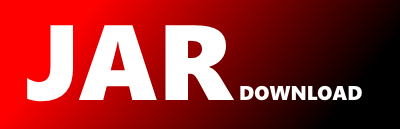
com.pulumi.azurenative.network.outputs.SingleQueryResultResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class SingleQueryResultResponse {
/**
* @return Describes what is the signature enforces
*
*/
private @Nullable String description;
/**
* @return Describes the list of destination ports related to this signature
*
*/
private @Nullable List destinationPorts;
/**
* @return Describes in which direction signature is being enforced: 0 - Inbound, 1 - OutBound, 2 - Bidirectional
*
*/
private @Nullable Integer direction;
/**
* @return Describes the groups the signature belongs to
*
*/
private @Nullable String group;
/**
* @return Describes if this override is inherited from base policy or not
*
*/
private @Nullable Boolean inheritedFromParentPolicy;
/**
* @return Describes the last updated time of the signature (provided from 3rd party vendor)
*
*/
private @Nullable String lastUpdated;
/**
* @return The current mode enforced, 0 - Disabled, 1 - Alert, 2 -Deny
*
*/
private @Nullable Integer mode;
/**
* @return Describes the protocol the signatures is being enforced in
*
*/
private @Nullable String protocol;
/**
* @return Describes the severity of signature: 1 - Low, 2 - Medium, 3 - High
*
*/
private @Nullable Integer severity;
/**
* @return The ID of the signature
*
*/
private @Nullable Integer signatureId;
/**
* @return Describes the list of source ports related to this signature
*
*/
private @Nullable List sourcePorts;
private SingleQueryResultResponse() {}
/**
* @return Describes what is the signature enforces
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return Describes the list of destination ports related to this signature
*
*/
public List destinationPorts() {
return this.destinationPorts == null ? List.of() : this.destinationPorts;
}
/**
* @return Describes in which direction signature is being enforced: 0 - Inbound, 1 - OutBound, 2 - Bidirectional
*
*/
public Optional direction() {
return Optional.ofNullable(this.direction);
}
/**
* @return Describes the groups the signature belongs to
*
*/
public Optional group() {
return Optional.ofNullable(this.group);
}
/**
* @return Describes if this override is inherited from base policy or not
*
*/
public Optional inheritedFromParentPolicy() {
return Optional.ofNullable(this.inheritedFromParentPolicy);
}
/**
* @return Describes the last updated time of the signature (provided from 3rd party vendor)
*
*/
public Optional lastUpdated() {
return Optional.ofNullable(this.lastUpdated);
}
/**
* @return The current mode enforced, 0 - Disabled, 1 - Alert, 2 -Deny
*
*/
public Optional mode() {
return Optional.ofNullable(this.mode);
}
/**
* @return Describes the protocol the signatures is being enforced in
*
*/
public Optional protocol() {
return Optional.ofNullable(this.protocol);
}
/**
* @return Describes the severity of signature: 1 - Low, 2 - Medium, 3 - High
*
*/
public Optional severity() {
return Optional.ofNullable(this.severity);
}
/**
* @return The ID of the signature
*
*/
public Optional signatureId() {
return Optional.ofNullable(this.signatureId);
}
/**
* @return Describes the list of source ports related to this signature
*
*/
public List sourcePorts() {
return this.sourcePorts == null ? List.of() : this.sourcePorts;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(SingleQueryResultResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String description;
private @Nullable List destinationPorts;
private @Nullable Integer direction;
private @Nullable String group;
private @Nullable Boolean inheritedFromParentPolicy;
private @Nullable String lastUpdated;
private @Nullable Integer mode;
private @Nullable String protocol;
private @Nullable Integer severity;
private @Nullable Integer signatureId;
private @Nullable List sourcePorts;
public Builder() {}
public Builder(SingleQueryResultResponse defaults) {
Objects.requireNonNull(defaults);
this.description = defaults.description;
this.destinationPorts = defaults.destinationPorts;
this.direction = defaults.direction;
this.group = defaults.group;
this.inheritedFromParentPolicy = defaults.inheritedFromParentPolicy;
this.lastUpdated = defaults.lastUpdated;
this.mode = defaults.mode;
this.protocol = defaults.protocol;
this.severity = defaults.severity;
this.signatureId = defaults.signatureId;
this.sourcePorts = defaults.sourcePorts;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder destinationPorts(@Nullable List destinationPorts) {
this.destinationPorts = destinationPorts;
return this;
}
public Builder destinationPorts(String... destinationPorts) {
return destinationPorts(List.of(destinationPorts));
}
@CustomType.Setter
public Builder direction(@Nullable Integer direction) {
this.direction = direction;
return this;
}
@CustomType.Setter
public Builder group(@Nullable String group) {
this.group = group;
return this;
}
@CustomType.Setter
public Builder inheritedFromParentPolicy(@Nullable Boolean inheritedFromParentPolicy) {
this.inheritedFromParentPolicy = inheritedFromParentPolicy;
return this;
}
@CustomType.Setter
public Builder lastUpdated(@Nullable String lastUpdated) {
this.lastUpdated = lastUpdated;
return this;
}
@CustomType.Setter
public Builder mode(@Nullable Integer mode) {
this.mode = mode;
return this;
}
@CustomType.Setter
public Builder protocol(@Nullable String protocol) {
this.protocol = protocol;
return this;
}
@CustomType.Setter
public Builder severity(@Nullable Integer severity) {
this.severity = severity;
return this;
}
@CustomType.Setter
public Builder signatureId(@Nullable Integer signatureId) {
this.signatureId = signatureId;
return this;
}
@CustomType.Setter
public Builder sourcePorts(@Nullable List sourcePorts) {
this.sourcePorts = sourcePorts;
return this;
}
public Builder sourcePorts(String... sourcePorts) {
return sourcePorts(List.of(sourcePorts));
}
public SingleQueryResultResponse build() {
final var _resultValue = new SingleQueryResultResponse();
_resultValue.description = description;
_resultValue.destinationPorts = destinationPorts;
_resultValue.direction = direction;
_resultValue.group = group;
_resultValue.inheritedFromParentPolicy = inheritedFromParentPolicy;
_resultValue.lastUpdated = lastUpdated;
_resultValue.mode = mode;
_resultValue.protocol = protocol;
_resultValue.severity = severity;
_resultValue.signatureId = signatureId;
_resultValue.sourcePorts = sourcePorts;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy