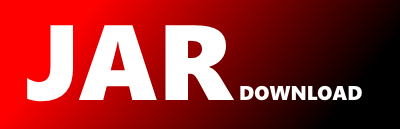
com.pulumi.azurenative.network.inputs.RulesEngineMatchConditionArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.inputs;
import com.pulumi.azurenative.network.enums.RulesEngineMatchVariable;
import com.pulumi.azurenative.network.enums.RulesEngineOperator;
import com.pulumi.azurenative.network.enums.Transform;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Define a match condition
*
*/
public final class RulesEngineMatchConditionArgs extends com.pulumi.resources.ResourceArgs {
public static final RulesEngineMatchConditionArgs Empty = new RulesEngineMatchConditionArgs();
/**
* Describes if this is negate condition or not
*
*/
@Import(name="negateCondition")
private @Nullable Output negateCondition;
/**
* @return Describes if this is negate condition or not
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy