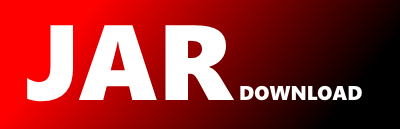
com.pulumi.azurenative.network.outputs.GetPacketCaptureResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.azurenative.network.outputs.PacketCaptureFilterResponse;
import com.pulumi.azurenative.network.outputs.PacketCaptureMachineScopeResponse;
import com.pulumi.azurenative.network.outputs.PacketCaptureStorageLocationResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetPacketCaptureResult {
/**
* @return Number of bytes captured per packet, the remaining bytes are truncated.
*
*/
private @Nullable Double bytesToCapturePerPacket;
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
private String etag;
/**
* @return A list of packet capture filters.
*
*/
private @Nullable List filters;
/**
* @return ID of the packet capture operation.
*
*/
private String id;
/**
* @return Name of the packet capture session.
*
*/
private String name;
/**
* @return The provisioning state of the packet capture session.
*
*/
private String provisioningState;
/**
* @return A list of AzureVMSS instances which can be included or excluded to run packet capture. If both included and excluded are empty, then the packet capture will run on all instances of AzureVMSS.
*
*/
private @Nullable PacketCaptureMachineScopeResponse scope;
/**
* @return The storage location for a packet capture session.
*
*/
private PacketCaptureStorageLocationResponse storageLocation;
/**
* @return The ID of the targeted resource, only AzureVM and AzureVMSS as target type are currently supported.
*
*/
private String target;
/**
* @return Target type of the resource provided.
*
*/
private @Nullable String targetType;
/**
* @return Maximum duration of the capture session in seconds.
*
*/
private @Nullable Integer timeLimitInSeconds;
/**
* @return Maximum size of the capture output.
*
*/
private @Nullable Double totalBytesPerSession;
private GetPacketCaptureResult() {}
/**
* @return Number of bytes captured per packet, the remaining bytes are truncated.
*
*/
public Optional bytesToCapturePerPacket() {
return Optional.ofNullable(this.bytesToCapturePerPacket);
}
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public String etag() {
return this.etag;
}
/**
* @return A list of packet capture filters.
*
*/
public List filters() {
return this.filters == null ? List.of() : this.filters;
}
/**
* @return ID of the packet capture operation.
*
*/
public String id() {
return this.id;
}
/**
* @return Name of the packet capture session.
*
*/
public String name() {
return this.name;
}
/**
* @return The provisioning state of the packet capture session.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return A list of AzureVMSS instances which can be included or excluded to run packet capture. If both included and excluded are empty, then the packet capture will run on all instances of AzureVMSS.
*
*/
public Optional scope() {
return Optional.ofNullable(this.scope);
}
/**
* @return The storage location for a packet capture session.
*
*/
public PacketCaptureStorageLocationResponse storageLocation() {
return this.storageLocation;
}
/**
* @return The ID of the targeted resource, only AzureVM and AzureVMSS as target type are currently supported.
*
*/
public String target() {
return this.target;
}
/**
* @return Target type of the resource provided.
*
*/
public Optional targetType() {
return Optional.ofNullable(this.targetType);
}
/**
* @return Maximum duration of the capture session in seconds.
*
*/
public Optional timeLimitInSeconds() {
return Optional.ofNullable(this.timeLimitInSeconds);
}
/**
* @return Maximum size of the capture output.
*
*/
public Optional totalBytesPerSession() {
return Optional.ofNullable(this.totalBytesPerSession);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetPacketCaptureResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Double bytesToCapturePerPacket;
private String etag;
private @Nullable List filters;
private String id;
private String name;
private String provisioningState;
private @Nullable PacketCaptureMachineScopeResponse scope;
private PacketCaptureStorageLocationResponse storageLocation;
private String target;
private @Nullable String targetType;
private @Nullable Integer timeLimitInSeconds;
private @Nullable Double totalBytesPerSession;
public Builder() {}
public Builder(GetPacketCaptureResult defaults) {
Objects.requireNonNull(defaults);
this.bytesToCapturePerPacket = defaults.bytesToCapturePerPacket;
this.etag = defaults.etag;
this.filters = defaults.filters;
this.id = defaults.id;
this.name = defaults.name;
this.provisioningState = defaults.provisioningState;
this.scope = defaults.scope;
this.storageLocation = defaults.storageLocation;
this.target = defaults.target;
this.targetType = defaults.targetType;
this.timeLimitInSeconds = defaults.timeLimitInSeconds;
this.totalBytesPerSession = defaults.totalBytesPerSession;
}
@CustomType.Setter
public Builder bytesToCapturePerPacket(@Nullable Double bytesToCapturePerPacket) {
this.bytesToCapturePerPacket = bytesToCapturePerPacket;
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("GetPacketCaptureResult", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder filters(@Nullable List filters) {
this.filters = filters;
return this;
}
public Builder filters(PacketCaptureFilterResponse... filters) {
return filters(List.of(filters));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetPacketCaptureResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetPacketCaptureResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetPacketCaptureResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder scope(@Nullable PacketCaptureMachineScopeResponse scope) {
this.scope = scope;
return this;
}
@CustomType.Setter
public Builder storageLocation(PacketCaptureStorageLocationResponse storageLocation) {
if (storageLocation == null) {
throw new MissingRequiredPropertyException("GetPacketCaptureResult", "storageLocation");
}
this.storageLocation = storageLocation;
return this;
}
@CustomType.Setter
public Builder target(String target) {
if (target == null) {
throw new MissingRequiredPropertyException("GetPacketCaptureResult", "target");
}
this.target = target;
return this;
}
@CustomType.Setter
public Builder targetType(@Nullable String targetType) {
this.targetType = targetType;
return this;
}
@CustomType.Setter
public Builder timeLimitInSeconds(@Nullable Integer timeLimitInSeconds) {
this.timeLimitInSeconds = timeLimitInSeconds;
return this;
}
@CustomType.Setter
public Builder totalBytesPerSession(@Nullable Double totalBytesPerSession) {
this.totalBytesPerSession = totalBytesPerSession;
return this;
}
public GetPacketCaptureResult build() {
final var _resultValue = new GetPacketCaptureResult();
_resultValue.bytesToCapturePerPacket = bytesToCapturePerPacket;
_resultValue.etag = etag;
_resultValue.filters = filters;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.provisioningState = provisioningState;
_resultValue.scope = scope;
_resultValue.storageLocation = storageLocation;
_resultValue.target = target;
_resultValue.targetType = targetType;
_resultValue.timeLimitInSeconds = timeLimitInSeconds;
_resultValue.totalBytesPerSession = totalBytesPerSession;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy