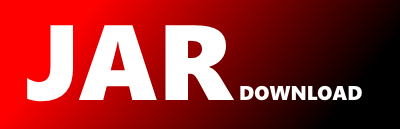
com.pulumi.azurenative.network.inputs.IpsecPolicyArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.inputs;
import com.pulumi.azurenative.network.enums.DhGroup;
import com.pulumi.azurenative.network.enums.IkeEncryption;
import com.pulumi.azurenative.network.enums.IkeIntegrity;
import com.pulumi.azurenative.network.enums.IpsecEncryption;
import com.pulumi.azurenative.network.enums.IpsecIntegrity;
import com.pulumi.azurenative.network.enums.PfsGroup;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
/**
* An IPSec Policy configuration for a virtual network gateway connection.
*
*/
public final class IpsecPolicyArgs extends com.pulumi.resources.ResourceArgs {
public static final IpsecPolicyArgs Empty = new IpsecPolicyArgs();
/**
* The DH Group used in IKE Phase 1 for initial SA.
*
*/
@Import(name="dhGroup", required=true)
private Output> dhGroup;
/**
* @return The DH Group used in IKE Phase 1 for initial SA.
*
*/
public Output> dhGroup() {
return this.dhGroup;
}
/**
* The IKE encryption algorithm (IKE phase 2).
*
*/
@Import(name="ikeEncryption", required=true)
private Output> ikeEncryption;
/**
* @return The IKE encryption algorithm (IKE phase 2).
*
*/
public Output> ikeEncryption() {
return this.ikeEncryption;
}
/**
* The IKE integrity algorithm (IKE phase 2).
*
*/
@Import(name="ikeIntegrity", required=true)
private Output> ikeIntegrity;
/**
* @return The IKE integrity algorithm (IKE phase 2).
*
*/
public Output> ikeIntegrity() {
return this.ikeIntegrity;
}
/**
* The IPSec encryption algorithm (IKE phase 1).
*
*/
@Import(name="ipsecEncryption", required=true)
private Output> ipsecEncryption;
/**
* @return The IPSec encryption algorithm (IKE phase 1).
*
*/
public Output> ipsecEncryption() {
return this.ipsecEncryption;
}
/**
* The IPSec integrity algorithm (IKE phase 1).
*
*/
@Import(name="ipsecIntegrity", required=true)
private Output> ipsecIntegrity;
/**
* @return The IPSec integrity algorithm (IKE phase 1).
*
*/
public Output> ipsecIntegrity() {
return this.ipsecIntegrity;
}
/**
* The Pfs Group used in IKE Phase 2 for new child SA.
*
*/
@Import(name="pfsGroup", required=true)
private Output> pfsGroup;
/**
* @return The Pfs Group used in IKE Phase 2 for new child SA.
*
*/
public Output> pfsGroup() {
return this.pfsGroup;
}
/**
* The IPSec Security Association (also called Quick Mode or Phase 2 SA) payload size in KB for a site to site VPN tunnel.
*
*/
@Import(name="saDataSizeKilobytes", required=true)
private Output saDataSizeKilobytes;
/**
* @return The IPSec Security Association (also called Quick Mode or Phase 2 SA) payload size in KB for a site to site VPN tunnel.
*
*/
public Output saDataSizeKilobytes() {
return this.saDataSizeKilobytes;
}
/**
* The IPSec Security Association (also called Quick Mode or Phase 2 SA) lifetime in seconds for a site to site VPN tunnel.
*
*/
@Import(name="saLifeTimeSeconds", required=true)
private Output saLifeTimeSeconds;
/**
* @return The IPSec Security Association (also called Quick Mode or Phase 2 SA) lifetime in seconds for a site to site VPN tunnel.
*
*/
public Output saLifeTimeSeconds() {
return this.saLifeTimeSeconds;
}
private IpsecPolicyArgs() {}
private IpsecPolicyArgs(IpsecPolicyArgs $) {
this.dhGroup = $.dhGroup;
this.ikeEncryption = $.ikeEncryption;
this.ikeIntegrity = $.ikeIntegrity;
this.ipsecEncryption = $.ipsecEncryption;
this.ipsecIntegrity = $.ipsecIntegrity;
this.pfsGroup = $.pfsGroup;
this.saDataSizeKilobytes = $.saDataSizeKilobytes;
this.saLifeTimeSeconds = $.saLifeTimeSeconds;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(IpsecPolicyArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private IpsecPolicyArgs $;
public Builder() {
$ = new IpsecPolicyArgs();
}
public Builder(IpsecPolicyArgs defaults) {
$ = new IpsecPolicyArgs(Objects.requireNonNull(defaults));
}
/**
* @param dhGroup The DH Group used in IKE Phase 1 for initial SA.
*
* @return builder
*
*/
public Builder dhGroup(Output> dhGroup) {
$.dhGroup = dhGroup;
return this;
}
/**
* @param dhGroup The DH Group used in IKE Phase 1 for initial SA.
*
* @return builder
*
*/
public Builder dhGroup(Either dhGroup) {
return dhGroup(Output.of(dhGroup));
}
/**
* @param dhGroup The DH Group used in IKE Phase 1 for initial SA.
*
* @return builder
*
*/
public Builder dhGroup(String dhGroup) {
return dhGroup(Either.ofLeft(dhGroup));
}
/**
* @param dhGroup The DH Group used in IKE Phase 1 for initial SA.
*
* @return builder
*
*/
public Builder dhGroup(DhGroup dhGroup) {
return dhGroup(Either.ofRight(dhGroup));
}
/**
* @param ikeEncryption The IKE encryption algorithm (IKE phase 2).
*
* @return builder
*
*/
public Builder ikeEncryption(Output> ikeEncryption) {
$.ikeEncryption = ikeEncryption;
return this;
}
/**
* @param ikeEncryption The IKE encryption algorithm (IKE phase 2).
*
* @return builder
*
*/
public Builder ikeEncryption(Either ikeEncryption) {
return ikeEncryption(Output.of(ikeEncryption));
}
/**
* @param ikeEncryption The IKE encryption algorithm (IKE phase 2).
*
* @return builder
*
*/
public Builder ikeEncryption(String ikeEncryption) {
return ikeEncryption(Either.ofLeft(ikeEncryption));
}
/**
* @param ikeEncryption The IKE encryption algorithm (IKE phase 2).
*
* @return builder
*
*/
public Builder ikeEncryption(IkeEncryption ikeEncryption) {
return ikeEncryption(Either.ofRight(ikeEncryption));
}
/**
* @param ikeIntegrity The IKE integrity algorithm (IKE phase 2).
*
* @return builder
*
*/
public Builder ikeIntegrity(Output> ikeIntegrity) {
$.ikeIntegrity = ikeIntegrity;
return this;
}
/**
* @param ikeIntegrity The IKE integrity algorithm (IKE phase 2).
*
* @return builder
*
*/
public Builder ikeIntegrity(Either ikeIntegrity) {
return ikeIntegrity(Output.of(ikeIntegrity));
}
/**
* @param ikeIntegrity The IKE integrity algorithm (IKE phase 2).
*
* @return builder
*
*/
public Builder ikeIntegrity(String ikeIntegrity) {
return ikeIntegrity(Either.ofLeft(ikeIntegrity));
}
/**
* @param ikeIntegrity The IKE integrity algorithm (IKE phase 2).
*
* @return builder
*
*/
public Builder ikeIntegrity(IkeIntegrity ikeIntegrity) {
return ikeIntegrity(Either.ofRight(ikeIntegrity));
}
/**
* @param ipsecEncryption The IPSec encryption algorithm (IKE phase 1).
*
* @return builder
*
*/
public Builder ipsecEncryption(Output> ipsecEncryption) {
$.ipsecEncryption = ipsecEncryption;
return this;
}
/**
* @param ipsecEncryption The IPSec encryption algorithm (IKE phase 1).
*
* @return builder
*
*/
public Builder ipsecEncryption(Either ipsecEncryption) {
return ipsecEncryption(Output.of(ipsecEncryption));
}
/**
* @param ipsecEncryption The IPSec encryption algorithm (IKE phase 1).
*
* @return builder
*
*/
public Builder ipsecEncryption(String ipsecEncryption) {
return ipsecEncryption(Either.ofLeft(ipsecEncryption));
}
/**
* @param ipsecEncryption The IPSec encryption algorithm (IKE phase 1).
*
* @return builder
*
*/
public Builder ipsecEncryption(IpsecEncryption ipsecEncryption) {
return ipsecEncryption(Either.ofRight(ipsecEncryption));
}
/**
* @param ipsecIntegrity The IPSec integrity algorithm (IKE phase 1).
*
* @return builder
*
*/
public Builder ipsecIntegrity(Output> ipsecIntegrity) {
$.ipsecIntegrity = ipsecIntegrity;
return this;
}
/**
* @param ipsecIntegrity The IPSec integrity algorithm (IKE phase 1).
*
* @return builder
*
*/
public Builder ipsecIntegrity(Either ipsecIntegrity) {
return ipsecIntegrity(Output.of(ipsecIntegrity));
}
/**
* @param ipsecIntegrity The IPSec integrity algorithm (IKE phase 1).
*
* @return builder
*
*/
public Builder ipsecIntegrity(String ipsecIntegrity) {
return ipsecIntegrity(Either.ofLeft(ipsecIntegrity));
}
/**
* @param ipsecIntegrity The IPSec integrity algorithm (IKE phase 1).
*
* @return builder
*
*/
public Builder ipsecIntegrity(IpsecIntegrity ipsecIntegrity) {
return ipsecIntegrity(Either.ofRight(ipsecIntegrity));
}
/**
* @param pfsGroup The Pfs Group used in IKE Phase 2 for new child SA.
*
* @return builder
*
*/
public Builder pfsGroup(Output> pfsGroup) {
$.pfsGroup = pfsGroup;
return this;
}
/**
* @param pfsGroup The Pfs Group used in IKE Phase 2 for new child SA.
*
* @return builder
*
*/
public Builder pfsGroup(Either pfsGroup) {
return pfsGroup(Output.of(pfsGroup));
}
/**
* @param pfsGroup The Pfs Group used in IKE Phase 2 for new child SA.
*
* @return builder
*
*/
public Builder pfsGroup(String pfsGroup) {
return pfsGroup(Either.ofLeft(pfsGroup));
}
/**
* @param pfsGroup The Pfs Group used in IKE Phase 2 for new child SA.
*
* @return builder
*
*/
public Builder pfsGroup(PfsGroup pfsGroup) {
return pfsGroup(Either.ofRight(pfsGroup));
}
/**
* @param saDataSizeKilobytes The IPSec Security Association (also called Quick Mode or Phase 2 SA) payload size in KB for a site to site VPN tunnel.
*
* @return builder
*
*/
public Builder saDataSizeKilobytes(Output saDataSizeKilobytes) {
$.saDataSizeKilobytes = saDataSizeKilobytes;
return this;
}
/**
* @param saDataSizeKilobytes The IPSec Security Association (also called Quick Mode or Phase 2 SA) payload size in KB for a site to site VPN tunnel.
*
* @return builder
*
*/
public Builder saDataSizeKilobytes(Integer saDataSizeKilobytes) {
return saDataSizeKilobytes(Output.of(saDataSizeKilobytes));
}
/**
* @param saLifeTimeSeconds The IPSec Security Association (also called Quick Mode or Phase 2 SA) lifetime in seconds for a site to site VPN tunnel.
*
* @return builder
*
*/
public Builder saLifeTimeSeconds(Output saLifeTimeSeconds) {
$.saLifeTimeSeconds = saLifeTimeSeconds;
return this;
}
/**
* @param saLifeTimeSeconds The IPSec Security Association (also called Quick Mode or Phase 2 SA) lifetime in seconds for a site to site VPN tunnel.
*
* @return builder
*
*/
public Builder saLifeTimeSeconds(Integer saLifeTimeSeconds) {
return saLifeTimeSeconds(Output.of(saLifeTimeSeconds));
}
public IpsecPolicyArgs build() {
if ($.dhGroup == null) {
throw new MissingRequiredPropertyException("IpsecPolicyArgs", "dhGroup");
}
if ($.ikeEncryption == null) {
throw new MissingRequiredPropertyException("IpsecPolicyArgs", "ikeEncryption");
}
if ($.ikeIntegrity == null) {
throw new MissingRequiredPropertyException("IpsecPolicyArgs", "ikeIntegrity");
}
if ($.ipsecEncryption == null) {
throw new MissingRequiredPropertyException("IpsecPolicyArgs", "ipsecEncryption");
}
if ($.ipsecIntegrity == null) {
throw new MissingRequiredPropertyException("IpsecPolicyArgs", "ipsecIntegrity");
}
if ($.pfsGroup == null) {
throw new MissingRequiredPropertyException("IpsecPolicyArgs", "pfsGroup");
}
if ($.saDataSizeKilobytes == null) {
throw new MissingRequiredPropertyException("IpsecPolicyArgs", "saDataSizeKilobytes");
}
if ($.saLifeTimeSeconds == null) {
throw new MissingRequiredPropertyException("IpsecPolicyArgs", "saLifeTimeSeconds");
}
return $;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy