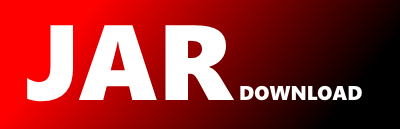
com.pulumi.azurenative.network.NetworkInterfaceArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network;
import com.pulumi.azurenative.network.enums.NetworkInterfaceAuxiliaryMode;
import com.pulumi.azurenative.network.enums.NetworkInterfaceAuxiliarySku;
import com.pulumi.azurenative.network.enums.NetworkInterfaceMigrationPhase;
import com.pulumi.azurenative.network.enums.NetworkInterfaceNicType;
import com.pulumi.azurenative.network.inputs.ExtendedLocationArgs;
import com.pulumi.azurenative.network.inputs.NetworkInterfaceDnsSettingsArgs;
import com.pulumi.azurenative.network.inputs.NetworkInterfaceIPConfigurationArgs;
import com.pulumi.azurenative.network.inputs.NetworkSecurityGroupArgs;
import com.pulumi.azurenative.network.inputs.PrivateLinkServiceArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class NetworkInterfaceArgs extends com.pulumi.resources.ResourceArgs {
public static final NetworkInterfaceArgs Empty = new NetworkInterfaceArgs();
/**
* Auxiliary mode of Network Interface resource.
*
*/
@Import(name="auxiliaryMode")
private @Nullable Output> auxiliaryMode;
/**
* @return Auxiliary mode of Network Interface resource.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy