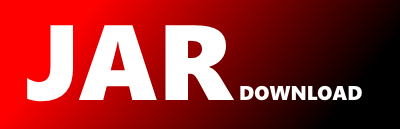
com.pulumi.azurenative.networkcloud.inputs.BgpAdvertisementArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.networkcloud.inputs;
import com.pulumi.azurenative.networkcloud.enums.AdvertiseToFabric;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class BgpAdvertisementArgs extends com.pulumi.resources.ResourceArgs {
public static final BgpAdvertisementArgs Empty = new BgpAdvertisementArgs();
/**
* The indicator of if this advertisement is also made to the network fabric associated with the Network Cloud Cluster. This field is ignored if fabricPeeringEnabled is set to False.
*
*/
@Import(name="advertiseToFabric")
private @Nullable Output> advertiseToFabric;
/**
* @return The indicator of if this advertisement is also made to the network fabric associated with the Network Cloud Cluster. This field is ignored if fabricPeeringEnabled is set to False.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy