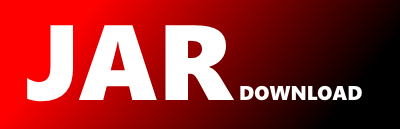
com.pulumi.azurenative.networkcloud.inputs.IpAddressPoolArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.networkcloud.inputs;
import com.pulumi.azurenative.networkcloud.enums.BfdEnabled;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class IpAddressPoolArgs extends com.pulumi.resources.ResourceArgs {
public static final IpAddressPoolArgs Empty = new IpAddressPoolArgs();
/**
* The list of IP address ranges. Each range can be a either a subnet in CIDR format or an explicit start-end range of IP addresses.
*
*/
@Import(name="addresses", required=true)
private Output> addresses;
/**
* @return The list of IP address ranges. Each range can be a either a subnet in CIDR format or an explicit start-end range of IP addresses.
*
*/
public Output> addresses() {
return this.addresses;
}
/**
* The indicator to determine if automatic allocation from the pool should occur.
*
*/
@Import(name="autoAssign")
private @Nullable Output> autoAssign;
/**
* @return The indicator to determine if automatic allocation from the pool should occur.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy