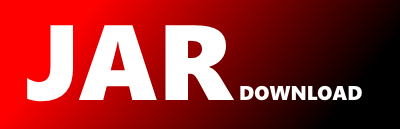
com.pulumi.azurenative.network.SecurityRuleArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network;
import com.pulumi.azurenative.network.enums.SecurityRuleAccess;
import com.pulumi.azurenative.network.enums.SecurityRuleDirection;
import com.pulumi.azurenative.network.enums.SecurityRuleProtocol;
import com.pulumi.azurenative.network.inputs.ApplicationSecurityGroupArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class SecurityRuleArgs extends com.pulumi.resources.ResourceArgs {
public static final SecurityRuleArgs Empty = new SecurityRuleArgs();
/**
* The network traffic is allowed or denied.
*
*/
@Import(name="access", required=true)
private Output> access;
/**
* @return The network traffic is allowed or denied.
*
*/
public Output> access() {
return this.access;
}
/**
* A description for this rule. Restricted to 140 chars.
*
*/
@Import(name="description")
private @Nullable Output description;
/**
* @return A description for this rule. Restricted to 140 chars.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy