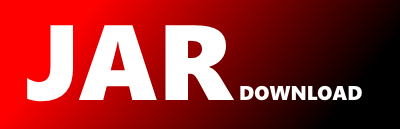
com.pulumi.azurenative.network.LoadBalancerArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network;
import com.pulumi.azurenative.network.inputs.BackendAddressPoolArgs;
import com.pulumi.azurenative.network.inputs.ExtendedLocationArgs;
import com.pulumi.azurenative.network.inputs.FrontendIPConfigurationArgs;
import com.pulumi.azurenative.network.inputs.InboundNatPoolArgs;
import com.pulumi.azurenative.network.inputs.InboundNatRuleArgs;
import com.pulumi.azurenative.network.inputs.LoadBalancerSkuArgs;
import com.pulumi.azurenative.network.inputs.LoadBalancingRuleArgs;
import com.pulumi.azurenative.network.inputs.OutboundRuleArgs;
import com.pulumi.azurenative.network.inputs.ProbeArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class LoadBalancerArgs extends com.pulumi.resources.ResourceArgs {
public static final LoadBalancerArgs Empty = new LoadBalancerArgs();
/**
* Collection of backend address pools used by a load balancer.
* These are also available as standalone resources. Do not mix inline and standalone resource as they will conflict with each other, leading to resources deletion.
*
*/
@Import(name="backendAddressPools")
private @Nullable Output> backendAddressPools;
/**
* @return Collection of backend address pools used by a load balancer.
* These are also available as standalone resources. Do not mix inline and standalone resource as they will conflict with each other, leading to resources deletion.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy