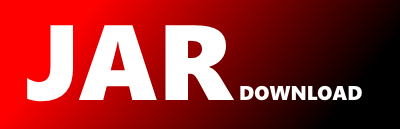
com.pulumi.azurenative.network.inputs.Ipv6ExpressRouteCircuitPeeringConfigArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.inputs;
import com.pulumi.azurenative.network.enums.ExpressRouteCircuitPeeringState;
import com.pulumi.azurenative.network.inputs.ExpressRouteCircuitPeeringConfigArgs;
import com.pulumi.azurenative.network.inputs.SubResourceArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Contains IPv6 peering config.
*
*/
public final class Ipv6ExpressRouteCircuitPeeringConfigArgs extends com.pulumi.resources.ResourceArgs {
public static final Ipv6ExpressRouteCircuitPeeringConfigArgs Empty = new Ipv6ExpressRouteCircuitPeeringConfigArgs();
/**
* The Microsoft peering configuration.
*
*/
@Import(name="microsoftPeeringConfig")
private @Nullable Output microsoftPeeringConfig;
/**
* @return The Microsoft peering configuration.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy