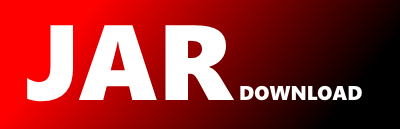
com.pulumi.azurenative.network.outputs.BackendResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class BackendResponse {
/**
* @return Location of the backend (IP address or FQDN)
*
*/
private @Nullable String address;
/**
* @return The value to use as the host header sent to the backend. If blank or unspecified, this defaults to the incoming host.
*
*/
private @Nullable String backendHostHeader;
/**
* @return Whether to enable use of this backend. Permitted values are 'Enabled' or 'Disabled'
*
*/
private @Nullable String enabledState;
/**
* @return The HTTP TCP port number. Must be between 1 and 65535.
*
*/
private @Nullable Integer httpPort;
/**
* @return The HTTPS TCP port number. Must be between 1 and 65535.
*
*/
private @Nullable Integer httpsPort;
/**
* @return Priority to use for load balancing. Higher priorities will not be used for load balancing if any lower priority backend is healthy.
*
*/
private @Nullable Integer priority;
/**
* @return The Approval status for the connection to the Private Link
*
*/
private String privateEndpointStatus;
/**
* @return The Alias of the Private Link resource. Populating this optional field indicates that this backend is 'Private'
*
*/
private @Nullable String privateLinkAlias;
/**
* @return A custom message to be included in the approval request to connect to the Private Link
*
*/
private @Nullable String privateLinkApprovalMessage;
/**
* @return The location of the Private Link resource. Required only if 'privateLinkResourceId' is populated
*
*/
private @Nullable String privateLinkLocation;
/**
* @return The Resource Id of the Private Link resource. Populating this optional field indicates that this backend is 'Private'
*
*/
private @Nullable String privateLinkResourceId;
/**
* @return Weight of this endpoint for load balancing purposes.
*
*/
private @Nullable Integer weight;
private BackendResponse() {}
/**
* @return Location of the backend (IP address or FQDN)
*
*/
public Optional address() {
return Optional.ofNullable(this.address);
}
/**
* @return The value to use as the host header sent to the backend. If blank or unspecified, this defaults to the incoming host.
*
*/
public Optional backendHostHeader() {
return Optional.ofNullable(this.backendHostHeader);
}
/**
* @return Whether to enable use of this backend. Permitted values are 'Enabled' or 'Disabled'
*
*/
public Optional enabledState() {
return Optional.ofNullable(this.enabledState);
}
/**
* @return The HTTP TCP port number. Must be between 1 and 65535.
*
*/
public Optional httpPort() {
return Optional.ofNullable(this.httpPort);
}
/**
* @return The HTTPS TCP port number. Must be between 1 and 65535.
*
*/
public Optional httpsPort() {
return Optional.ofNullable(this.httpsPort);
}
/**
* @return Priority to use for load balancing. Higher priorities will not be used for load balancing if any lower priority backend is healthy.
*
*/
public Optional priority() {
return Optional.ofNullable(this.priority);
}
/**
* @return The Approval status for the connection to the Private Link
*
*/
public String privateEndpointStatus() {
return this.privateEndpointStatus;
}
/**
* @return The Alias of the Private Link resource. Populating this optional field indicates that this backend is 'Private'
*
*/
public Optional privateLinkAlias() {
return Optional.ofNullable(this.privateLinkAlias);
}
/**
* @return A custom message to be included in the approval request to connect to the Private Link
*
*/
public Optional privateLinkApprovalMessage() {
return Optional.ofNullable(this.privateLinkApprovalMessage);
}
/**
* @return The location of the Private Link resource. Required only if 'privateLinkResourceId' is populated
*
*/
public Optional privateLinkLocation() {
return Optional.ofNullable(this.privateLinkLocation);
}
/**
* @return The Resource Id of the Private Link resource. Populating this optional field indicates that this backend is 'Private'
*
*/
public Optional privateLinkResourceId() {
return Optional.ofNullable(this.privateLinkResourceId);
}
/**
* @return Weight of this endpoint for load balancing purposes.
*
*/
public Optional weight() {
return Optional.ofNullable(this.weight);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(BackendResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String address;
private @Nullable String backendHostHeader;
private @Nullable String enabledState;
private @Nullable Integer httpPort;
private @Nullable Integer httpsPort;
private @Nullable Integer priority;
private String privateEndpointStatus;
private @Nullable String privateLinkAlias;
private @Nullable String privateLinkApprovalMessage;
private @Nullable String privateLinkLocation;
private @Nullable String privateLinkResourceId;
private @Nullable Integer weight;
public Builder() {}
public Builder(BackendResponse defaults) {
Objects.requireNonNull(defaults);
this.address = defaults.address;
this.backendHostHeader = defaults.backendHostHeader;
this.enabledState = defaults.enabledState;
this.httpPort = defaults.httpPort;
this.httpsPort = defaults.httpsPort;
this.priority = defaults.priority;
this.privateEndpointStatus = defaults.privateEndpointStatus;
this.privateLinkAlias = defaults.privateLinkAlias;
this.privateLinkApprovalMessage = defaults.privateLinkApprovalMessage;
this.privateLinkLocation = defaults.privateLinkLocation;
this.privateLinkResourceId = defaults.privateLinkResourceId;
this.weight = defaults.weight;
}
@CustomType.Setter
public Builder address(@Nullable String address) {
this.address = address;
return this;
}
@CustomType.Setter
public Builder backendHostHeader(@Nullable String backendHostHeader) {
this.backendHostHeader = backendHostHeader;
return this;
}
@CustomType.Setter
public Builder enabledState(@Nullable String enabledState) {
this.enabledState = enabledState;
return this;
}
@CustomType.Setter
public Builder httpPort(@Nullable Integer httpPort) {
this.httpPort = httpPort;
return this;
}
@CustomType.Setter
public Builder httpsPort(@Nullable Integer httpsPort) {
this.httpsPort = httpsPort;
return this;
}
@CustomType.Setter
public Builder priority(@Nullable Integer priority) {
this.priority = priority;
return this;
}
@CustomType.Setter
public Builder privateEndpointStatus(String privateEndpointStatus) {
if (privateEndpointStatus == null) {
throw new MissingRequiredPropertyException("BackendResponse", "privateEndpointStatus");
}
this.privateEndpointStatus = privateEndpointStatus;
return this;
}
@CustomType.Setter
public Builder privateLinkAlias(@Nullable String privateLinkAlias) {
this.privateLinkAlias = privateLinkAlias;
return this;
}
@CustomType.Setter
public Builder privateLinkApprovalMessage(@Nullable String privateLinkApprovalMessage) {
this.privateLinkApprovalMessage = privateLinkApprovalMessage;
return this;
}
@CustomType.Setter
public Builder privateLinkLocation(@Nullable String privateLinkLocation) {
this.privateLinkLocation = privateLinkLocation;
return this;
}
@CustomType.Setter
public Builder privateLinkResourceId(@Nullable String privateLinkResourceId) {
this.privateLinkResourceId = privateLinkResourceId;
return this;
}
@CustomType.Setter
public Builder weight(@Nullable Integer weight) {
this.weight = weight;
return this;
}
public BackendResponse build() {
final var _resultValue = new BackendResponse();
_resultValue.address = address;
_resultValue.backendHostHeader = backendHostHeader;
_resultValue.enabledState = enabledState;
_resultValue.httpPort = httpPort;
_resultValue.httpsPort = httpsPort;
_resultValue.priority = priority;
_resultValue.privateEndpointStatus = privateEndpointStatus;
_resultValue.privateLinkAlias = privateLinkAlias;
_resultValue.privateLinkApprovalMessage = privateLinkApprovalMessage;
_resultValue.privateLinkLocation = privateLinkLocation;
_resultValue.privateLinkResourceId = privateLinkResourceId;
_resultValue.weight = weight;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy