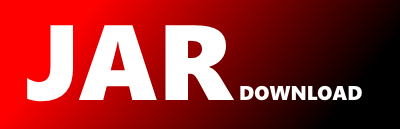
com.pulumi.azurenative.network.SubnetArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network;
import com.pulumi.azurenative.network.enums.VirtualNetworkPrivateEndpointNetworkPolicies;
import com.pulumi.azurenative.network.enums.VirtualNetworkPrivateLinkServiceNetworkPolicies;
import com.pulumi.azurenative.network.inputs.ApplicationGatewayIPConfigurationArgs;
import com.pulumi.azurenative.network.inputs.DelegationArgs;
import com.pulumi.azurenative.network.inputs.NetworkSecurityGroupArgs;
import com.pulumi.azurenative.network.inputs.RouteTableArgs;
import com.pulumi.azurenative.network.inputs.ServiceEndpointPolicyArgs;
import com.pulumi.azurenative.network.inputs.ServiceEndpointPropertiesFormatArgs;
import com.pulumi.azurenative.network.inputs.SubResourceArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class SubnetArgs extends com.pulumi.resources.ResourceArgs {
public static final SubnetArgs Empty = new SubnetArgs();
/**
* The address prefix for the subnet.
*
*/
@Import(name="addressPrefix")
private @Nullable Output addressPrefix;
/**
* @return The address prefix for the subnet.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy