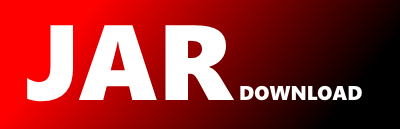
com.pulumi.azurenative.networkanalytics.outputs.GetDataProductResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.networkanalytics.outputs;
import com.pulumi.azurenative.networkanalytics.outputs.ConsumptionEndpointsPropertiesResponse;
import com.pulumi.azurenative.networkanalytics.outputs.DataProductNetworkAclsResponse;
import com.pulumi.azurenative.networkanalytics.outputs.EncryptionKeyDetailsResponse;
import com.pulumi.azurenative.networkanalytics.outputs.ManagedServiceIdentityResponse;
import com.pulumi.azurenative.networkanalytics.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetDataProductResult {
/**
* @return List of available minor versions of the data product resource.
*
*/
private List availableMinorVersions;
/**
* @return Resource links which exposed to the customer to query the data.
*
*/
private ConsumptionEndpointsPropertiesResponse consumptionEndpoints;
/**
* @return Current configured minor version of the data product resource.
*
*/
private @Nullable String currentMinorVersion;
/**
* @return Customer managed encryption key details for data product.
*
*/
private @Nullable EncryptionKeyDetailsResponse customerEncryptionKey;
/**
* @return Flag to enable customer managed key encryption for data product.
*
*/
private @Nullable String customerManagedKeyEncryptionEnabled;
/**
* @return Documentation link for the data product based on definition file.
*
*/
private String documentation;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The managed service identities assigned to this resource.
*
*/
private @Nullable ManagedServiceIdentityResponse identity;
/**
* @return Key vault url.
*
*/
private String keyVaultUrl;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return Major version of data product.
*
*/
private String majorVersion;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Network rule set for data product.
*
*/
private @Nullable DataProductNetworkAclsResponse networkacls;
/**
* @return List of name or email associated with data product resource deployment.
*
*/
private @Nullable List owners;
/**
* @return Flag to enable or disable private link for data product resource.
*
*/
private @Nullable String privateLinksEnabled;
/**
* @return Product name of data product.
*
*/
private String product;
/**
* @return Latest provisioning state of data product.
*
*/
private String provisioningState;
/**
* @return Flag to enable or disable public access of data product resource.
*
*/
private @Nullable String publicNetworkAccess;
/**
* @return Data product publisher name.
*
*/
private String publisher;
/**
* @return Purview account url for data product to connect to.
*
*/
private @Nullable String purviewAccount;
/**
* @return Purview collection url for data product to connect to.
*
*/
private @Nullable String purviewCollection;
/**
* @return Flag to enable or disable redundancy for data product.
*
*/
private @Nullable String redundancy;
/**
* @return The resource GUID property of the data product resource.
*
*/
private String resourceGuid;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetDataProductResult() {}
/**
* @return List of available minor versions of the data product resource.
*
*/
public List availableMinorVersions() {
return this.availableMinorVersions;
}
/**
* @return Resource links which exposed to the customer to query the data.
*
*/
public ConsumptionEndpointsPropertiesResponse consumptionEndpoints() {
return this.consumptionEndpoints;
}
/**
* @return Current configured minor version of the data product resource.
*
*/
public Optional currentMinorVersion() {
return Optional.ofNullable(this.currentMinorVersion);
}
/**
* @return Customer managed encryption key details for data product.
*
*/
public Optional customerEncryptionKey() {
return Optional.ofNullable(this.customerEncryptionKey);
}
/**
* @return Flag to enable customer managed key encryption for data product.
*
*/
public Optional customerManagedKeyEncryptionEnabled() {
return Optional.ofNullable(this.customerManagedKeyEncryptionEnabled);
}
/**
* @return Documentation link for the data product based on definition file.
*
*/
public String documentation() {
return this.documentation;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The managed service identities assigned to this resource.
*
*/
public Optional identity() {
return Optional.ofNullable(this.identity);
}
/**
* @return Key vault url.
*
*/
public String keyVaultUrl() {
return this.keyVaultUrl;
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return Major version of data product.
*
*/
public String majorVersion() {
return this.majorVersion;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Network rule set for data product.
*
*/
public Optional networkacls() {
return Optional.ofNullable(this.networkacls);
}
/**
* @return List of name or email associated with data product resource deployment.
*
*/
public List owners() {
return this.owners == null ? List.of() : this.owners;
}
/**
* @return Flag to enable or disable private link for data product resource.
*
*/
public Optional privateLinksEnabled() {
return Optional.ofNullable(this.privateLinksEnabled);
}
/**
* @return Product name of data product.
*
*/
public String product() {
return this.product;
}
/**
* @return Latest provisioning state of data product.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Flag to enable or disable public access of data product resource.
*
*/
public Optional publicNetworkAccess() {
return Optional.ofNullable(this.publicNetworkAccess);
}
/**
* @return Data product publisher name.
*
*/
public String publisher() {
return this.publisher;
}
/**
* @return Purview account url for data product to connect to.
*
*/
public Optional purviewAccount() {
return Optional.ofNullable(this.purviewAccount);
}
/**
* @return Purview collection url for data product to connect to.
*
*/
public Optional purviewCollection() {
return Optional.ofNullable(this.purviewCollection);
}
/**
* @return Flag to enable or disable redundancy for data product.
*
*/
public Optional redundancy() {
return Optional.ofNullable(this.redundancy);
}
/**
* @return The resource GUID property of the data product resource.
*
*/
public String resourceGuid() {
return this.resourceGuid;
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetDataProductResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List availableMinorVersions;
private ConsumptionEndpointsPropertiesResponse consumptionEndpoints;
private @Nullable String currentMinorVersion;
private @Nullable EncryptionKeyDetailsResponse customerEncryptionKey;
private @Nullable String customerManagedKeyEncryptionEnabled;
private String documentation;
private String id;
private @Nullable ManagedServiceIdentityResponse identity;
private String keyVaultUrl;
private String location;
private String majorVersion;
private String name;
private @Nullable DataProductNetworkAclsResponse networkacls;
private @Nullable List owners;
private @Nullable String privateLinksEnabled;
private String product;
private String provisioningState;
private @Nullable String publicNetworkAccess;
private String publisher;
private @Nullable String purviewAccount;
private @Nullable String purviewCollection;
private @Nullable String redundancy;
private String resourceGuid;
private SystemDataResponse systemData;
private @Nullable Map tags;
private String type;
public Builder() {}
public Builder(GetDataProductResult defaults) {
Objects.requireNonNull(defaults);
this.availableMinorVersions = defaults.availableMinorVersions;
this.consumptionEndpoints = defaults.consumptionEndpoints;
this.currentMinorVersion = defaults.currentMinorVersion;
this.customerEncryptionKey = defaults.customerEncryptionKey;
this.customerManagedKeyEncryptionEnabled = defaults.customerManagedKeyEncryptionEnabled;
this.documentation = defaults.documentation;
this.id = defaults.id;
this.identity = defaults.identity;
this.keyVaultUrl = defaults.keyVaultUrl;
this.location = defaults.location;
this.majorVersion = defaults.majorVersion;
this.name = defaults.name;
this.networkacls = defaults.networkacls;
this.owners = defaults.owners;
this.privateLinksEnabled = defaults.privateLinksEnabled;
this.product = defaults.product;
this.provisioningState = defaults.provisioningState;
this.publicNetworkAccess = defaults.publicNetworkAccess;
this.publisher = defaults.publisher;
this.purviewAccount = defaults.purviewAccount;
this.purviewCollection = defaults.purviewCollection;
this.redundancy = defaults.redundancy;
this.resourceGuid = defaults.resourceGuid;
this.systemData = defaults.systemData;
this.tags = defaults.tags;
this.type = defaults.type;
}
@CustomType.Setter
public Builder availableMinorVersions(List availableMinorVersions) {
if (availableMinorVersions == null) {
throw new MissingRequiredPropertyException("GetDataProductResult", "availableMinorVersions");
}
this.availableMinorVersions = availableMinorVersions;
return this;
}
public Builder availableMinorVersions(String... availableMinorVersions) {
return availableMinorVersions(List.of(availableMinorVersions));
}
@CustomType.Setter
public Builder consumptionEndpoints(ConsumptionEndpointsPropertiesResponse consumptionEndpoints) {
if (consumptionEndpoints == null) {
throw new MissingRequiredPropertyException("GetDataProductResult", "consumptionEndpoints");
}
this.consumptionEndpoints = consumptionEndpoints;
return this;
}
@CustomType.Setter
public Builder currentMinorVersion(@Nullable String currentMinorVersion) {
this.currentMinorVersion = currentMinorVersion;
return this;
}
@CustomType.Setter
public Builder customerEncryptionKey(@Nullable EncryptionKeyDetailsResponse customerEncryptionKey) {
this.customerEncryptionKey = customerEncryptionKey;
return this;
}
@CustomType.Setter
public Builder customerManagedKeyEncryptionEnabled(@Nullable String customerManagedKeyEncryptionEnabled) {
this.customerManagedKeyEncryptionEnabled = customerManagedKeyEncryptionEnabled;
return this;
}
@CustomType.Setter
public Builder documentation(String documentation) {
if (documentation == null) {
throw new MissingRequiredPropertyException("GetDataProductResult", "documentation");
}
this.documentation = documentation;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetDataProductResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder identity(@Nullable ManagedServiceIdentityResponse identity) {
this.identity = identity;
return this;
}
@CustomType.Setter
public Builder keyVaultUrl(String keyVaultUrl) {
if (keyVaultUrl == null) {
throw new MissingRequiredPropertyException("GetDataProductResult", "keyVaultUrl");
}
this.keyVaultUrl = keyVaultUrl;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetDataProductResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder majorVersion(String majorVersion) {
if (majorVersion == null) {
throw new MissingRequiredPropertyException("GetDataProductResult", "majorVersion");
}
this.majorVersion = majorVersion;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetDataProductResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder networkacls(@Nullable DataProductNetworkAclsResponse networkacls) {
this.networkacls = networkacls;
return this;
}
@CustomType.Setter
public Builder owners(@Nullable List owners) {
this.owners = owners;
return this;
}
public Builder owners(String... owners) {
return owners(List.of(owners));
}
@CustomType.Setter
public Builder privateLinksEnabled(@Nullable String privateLinksEnabled) {
this.privateLinksEnabled = privateLinksEnabled;
return this;
}
@CustomType.Setter
public Builder product(String product) {
if (product == null) {
throw new MissingRequiredPropertyException("GetDataProductResult", "product");
}
this.product = product;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetDataProductResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder publicNetworkAccess(@Nullable String publicNetworkAccess) {
this.publicNetworkAccess = publicNetworkAccess;
return this;
}
@CustomType.Setter
public Builder publisher(String publisher) {
if (publisher == null) {
throw new MissingRequiredPropertyException("GetDataProductResult", "publisher");
}
this.publisher = publisher;
return this;
}
@CustomType.Setter
public Builder purviewAccount(@Nullable String purviewAccount) {
this.purviewAccount = purviewAccount;
return this;
}
@CustomType.Setter
public Builder purviewCollection(@Nullable String purviewCollection) {
this.purviewCollection = purviewCollection;
return this;
}
@CustomType.Setter
public Builder redundancy(@Nullable String redundancy) {
this.redundancy = redundancy;
return this;
}
@CustomType.Setter
public Builder resourceGuid(String resourceGuid) {
if (resourceGuid == null) {
throw new MissingRequiredPropertyException("GetDataProductResult", "resourceGuid");
}
this.resourceGuid = resourceGuid;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetDataProductResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetDataProductResult", "type");
}
this.type = type;
return this;
}
public GetDataProductResult build() {
final var _resultValue = new GetDataProductResult();
_resultValue.availableMinorVersions = availableMinorVersions;
_resultValue.consumptionEndpoints = consumptionEndpoints;
_resultValue.currentMinorVersion = currentMinorVersion;
_resultValue.customerEncryptionKey = customerEncryptionKey;
_resultValue.customerManagedKeyEncryptionEnabled = customerManagedKeyEncryptionEnabled;
_resultValue.documentation = documentation;
_resultValue.id = id;
_resultValue.identity = identity;
_resultValue.keyVaultUrl = keyVaultUrl;
_resultValue.location = location;
_resultValue.majorVersion = majorVersion;
_resultValue.name = name;
_resultValue.networkacls = networkacls;
_resultValue.owners = owners;
_resultValue.privateLinksEnabled = privateLinksEnabled;
_resultValue.product = product;
_resultValue.provisioningState = provisioningState;
_resultValue.publicNetworkAccess = publicNetworkAccess;
_resultValue.publisher = publisher;
_resultValue.purviewAccount = purviewAccount;
_resultValue.purviewCollection = purviewCollection;
_resultValue.redundancy = redundancy;
_resultValue.resourceGuid = resourceGuid;
_resultValue.systemData = systemData;
_resultValue.tags = tags;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy