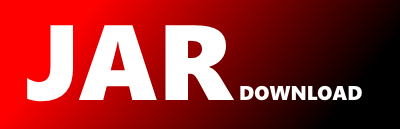
com.pulumi.azurenative.network.outputs.GetVirtualNetworkPeeringResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.azurenative.network.outputs.AddressSpaceResponse;
import com.pulumi.azurenative.network.outputs.SubResourceResponse;
import com.pulumi.azurenative.network.outputs.VirtualNetworkBgpCommunitiesResponse;
import com.pulumi.azurenative.network.outputs.VirtualNetworkEncryptionResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetVirtualNetworkPeeringResult {
/**
* @return Whether the forwarded traffic from the VMs in the local virtual network will be allowed/disallowed in remote virtual network.
*
*/
private @Nullable Boolean allowForwardedTraffic;
/**
* @return If gateway links can be used in remote virtual networking to link to this virtual network.
*
*/
private @Nullable Boolean allowGatewayTransit;
/**
* @return Whether the VMs in the local virtual network space would be able to access the VMs in remote virtual network space.
*
*/
private @Nullable Boolean allowVirtualNetworkAccess;
/**
* @return If we need to verify the provisioning state of the remote gateway.
*
*/
private @Nullable Boolean doNotVerifyRemoteGateways;
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
private String etag;
/**
* @return Resource ID.
*
*/
private @Nullable String id;
/**
* @return The name of the resource that is unique within a resource group. This name can be used to access the resource.
*
*/
private @Nullable String name;
/**
* @return The status of the virtual network peering.
*
*/
private @Nullable String peeringState;
/**
* @return The peering sync status of the virtual network peering.
*
*/
private @Nullable String peeringSyncLevel;
/**
* @return The provisioning state of the virtual network peering resource.
*
*/
private String provisioningState;
/**
* @return The reference to the address space peered with the remote virtual network.
*
*/
private @Nullable AddressSpaceResponse remoteAddressSpace;
/**
* @return The reference to the remote virtual network's Bgp Communities.
*
*/
private @Nullable VirtualNetworkBgpCommunitiesResponse remoteBgpCommunities;
/**
* @return The reference to the remote virtual network. The remote virtual network can be in the same or different region (preview). See here to register for the preview and learn more (https://docs.microsoft.com/en-us/azure/virtual-network/virtual-network-create-peering).
*
*/
private @Nullable SubResourceResponse remoteVirtualNetwork;
/**
* @return The reference to the current address space of the remote virtual network.
*
*/
private @Nullable AddressSpaceResponse remoteVirtualNetworkAddressSpace;
/**
* @return The reference to the remote virtual network's encryption
*
*/
private VirtualNetworkEncryptionResponse remoteVirtualNetworkEncryption;
/**
* @return The resourceGuid property of the Virtual Network peering resource.
*
*/
private String resourceGuid;
/**
* @return Resource type.
*
*/
private @Nullable String type;
/**
* @return If remote gateways can be used on this virtual network. If the flag is set to true, and allowGatewayTransit on remote peering is also true, virtual network will use gateways of remote virtual network for transit. Only one peering can have this flag set to true. This flag cannot be set if virtual network already has a gateway.
*
*/
private @Nullable Boolean useRemoteGateways;
private GetVirtualNetworkPeeringResult() {}
/**
* @return Whether the forwarded traffic from the VMs in the local virtual network will be allowed/disallowed in remote virtual network.
*
*/
public Optional allowForwardedTraffic() {
return Optional.ofNullable(this.allowForwardedTraffic);
}
/**
* @return If gateway links can be used in remote virtual networking to link to this virtual network.
*
*/
public Optional allowGatewayTransit() {
return Optional.ofNullable(this.allowGatewayTransit);
}
/**
* @return Whether the VMs in the local virtual network space would be able to access the VMs in remote virtual network space.
*
*/
public Optional allowVirtualNetworkAccess() {
return Optional.ofNullable(this.allowVirtualNetworkAccess);
}
/**
* @return If we need to verify the provisioning state of the remote gateway.
*
*/
public Optional doNotVerifyRemoteGateways() {
return Optional.ofNullable(this.doNotVerifyRemoteGateways);
}
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Resource ID.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return The name of the resource that is unique within a resource group. This name can be used to access the resource.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return The status of the virtual network peering.
*
*/
public Optional peeringState() {
return Optional.ofNullable(this.peeringState);
}
/**
* @return The peering sync status of the virtual network peering.
*
*/
public Optional peeringSyncLevel() {
return Optional.ofNullable(this.peeringSyncLevel);
}
/**
* @return The provisioning state of the virtual network peering resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The reference to the address space peered with the remote virtual network.
*
*/
public Optional remoteAddressSpace() {
return Optional.ofNullable(this.remoteAddressSpace);
}
/**
* @return The reference to the remote virtual network's Bgp Communities.
*
*/
public Optional remoteBgpCommunities() {
return Optional.ofNullable(this.remoteBgpCommunities);
}
/**
* @return The reference to the remote virtual network. The remote virtual network can be in the same or different region (preview). See here to register for the preview and learn more (https://docs.microsoft.com/en-us/azure/virtual-network/virtual-network-create-peering).
*
*/
public Optional remoteVirtualNetwork() {
return Optional.ofNullable(this.remoteVirtualNetwork);
}
/**
* @return The reference to the current address space of the remote virtual network.
*
*/
public Optional remoteVirtualNetworkAddressSpace() {
return Optional.ofNullable(this.remoteVirtualNetworkAddressSpace);
}
/**
* @return The reference to the remote virtual network's encryption
*
*/
public VirtualNetworkEncryptionResponse remoteVirtualNetworkEncryption() {
return this.remoteVirtualNetworkEncryption;
}
/**
* @return The resourceGuid property of the Virtual Network peering resource.
*
*/
public String resourceGuid() {
return this.resourceGuid;
}
/**
* @return Resource type.
*
*/
public Optional type() {
return Optional.ofNullable(this.type);
}
/**
* @return If remote gateways can be used on this virtual network. If the flag is set to true, and allowGatewayTransit on remote peering is also true, virtual network will use gateways of remote virtual network for transit. Only one peering can have this flag set to true. This flag cannot be set if virtual network already has a gateway.
*
*/
public Optional useRemoteGateways() {
return Optional.ofNullable(this.useRemoteGateways);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetVirtualNetworkPeeringResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean allowForwardedTraffic;
private @Nullable Boolean allowGatewayTransit;
private @Nullable Boolean allowVirtualNetworkAccess;
private @Nullable Boolean doNotVerifyRemoteGateways;
private String etag;
private @Nullable String id;
private @Nullable String name;
private @Nullable String peeringState;
private @Nullable String peeringSyncLevel;
private String provisioningState;
private @Nullable AddressSpaceResponse remoteAddressSpace;
private @Nullable VirtualNetworkBgpCommunitiesResponse remoteBgpCommunities;
private @Nullable SubResourceResponse remoteVirtualNetwork;
private @Nullable AddressSpaceResponse remoteVirtualNetworkAddressSpace;
private VirtualNetworkEncryptionResponse remoteVirtualNetworkEncryption;
private String resourceGuid;
private @Nullable String type;
private @Nullable Boolean useRemoteGateways;
public Builder() {}
public Builder(GetVirtualNetworkPeeringResult defaults) {
Objects.requireNonNull(defaults);
this.allowForwardedTraffic = defaults.allowForwardedTraffic;
this.allowGatewayTransit = defaults.allowGatewayTransit;
this.allowVirtualNetworkAccess = defaults.allowVirtualNetworkAccess;
this.doNotVerifyRemoteGateways = defaults.doNotVerifyRemoteGateways;
this.etag = defaults.etag;
this.id = defaults.id;
this.name = defaults.name;
this.peeringState = defaults.peeringState;
this.peeringSyncLevel = defaults.peeringSyncLevel;
this.provisioningState = defaults.provisioningState;
this.remoteAddressSpace = defaults.remoteAddressSpace;
this.remoteBgpCommunities = defaults.remoteBgpCommunities;
this.remoteVirtualNetwork = defaults.remoteVirtualNetwork;
this.remoteVirtualNetworkAddressSpace = defaults.remoteVirtualNetworkAddressSpace;
this.remoteVirtualNetworkEncryption = defaults.remoteVirtualNetworkEncryption;
this.resourceGuid = defaults.resourceGuid;
this.type = defaults.type;
this.useRemoteGateways = defaults.useRemoteGateways;
}
@CustomType.Setter
public Builder allowForwardedTraffic(@Nullable Boolean allowForwardedTraffic) {
this.allowForwardedTraffic = allowForwardedTraffic;
return this;
}
@CustomType.Setter
public Builder allowGatewayTransit(@Nullable Boolean allowGatewayTransit) {
this.allowGatewayTransit = allowGatewayTransit;
return this;
}
@CustomType.Setter
public Builder allowVirtualNetworkAccess(@Nullable Boolean allowVirtualNetworkAccess) {
this.allowVirtualNetworkAccess = allowVirtualNetworkAccess;
return this;
}
@CustomType.Setter
public Builder doNotVerifyRemoteGateways(@Nullable Boolean doNotVerifyRemoteGateways) {
this.doNotVerifyRemoteGateways = doNotVerifyRemoteGateways;
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("GetVirtualNetworkPeeringResult", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder peeringState(@Nullable String peeringState) {
this.peeringState = peeringState;
return this;
}
@CustomType.Setter
public Builder peeringSyncLevel(@Nullable String peeringSyncLevel) {
this.peeringSyncLevel = peeringSyncLevel;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetVirtualNetworkPeeringResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder remoteAddressSpace(@Nullable AddressSpaceResponse remoteAddressSpace) {
this.remoteAddressSpace = remoteAddressSpace;
return this;
}
@CustomType.Setter
public Builder remoteBgpCommunities(@Nullable VirtualNetworkBgpCommunitiesResponse remoteBgpCommunities) {
this.remoteBgpCommunities = remoteBgpCommunities;
return this;
}
@CustomType.Setter
public Builder remoteVirtualNetwork(@Nullable SubResourceResponse remoteVirtualNetwork) {
this.remoteVirtualNetwork = remoteVirtualNetwork;
return this;
}
@CustomType.Setter
public Builder remoteVirtualNetworkAddressSpace(@Nullable AddressSpaceResponse remoteVirtualNetworkAddressSpace) {
this.remoteVirtualNetworkAddressSpace = remoteVirtualNetworkAddressSpace;
return this;
}
@CustomType.Setter
public Builder remoteVirtualNetworkEncryption(VirtualNetworkEncryptionResponse remoteVirtualNetworkEncryption) {
if (remoteVirtualNetworkEncryption == null) {
throw new MissingRequiredPropertyException("GetVirtualNetworkPeeringResult", "remoteVirtualNetworkEncryption");
}
this.remoteVirtualNetworkEncryption = remoteVirtualNetworkEncryption;
return this;
}
@CustomType.Setter
public Builder resourceGuid(String resourceGuid) {
if (resourceGuid == null) {
throw new MissingRequiredPropertyException("GetVirtualNetworkPeeringResult", "resourceGuid");
}
this.resourceGuid = resourceGuid;
return this;
}
@CustomType.Setter
public Builder type(@Nullable String type) {
this.type = type;
return this;
}
@CustomType.Setter
public Builder useRemoteGateways(@Nullable Boolean useRemoteGateways) {
this.useRemoteGateways = useRemoteGateways;
return this;
}
public GetVirtualNetworkPeeringResult build() {
final var _resultValue = new GetVirtualNetworkPeeringResult();
_resultValue.allowForwardedTraffic = allowForwardedTraffic;
_resultValue.allowGatewayTransit = allowGatewayTransit;
_resultValue.allowVirtualNetworkAccess = allowVirtualNetworkAccess;
_resultValue.doNotVerifyRemoteGateways = doNotVerifyRemoteGateways;
_resultValue.etag = etag;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.peeringState = peeringState;
_resultValue.peeringSyncLevel = peeringSyncLevel;
_resultValue.provisioningState = provisioningState;
_resultValue.remoteAddressSpace = remoteAddressSpace;
_resultValue.remoteBgpCommunities = remoteBgpCommunities;
_resultValue.remoteVirtualNetwork = remoteVirtualNetwork;
_resultValue.remoteVirtualNetworkAddressSpace = remoteVirtualNetworkAddressSpace;
_resultValue.remoteVirtualNetworkEncryption = remoteVirtualNetworkEncryption;
_resultValue.resourceGuid = resourceGuid;
_resultValue.type = type;
_resultValue.useRemoteGateways = useRemoteGateways;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy