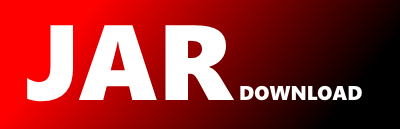
com.pulumi.azurenative.network.inputs.VirtualNetworkGatewayArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.inputs;
import com.pulumi.azurenative.network.enums.AdminState;
import com.pulumi.azurenative.network.enums.VirtualNetworkGatewayType;
import com.pulumi.azurenative.network.enums.VpnGatewayGeneration;
import com.pulumi.azurenative.network.enums.VpnType;
import com.pulumi.azurenative.network.inputs.AddressSpaceArgs;
import com.pulumi.azurenative.network.inputs.BgpSettingsArgs;
import com.pulumi.azurenative.network.inputs.ExtendedLocationArgs;
import com.pulumi.azurenative.network.inputs.SubResourceArgs;
import com.pulumi.azurenative.network.inputs.VirtualNetworkGatewayIPConfigurationArgs;
import com.pulumi.azurenative.network.inputs.VirtualNetworkGatewayNatRuleArgs;
import com.pulumi.azurenative.network.inputs.VirtualNetworkGatewayPolicyGroupArgs;
import com.pulumi.azurenative.network.inputs.VirtualNetworkGatewaySkuArgs;
import com.pulumi.azurenative.network.inputs.VpnClientConfigurationArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A common class for general resource information.
*
*/
public final class VirtualNetworkGatewayArgs extends com.pulumi.resources.ResourceArgs {
public static final VirtualNetworkGatewayArgs Empty = new VirtualNetworkGatewayArgs();
/**
* ActiveActive flag.
*
*/
@Import(name="activeActive")
private @Nullable Output activeActive;
/**
* @return ActiveActive flag.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy