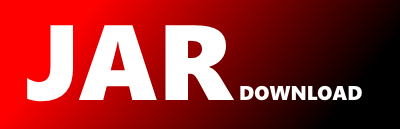
com.pulumi.azurenative.network.inputs.FrontendIPConfigurationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.inputs;
import com.pulumi.azurenative.network.enums.IPAllocationMethod;
import com.pulumi.azurenative.network.enums.IPVersion;
import com.pulumi.azurenative.network.inputs.PublicIPAddressArgs;
import com.pulumi.azurenative.network.inputs.SubResourceArgs;
import com.pulumi.azurenative.network.inputs.SubnetArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Frontend IP address of the load balancer.
*
*/
public final class FrontendIPConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final FrontendIPConfigurationArgs Empty = new FrontendIPConfigurationArgs();
/**
* The reference to gateway load balancer frontend IP.
*
*/
@Import(name="gatewayLoadBalancer")
private @Nullable Output gatewayLoadBalancer;
/**
* @return The reference to gateway load balancer frontend IP.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy