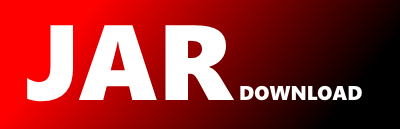
com.pulumi.azurenative.network.inputs.VpnClientConfigurationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.inputs;
import com.pulumi.azurenative.network.enums.VpnAuthenticationType;
import com.pulumi.azurenative.network.enums.VpnClientProtocol;
import com.pulumi.azurenative.network.inputs.AddressSpaceArgs;
import com.pulumi.azurenative.network.inputs.IpsecPolicyArgs;
import com.pulumi.azurenative.network.inputs.RadiusServerArgs;
import com.pulumi.azurenative.network.inputs.VngClientConnectionConfigurationArgs;
import com.pulumi.azurenative.network.inputs.VpnClientRevokedCertificateArgs;
import com.pulumi.azurenative.network.inputs.VpnClientRootCertificateArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* VpnClientConfiguration for P2S client.
*
*/
public final class VpnClientConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final VpnClientConfigurationArgs Empty = new VpnClientConfigurationArgs();
/**
* The AADAudience property of the VirtualNetworkGateway resource for vpn client connection used for AAD authentication.
*
*/
@Import(name="aadAudience")
private @Nullable Output aadAudience;
/**
* @return The AADAudience property of the VirtualNetworkGateway resource for vpn client connection used for AAD authentication.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy