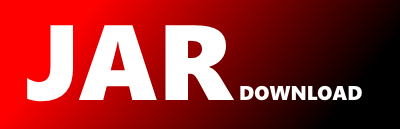
com.pulumi.azurenative.networkcloud.ClusterArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.networkcloud;
import com.pulumi.azurenative.networkcloud.enums.ClusterType;
import com.pulumi.azurenative.networkcloud.inputs.ClusterSecretArchiveArgs;
import com.pulumi.azurenative.networkcloud.inputs.ClusterUpdateStrategyArgs;
import com.pulumi.azurenative.networkcloud.inputs.ExtendedLocationArgs;
import com.pulumi.azurenative.networkcloud.inputs.ManagedResourceGroupConfigurationArgs;
import com.pulumi.azurenative.networkcloud.inputs.RackDefinitionArgs;
import com.pulumi.azurenative.networkcloud.inputs.RuntimeProtectionConfigurationArgs;
import com.pulumi.azurenative.networkcloud.inputs.ServicePrincipalInformationArgs;
import com.pulumi.azurenative.networkcloud.inputs.ValidationThresholdArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ClusterArgs extends com.pulumi.resources.ResourceArgs {
public static final ClusterArgs Empty = new ClusterArgs();
/**
* The rack definition that is intended to reflect only a single rack in a single rack cluster, or an aggregator rack in a multi-rack cluster.
*
*/
@Import(name="aggregatorOrSingleRackDefinition", required=true)
private Output aggregatorOrSingleRackDefinition;
/**
* @return The rack definition that is intended to reflect only a single rack in a single rack cluster, or an aggregator rack in a multi-rack cluster.
*
*/
public Output aggregatorOrSingleRackDefinition() {
return this.aggregatorOrSingleRackDefinition;
}
/**
* The resource ID of the Log Analytics Workspace that will be used for storing relevant logs.
*
*/
@Import(name="analyticsWorkspaceId")
private @Nullable Output analyticsWorkspaceId;
/**
* @return The resource ID of the Log Analytics Workspace that will be used for storing relevant logs.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy