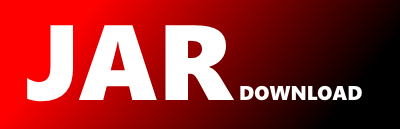
com.pulumi.azurenative.networkcloud.inputs.NetworkConfigurationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.networkcloud.inputs;
import com.pulumi.azurenative.networkcloud.inputs.AttachedNetworkConfigurationArgs;
import com.pulumi.azurenative.networkcloud.inputs.BgpServiceLoadBalancerConfigurationArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class NetworkConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final NetworkConfigurationArgs Empty = new NetworkConfigurationArgs();
/**
* The configuration of networks being attached to the cluster for use by the workloads that run on this Kubernetes cluster.
*
*/
@Import(name="attachedNetworkConfiguration")
private @Nullable Output attachedNetworkConfiguration;
/**
* @return The configuration of networks being attached to the cluster for use by the workloads that run on this Kubernetes cluster.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy