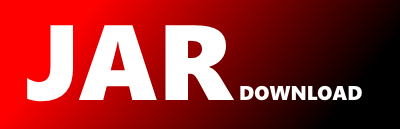
com.pulumi.azurenative.network.outputs.GetBastionHostResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.azurenative.network.outputs.BastionHostIPConfigurationResponse;
import com.pulumi.azurenative.network.outputs.SkuResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetBastionHostResult {
/**
* @return Enable/Disable Copy/Paste feature of the Bastion Host resource.
*
*/
private @Nullable Boolean disableCopyPaste;
/**
* @return FQDN for the endpoint on which bastion host is accessible.
*
*/
private @Nullable String dnsName;
/**
* @return Enable/Disable File Copy feature of the Bastion Host resource.
*
*/
private @Nullable Boolean enableFileCopy;
/**
* @return Enable/Disable IP Connect feature of the Bastion Host resource.
*
*/
private @Nullable Boolean enableIpConnect;
/**
* @return Enable/Disable Kerberos feature of the Bastion Host resource.
*
*/
private @Nullable Boolean enableKerberos;
/**
* @return Enable/Disable Shareable Link of the Bastion Host resource.
*
*/
private @Nullable Boolean enableShareableLink;
/**
* @return Enable/Disable Tunneling feature of the Bastion Host resource.
*
*/
private @Nullable Boolean enableTunneling;
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
private String etag;
/**
* @return Resource ID.
*
*/
private @Nullable String id;
/**
* @return IP configuration of the Bastion Host resource.
*
*/
private @Nullable List ipConfigurations;
/**
* @return Resource location.
*
*/
private @Nullable String location;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return The provisioning state of the bastion host resource.
*
*/
private String provisioningState;
/**
* @return The scale units for the Bastion Host resource.
*
*/
private @Nullable Integer scaleUnits;
/**
* @return The sku of this Bastion Host.
*
*/
private @Nullable SkuResponse sku;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Resource type.
*
*/
private String type;
private GetBastionHostResult() {}
/**
* @return Enable/Disable Copy/Paste feature of the Bastion Host resource.
*
*/
public Optional disableCopyPaste() {
return Optional.ofNullable(this.disableCopyPaste);
}
/**
* @return FQDN for the endpoint on which bastion host is accessible.
*
*/
public Optional dnsName() {
return Optional.ofNullable(this.dnsName);
}
/**
* @return Enable/Disable File Copy feature of the Bastion Host resource.
*
*/
public Optional enableFileCopy() {
return Optional.ofNullable(this.enableFileCopy);
}
/**
* @return Enable/Disable IP Connect feature of the Bastion Host resource.
*
*/
public Optional enableIpConnect() {
return Optional.ofNullable(this.enableIpConnect);
}
/**
* @return Enable/Disable Kerberos feature of the Bastion Host resource.
*
*/
public Optional enableKerberos() {
return Optional.ofNullable(this.enableKerberos);
}
/**
* @return Enable/Disable Shareable Link of the Bastion Host resource.
*
*/
public Optional enableShareableLink() {
return Optional.ofNullable(this.enableShareableLink);
}
/**
* @return Enable/Disable Tunneling feature of the Bastion Host resource.
*
*/
public Optional enableTunneling() {
return Optional.ofNullable(this.enableTunneling);
}
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Resource ID.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return IP configuration of the Bastion Host resource.
*
*/
public List ipConfigurations() {
return this.ipConfigurations == null ? List.of() : this.ipConfigurations;
}
/**
* @return Resource location.
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return The provisioning state of the bastion host resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The scale units for the Bastion Host resource.
*
*/
public Optional scaleUnits() {
return Optional.ofNullable(this.scaleUnits);
}
/**
* @return The sku of this Bastion Host.
*
*/
public Optional sku() {
return Optional.ofNullable(this.sku);
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetBastionHostResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean disableCopyPaste;
private @Nullable String dnsName;
private @Nullable Boolean enableFileCopy;
private @Nullable Boolean enableIpConnect;
private @Nullable Boolean enableKerberos;
private @Nullable Boolean enableShareableLink;
private @Nullable Boolean enableTunneling;
private String etag;
private @Nullable String id;
private @Nullable List ipConfigurations;
private @Nullable String location;
private String name;
private String provisioningState;
private @Nullable Integer scaleUnits;
private @Nullable SkuResponse sku;
private @Nullable Map tags;
private String type;
public Builder() {}
public Builder(GetBastionHostResult defaults) {
Objects.requireNonNull(defaults);
this.disableCopyPaste = defaults.disableCopyPaste;
this.dnsName = defaults.dnsName;
this.enableFileCopy = defaults.enableFileCopy;
this.enableIpConnect = defaults.enableIpConnect;
this.enableKerberos = defaults.enableKerberos;
this.enableShareableLink = defaults.enableShareableLink;
this.enableTunneling = defaults.enableTunneling;
this.etag = defaults.etag;
this.id = defaults.id;
this.ipConfigurations = defaults.ipConfigurations;
this.location = defaults.location;
this.name = defaults.name;
this.provisioningState = defaults.provisioningState;
this.scaleUnits = defaults.scaleUnits;
this.sku = defaults.sku;
this.tags = defaults.tags;
this.type = defaults.type;
}
@CustomType.Setter
public Builder disableCopyPaste(@Nullable Boolean disableCopyPaste) {
this.disableCopyPaste = disableCopyPaste;
return this;
}
@CustomType.Setter
public Builder dnsName(@Nullable String dnsName) {
this.dnsName = dnsName;
return this;
}
@CustomType.Setter
public Builder enableFileCopy(@Nullable Boolean enableFileCopy) {
this.enableFileCopy = enableFileCopy;
return this;
}
@CustomType.Setter
public Builder enableIpConnect(@Nullable Boolean enableIpConnect) {
this.enableIpConnect = enableIpConnect;
return this;
}
@CustomType.Setter
public Builder enableKerberos(@Nullable Boolean enableKerberos) {
this.enableKerberos = enableKerberos;
return this;
}
@CustomType.Setter
public Builder enableShareableLink(@Nullable Boolean enableShareableLink) {
this.enableShareableLink = enableShareableLink;
return this;
}
@CustomType.Setter
public Builder enableTunneling(@Nullable Boolean enableTunneling) {
this.enableTunneling = enableTunneling;
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("GetBastionHostResult", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder ipConfigurations(@Nullable List ipConfigurations) {
this.ipConfigurations = ipConfigurations;
return this;
}
public Builder ipConfigurations(BastionHostIPConfigurationResponse... ipConfigurations) {
return ipConfigurations(List.of(ipConfigurations));
}
@CustomType.Setter
public Builder location(@Nullable String location) {
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetBastionHostResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetBastionHostResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder scaleUnits(@Nullable Integer scaleUnits) {
this.scaleUnits = scaleUnits;
return this;
}
@CustomType.Setter
public Builder sku(@Nullable SkuResponse sku) {
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetBastionHostResult", "type");
}
this.type = type;
return this;
}
public GetBastionHostResult build() {
final var _resultValue = new GetBastionHostResult();
_resultValue.disableCopyPaste = disableCopyPaste;
_resultValue.dnsName = dnsName;
_resultValue.enableFileCopy = enableFileCopy;
_resultValue.enableIpConnect = enableIpConnect;
_resultValue.enableKerberos = enableKerberos;
_resultValue.enableShareableLink = enableShareableLink;
_resultValue.enableTunneling = enableTunneling;
_resultValue.etag = etag;
_resultValue.id = id;
_resultValue.ipConfigurations = ipConfigurations;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.provisioningState = provisioningState;
_resultValue.scaleUnits = scaleUnits;
_resultValue.sku = sku;
_resultValue.tags = tags;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy