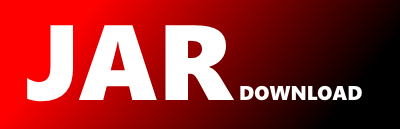
com.pulumi.azurenative.network.outputs.GetLoadBalancerResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.azurenative.network.outputs.BackendAddressPoolResponse;
import com.pulumi.azurenative.network.outputs.ExtendedLocationResponse;
import com.pulumi.azurenative.network.outputs.FrontendIPConfigurationResponse;
import com.pulumi.azurenative.network.outputs.InboundNatPoolResponse;
import com.pulumi.azurenative.network.outputs.InboundNatRuleResponse;
import com.pulumi.azurenative.network.outputs.LoadBalancerSkuResponse;
import com.pulumi.azurenative.network.outputs.LoadBalancingRuleResponse;
import com.pulumi.azurenative.network.outputs.OutboundRuleResponse;
import com.pulumi.azurenative.network.outputs.ProbeResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetLoadBalancerResult {
/**
* @return Collection of backend address pools used by a load balancer.
*
*/
private @Nullable List backendAddressPools;
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
private String etag;
/**
* @return The extended location of the load balancer.
*
*/
private @Nullable ExtendedLocationResponse extendedLocation;
/**
* @return Object representing the frontend IPs to be used for the load balancer.
*
*/
private @Nullable List frontendIPConfigurations;
/**
* @return Resource ID.
*
*/
private @Nullable String id;
/**
* @return Defines an external port range for inbound NAT to a single backend port on NICs associated with a load balancer. Inbound NAT rules are created automatically for each NIC associated with the Load Balancer using an external port from this range. Defining an Inbound NAT pool on your Load Balancer is mutually exclusive with defining inbound NAT rules. Inbound NAT pools are referenced from virtual machine scale sets. NICs that are associated with individual virtual machines cannot reference an inbound NAT pool. They have to reference individual inbound NAT rules.
*
*/
private @Nullable List inboundNatPools;
/**
* @return Collection of inbound NAT Rules used by a load balancer. Defining inbound NAT rules on your load balancer is mutually exclusive with defining an inbound NAT pool. Inbound NAT pools are referenced from virtual machine scale sets. NICs that are associated with individual virtual machines cannot reference an Inbound NAT pool. They have to reference individual inbound NAT rules.
*
*/
private @Nullable List inboundNatRules;
/**
* @return Object collection representing the load balancing rules Gets the provisioning.
*
*/
private @Nullable List loadBalancingRules;
/**
* @return Resource location.
*
*/
private @Nullable String location;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return The outbound rules.
*
*/
private @Nullable List outboundRules;
/**
* @return Collection of probe objects used in the load balancer.
*
*/
private @Nullable List probes;
/**
* @return The provisioning state of the load balancer resource.
*
*/
private String provisioningState;
/**
* @return The resource GUID property of the load balancer resource.
*
*/
private String resourceGuid;
/**
* @return The load balancer SKU.
*
*/
private @Nullable LoadBalancerSkuResponse sku;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Resource type.
*
*/
private String type;
private GetLoadBalancerResult() {}
/**
* @return Collection of backend address pools used by a load balancer.
*
*/
public List backendAddressPools() {
return this.backendAddressPools == null ? List.of() : this.backendAddressPools;
}
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public String etag() {
return this.etag;
}
/**
* @return The extended location of the load balancer.
*
*/
public Optional extendedLocation() {
return Optional.ofNullable(this.extendedLocation);
}
/**
* @return Object representing the frontend IPs to be used for the load balancer.
*
*/
public List frontendIPConfigurations() {
return this.frontendIPConfigurations == null ? List.of() : this.frontendIPConfigurations;
}
/**
* @return Resource ID.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return Defines an external port range for inbound NAT to a single backend port on NICs associated with a load balancer. Inbound NAT rules are created automatically for each NIC associated with the Load Balancer using an external port from this range. Defining an Inbound NAT pool on your Load Balancer is mutually exclusive with defining inbound NAT rules. Inbound NAT pools are referenced from virtual machine scale sets. NICs that are associated with individual virtual machines cannot reference an inbound NAT pool. They have to reference individual inbound NAT rules.
*
*/
public List inboundNatPools() {
return this.inboundNatPools == null ? List.of() : this.inboundNatPools;
}
/**
* @return Collection of inbound NAT Rules used by a load balancer. Defining inbound NAT rules on your load balancer is mutually exclusive with defining an inbound NAT pool. Inbound NAT pools are referenced from virtual machine scale sets. NICs that are associated with individual virtual machines cannot reference an Inbound NAT pool. They have to reference individual inbound NAT rules.
*
*/
public List inboundNatRules() {
return this.inboundNatRules == null ? List.of() : this.inboundNatRules;
}
/**
* @return Object collection representing the load balancing rules Gets the provisioning.
*
*/
public List loadBalancingRules() {
return this.loadBalancingRules == null ? List.of() : this.loadBalancingRules;
}
/**
* @return Resource location.
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return The outbound rules.
*
*/
public List outboundRules() {
return this.outboundRules == null ? List.of() : this.outboundRules;
}
/**
* @return Collection of probe objects used in the load balancer.
*
*/
public List probes() {
return this.probes == null ? List.of() : this.probes;
}
/**
* @return The provisioning state of the load balancer resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The resource GUID property of the load balancer resource.
*
*/
public String resourceGuid() {
return this.resourceGuid;
}
/**
* @return The load balancer SKU.
*
*/
public Optional sku() {
return Optional.ofNullable(this.sku);
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetLoadBalancerResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List backendAddressPools;
private String etag;
private @Nullable ExtendedLocationResponse extendedLocation;
private @Nullable List frontendIPConfigurations;
private @Nullable String id;
private @Nullable List inboundNatPools;
private @Nullable List inboundNatRules;
private @Nullable List loadBalancingRules;
private @Nullable String location;
private String name;
private @Nullable List outboundRules;
private @Nullable List probes;
private String provisioningState;
private String resourceGuid;
private @Nullable LoadBalancerSkuResponse sku;
private @Nullable Map tags;
private String type;
public Builder() {}
public Builder(GetLoadBalancerResult defaults) {
Objects.requireNonNull(defaults);
this.backendAddressPools = defaults.backendAddressPools;
this.etag = defaults.etag;
this.extendedLocation = defaults.extendedLocation;
this.frontendIPConfigurations = defaults.frontendIPConfigurations;
this.id = defaults.id;
this.inboundNatPools = defaults.inboundNatPools;
this.inboundNatRules = defaults.inboundNatRules;
this.loadBalancingRules = defaults.loadBalancingRules;
this.location = defaults.location;
this.name = defaults.name;
this.outboundRules = defaults.outboundRules;
this.probes = defaults.probes;
this.provisioningState = defaults.provisioningState;
this.resourceGuid = defaults.resourceGuid;
this.sku = defaults.sku;
this.tags = defaults.tags;
this.type = defaults.type;
}
@CustomType.Setter
public Builder backendAddressPools(@Nullable List backendAddressPools) {
this.backendAddressPools = backendAddressPools;
return this;
}
public Builder backendAddressPools(BackendAddressPoolResponse... backendAddressPools) {
return backendAddressPools(List.of(backendAddressPools));
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("GetLoadBalancerResult", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder extendedLocation(@Nullable ExtendedLocationResponse extendedLocation) {
this.extendedLocation = extendedLocation;
return this;
}
@CustomType.Setter
public Builder frontendIPConfigurations(@Nullable List frontendIPConfigurations) {
this.frontendIPConfigurations = frontendIPConfigurations;
return this;
}
public Builder frontendIPConfigurations(FrontendIPConfigurationResponse... frontendIPConfigurations) {
return frontendIPConfigurations(List.of(frontendIPConfigurations));
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder inboundNatPools(@Nullable List inboundNatPools) {
this.inboundNatPools = inboundNatPools;
return this;
}
public Builder inboundNatPools(InboundNatPoolResponse... inboundNatPools) {
return inboundNatPools(List.of(inboundNatPools));
}
@CustomType.Setter
public Builder inboundNatRules(@Nullable List inboundNatRules) {
this.inboundNatRules = inboundNatRules;
return this;
}
public Builder inboundNatRules(InboundNatRuleResponse... inboundNatRules) {
return inboundNatRules(List.of(inboundNatRules));
}
@CustomType.Setter
public Builder loadBalancingRules(@Nullable List loadBalancingRules) {
this.loadBalancingRules = loadBalancingRules;
return this;
}
public Builder loadBalancingRules(LoadBalancingRuleResponse... loadBalancingRules) {
return loadBalancingRules(List.of(loadBalancingRules));
}
@CustomType.Setter
public Builder location(@Nullable String location) {
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetLoadBalancerResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder outboundRules(@Nullable List outboundRules) {
this.outboundRules = outboundRules;
return this;
}
public Builder outboundRules(OutboundRuleResponse... outboundRules) {
return outboundRules(List.of(outboundRules));
}
@CustomType.Setter
public Builder probes(@Nullable List probes) {
this.probes = probes;
return this;
}
public Builder probes(ProbeResponse... probes) {
return probes(List.of(probes));
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetLoadBalancerResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder resourceGuid(String resourceGuid) {
if (resourceGuid == null) {
throw new MissingRequiredPropertyException("GetLoadBalancerResult", "resourceGuid");
}
this.resourceGuid = resourceGuid;
return this;
}
@CustomType.Setter
public Builder sku(@Nullable LoadBalancerSkuResponse sku) {
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetLoadBalancerResult", "type");
}
this.type = type;
return this;
}
public GetLoadBalancerResult build() {
final var _resultValue = new GetLoadBalancerResult();
_resultValue.backendAddressPools = backendAddressPools;
_resultValue.etag = etag;
_resultValue.extendedLocation = extendedLocation;
_resultValue.frontendIPConfigurations = frontendIPConfigurations;
_resultValue.id = id;
_resultValue.inboundNatPools = inboundNatPools;
_resultValue.inboundNatRules = inboundNatRules;
_resultValue.loadBalancingRules = loadBalancingRules;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.outboundRules = outboundRules;
_resultValue.probes = probes;
_resultValue.provisioningState = provisioningState;
_resultValue.resourceGuid = resourceGuid;
_resultValue.sku = sku;
_resultValue.tags = tags;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy