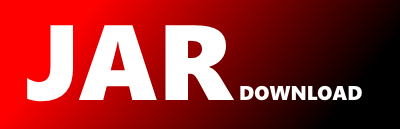
com.pulumi.azurenative.networkcloud.KubernetesClusterArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.networkcloud;
import com.pulumi.azurenative.networkcloud.inputs.AadConfigurationArgs;
import com.pulumi.azurenative.networkcloud.inputs.AdministratorConfigurationArgs;
import com.pulumi.azurenative.networkcloud.inputs.ControlPlaneNodeConfigurationArgs;
import com.pulumi.azurenative.networkcloud.inputs.ExtendedLocationArgs;
import com.pulumi.azurenative.networkcloud.inputs.InitialAgentPoolConfigurationArgs;
import com.pulumi.azurenative.networkcloud.inputs.ManagedResourceGroupConfigurationArgs;
import com.pulumi.azurenative.networkcloud.inputs.NetworkConfigurationArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class KubernetesClusterArgs extends com.pulumi.resources.ResourceArgs {
public static final KubernetesClusterArgs Empty = new KubernetesClusterArgs();
/**
* The Azure Active Directory Integration properties.
*
*/
@Import(name="aadConfiguration")
private @Nullable Output aadConfiguration;
/**
* @return The Azure Active Directory Integration properties.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy