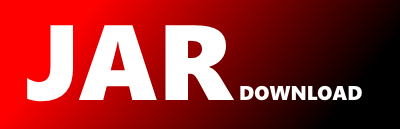
com.pulumi.azurenative.network.outputs.GetVpnConnectionResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.azurenative.network.outputs.IpsecPolicyResponse;
import com.pulumi.azurenative.network.outputs.RoutingConfigurationResponse;
import com.pulumi.azurenative.network.outputs.SubResourceResponse;
import com.pulumi.azurenative.network.outputs.TrafficSelectorPolicyResponse;
import com.pulumi.azurenative.network.outputs.VpnSiteLinkConnectionResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetVpnConnectionResult {
/**
* @return Expected bandwidth in MBPS.
*
*/
private @Nullable Integer connectionBandwidth;
/**
* @return The connection status.
*
*/
private String connectionStatus;
/**
* @return DPD timeout in seconds for vpn connection.
*
*/
private @Nullable Integer dpdTimeoutSeconds;
/**
* @return Egress bytes transferred.
*
*/
private Double egressBytesTransferred;
/**
* @return EnableBgp flag.
*
*/
private @Nullable Boolean enableBgp;
/**
* @return Enable internet security.
*
*/
private @Nullable Boolean enableInternetSecurity;
/**
* @return EnableBgp flag.
*
*/
private @Nullable Boolean enableRateLimiting;
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
private String etag;
/**
* @return Resource ID.
*
*/
private @Nullable String id;
/**
* @return Ingress bytes transferred.
*
*/
private Double ingressBytesTransferred;
/**
* @return The IPSec Policies to be considered by this connection.
*
*/
private @Nullable List ipsecPolicies;
/**
* @return The name of the resource that is unique within a resource group. This name can be used to access the resource.
*
*/
private @Nullable String name;
/**
* @return The provisioning state of the VPN connection resource.
*
*/
private String provisioningState;
/**
* @return Id of the connected vpn site.
*
*/
private @Nullable SubResourceResponse remoteVpnSite;
/**
* @return The Routing Configuration indicating the associated and propagated route tables on this connection.
*
*/
private @Nullable RoutingConfigurationResponse routingConfiguration;
/**
* @return Routing weight for vpn connection.
*
*/
private @Nullable Integer routingWeight;
/**
* @return SharedKey for the vpn connection.
*
*/
private @Nullable String sharedKey;
/**
* @return The Traffic Selector Policies to be considered by this connection.
*
*/
private @Nullable List trafficSelectorPolicies;
/**
* @return Use local azure ip to initiate connection.
*
*/
private @Nullable Boolean useLocalAzureIpAddress;
/**
* @return Enable policy-based traffic selectors.
*
*/
private @Nullable Boolean usePolicyBasedTrafficSelectors;
/**
* @return Connection protocol used for this connection.
*
*/
private @Nullable String vpnConnectionProtocolType;
/**
* @return List of all vpn site link connections to the gateway.
*
*/
private @Nullable List vpnLinkConnections;
private GetVpnConnectionResult() {}
/**
* @return Expected bandwidth in MBPS.
*
*/
public Optional connectionBandwidth() {
return Optional.ofNullable(this.connectionBandwidth);
}
/**
* @return The connection status.
*
*/
public String connectionStatus() {
return this.connectionStatus;
}
/**
* @return DPD timeout in seconds for vpn connection.
*
*/
public Optional dpdTimeoutSeconds() {
return Optional.ofNullable(this.dpdTimeoutSeconds);
}
/**
* @return Egress bytes transferred.
*
*/
public Double egressBytesTransferred() {
return this.egressBytesTransferred;
}
/**
* @return EnableBgp flag.
*
*/
public Optional enableBgp() {
return Optional.ofNullable(this.enableBgp);
}
/**
* @return Enable internet security.
*
*/
public Optional enableInternetSecurity() {
return Optional.ofNullable(this.enableInternetSecurity);
}
/**
* @return EnableBgp flag.
*
*/
public Optional enableRateLimiting() {
return Optional.ofNullable(this.enableRateLimiting);
}
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Resource ID.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return Ingress bytes transferred.
*
*/
public Double ingressBytesTransferred() {
return this.ingressBytesTransferred;
}
/**
* @return The IPSec Policies to be considered by this connection.
*
*/
public List ipsecPolicies() {
return this.ipsecPolicies == null ? List.of() : this.ipsecPolicies;
}
/**
* @return The name of the resource that is unique within a resource group. This name can be used to access the resource.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return The provisioning state of the VPN connection resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Id of the connected vpn site.
*
*/
public Optional remoteVpnSite() {
return Optional.ofNullable(this.remoteVpnSite);
}
/**
* @return The Routing Configuration indicating the associated and propagated route tables on this connection.
*
*/
public Optional routingConfiguration() {
return Optional.ofNullable(this.routingConfiguration);
}
/**
* @return Routing weight for vpn connection.
*
*/
public Optional routingWeight() {
return Optional.ofNullable(this.routingWeight);
}
/**
* @return SharedKey for the vpn connection.
*
*/
public Optional sharedKey() {
return Optional.ofNullable(this.sharedKey);
}
/**
* @return The Traffic Selector Policies to be considered by this connection.
*
*/
public List trafficSelectorPolicies() {
return this.trafficSelectorPolicies == null ? List.of() : this.trafficSelectorPolicies;
}
/**
* @return Use local azure ip to initiate connection.
*
*/
public Optional useLocalAzureIpAddress() {
return Optional.ofNullable(this.useLocalAzureIpAddress);
}
/**
* @return Enable policy-based traffic selectors.
*
*/
public Optional usePolicyBasedTrafficSelectors() {
return Optional.ofNullable(this.usePolicyBasedTrafficSelectors);
}
/**
* @return Connection protocol used for this connection.
*
*/
public Optional vpnConnectionProtocolType() {
return Optional.ofNullable(this.vpnConnectionProtocolType);
}
/**
* @return List of all vpn site link connections to the gateway.
*
*/
public List vpnLinkConnections() {
return this.vpnLinkConnections == null ? List.of() : this.vpnLinkConnections;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetVpnConnectionResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Integer connectionBandwidth;
private String connectionStatus;
private @Nullable Integer dpdTimeoutSeconds;
private Double egressBytesTransferred;
private @Nullable Boolean enableBgp;
private @Nullable Boolean enableInternetSecurity;
private @Nullable Boolean enableRateLimiting;
private String etag;
private @Nullable String id;
private Double ingressBytesTransferred;
private @Nullable List ipsecPolicies;
private @Nullable String name;
private String provisioningState;
private @Nullable SubResourceResponse remoteVpnSite;
private @Nullable RoutingConfigurationResponse routingConfiguration;
private @Nullable Integer routingWeight;
private @Nullable String sharedKey;
private @Nullable List trafficSelectorPolicies;
private @Nullable Boolean useLocalAzureIpAddress;
private @Nullable Boolean usePolicyBasedTrafficSelectors;
private @Nullable String vpnConnectionProtocolType;
private @Nullable List vpnLinkConnections;
public Builder() {}
public Builder(GetVpnConnectionResult defaults) {
Objects.requireNonNull(defaults);
this.connectionBandwidth = defaults.connectionBandwidth;
this.connectionStatus = defaults.connectionStatus;
this.dpdTimeoutSeconds = defaults.dpdTimeoutSeconds;
this.egressBytesTransferred = defaults.egressBytesTransferred;
this.enableBgp = defaults.enableBgp;
this.enableInternetSecurity = defaults.enableInternetSecurity;
this.enableRateLimiting = defaults.enableRateLimiting;
this.etag = defaults.etag;
this.id = defaults.id;
this.ingressBytesTransferred = defaults.ingressBytesTransferred;
this.ipsecPolicies = defaults.ipsecPolicies;
this.name = defaults.name;
this.provisioningState = defaults.provisioningState;
this.remoteVpnSite = defaults.remoteVpnSite;
this.routingConfiguration = defaults.routingConfiguration;
this.routingWeight = defaults.routingWeight;
this.sharedKey = defaults.sharedKey;
this.trafficSelectorPolicies = defaults.trafficSelectorPolicies;
this.useLocalAzureIpAddress = defaults.useLocalAzureIpAddress;
this.usePolicyBasedTrafficSelectors = defaults.usePolicyBasedTrafficSelectors;
this.vpnConnectionProtocolType = defaults.vpnConnectionProtocolType;
this.vpnLinkConnections = defaults.vpnLinkConnections;
}
@CustomType.Setter
public Builder connectionBandwidth(@Nullable Integer connectionBandwidth) {
this.connectionBandwidth = connectionBandwidth;
return this;
}
@CustomType.Setter
public Builder connectionStatus(String connectionStatus) {
if (connectionStatus == null) {
throw new MissingRequiredPropertyException("GetVpnConnectionResult", "connectionStatus");
}
this.connectionStatus = connectionStatus;
return this;
}
@CustomType.Setter
public Builder dpdTimeoutSeconds(@Nullable Integer dpdTimeoutSeconds) {
this.dpdTimeoutSeconds = dpdTimeoutSeconds;
return this;
}
@CustomType.Setter
public Builder egressBytesTransferred(Double egressBytesTransferred) {
if (egressBytesTransferred == null) {
throw new MissingRequiredPropertyException("GetVpnConnectionResult", "egressBytesTransferred");
}
this.egressBytesTransferred = egressBytesTransferred;
return this;
}
@CustomType.Setter
public Builder enableBgp(@Nullable Boolean enableBgp) {
this.enableBgp = enableBgp;
return this;
}
@CustomType.Setter
public Builder enableInternetSecurity(@Nullable Boolean enableInternetSecurity) {
this.enableInternetSecurity = enableInternetSecurity;
return this;
}
@CustomType.Setter
public Builder enableRateLimiting(@Nullable Boolean enableRateLimiting) {
this.enableRateLimiting = enableRateLimiting;
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("GetVpnConnectionResult", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder ingressBytesTransferred(Double ingressBytesTransferred) {
if (ingressBytesTransferred == null) {
throw new MissingRequiredPropertyException("GetVpnConnectionResult", "ingressBytesTransferred");
}
this.ingressBytesTransferred = ingressBytesTransferred;
return this;
}
@CustomType.Setter
public Builder ipsecPolicies(@Nullable List ipsecPolicies) {
this.ipsecPolicies = ipsecPolicies;
return this;
}
public Builder ipsecPolicies(IpsecPolicyResponse... ipsecPolicies) {
return ipsecPolicies(List.of(ipsecPolicies));
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetVpnConnectionResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder remoteVpnSite(@Nullable SubResourceResponse remoteVpnSite) {
this.remoteVpnSite = remoteVpnSite;
return this;
}
@CustomType.Setter
public Builder routingConfiguration(@Nullable RoutingConfigurationResponse routingConfiguration) {
this.routingConfiguration = routingConfiguration;
return this;
}
@CustomType.Setter
public Builder routingWeight(@Nullable Integer routingWeight) {
this.routingWeight = routingWeight;
return this;
}
@CustomType.Setter
public Builder sharedKey(@Nullable String sharedKey) {
this.sharedKey = sharedKey;
return this;
}
@CustomType.Setter
public Builder trafficSelectorPolicies(@Nullable List trafficSelectorPolicies) {
this.trafficSelectorPolicies = trafficSelectorPolicies;
return this;
}
public Builder trafficSelectorPolicies(TrafficSelectorPolicyResponse... trafficSelectorPolicies) {
return trafficSelectorPolicies(List.of(trafficSelectorPolicies));
}
@CustomType.Setter
public Builder useLocalAzureIpAddress(@Nullable Boolean useLocalAzureIpAddress) {
this.useLocalAzureIpAddress = useLocalAzureIpAddress;
return this;
}
@CustomType.Setter
public Builder usePolicyBasedTrafficSelectors(@Nullable Boolean usePolicyBasedTrafficSelectors) {
this.usePolicyBasedTrafficSelectors = usePolicyBasedTrafficSelectors;
return this;
}
@CustomType.Setter
public Builder vpnConnectionProtocolType(@Nullable String vpnConnectionProtocolType) {
this.vpnConnectionProtocolType = vpnConnectionProtocolType;
return this;
}
@CustomType.Setter
public Builder vpnLinkConnections(@Nullable List vpnLinkConnections) {
this.vpnLinkConnections = vpnLinkConnections;
return this;
}
public Builder vpnLinkConnections(VpnSiteLinkConnectionResponse... vpnLinkConnections) {
return vpnLinkConnections(List.of(vpnLinkConnections));
}
public GetVpnConnectionResult build() {
final var _resultValue = new GetVpnConnectionResult();
_resultValue.connectionBandwidth = connectionBandwidth;
_resultValue.connectionStatus = connectionStatus;
_resultValue.dpdTimeoutSeconds = dpdTimeoutSeconds;
_resultValue.egressBytesTransferred = egressBytesTransferred;
_resultValue.enableBgp = enableBgp;
_resultValue.enableInternetSecurity = enableInternetSecurity;
_resultValue.enableRateLimiting = enableRateLimiting;
_resultValue.etag = etag;
_resultValue.id = id;
_resultValue.ingressBytesTransferred = ingressBytesTransferred;
_resultValue.ipsecPolicies = ipsecPolicies;
_resultValue.name = name;
_resultValue.provisioningState = provisioningState;
_resultValue.remoteVpnSite = remoteVpnSite;
_resultValue.routingConfiguration = routingConfiguration;
_resultValue.routingWeight = routingWeight;
_resultValue.sharedKey = sharedKey;
_resultValue.trafficSelectorPolicies = trafficSelectorPolicies;
_resultValue.useLocalAzureIpAddress = useLocalAzureIpAddress;
_resultValue.usePolicyBasedTrafficSelectors = usePolicyBasedTrafficSelectors;
_resultValue.vpnConnectionProtocolType = vpnConnectionProtocolType;
_resultValue.vpnLinkConnections = vpnLinkConnections;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy