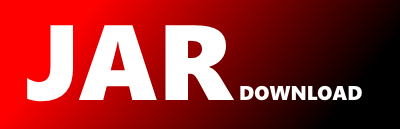
com.pulumi.azurenative.networkcloud.outputs.GetBareMetalMachineResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.networkcloud.outputs;
import com.pulumi.azurenative.networkcloud.outputs.AdministrativeCredentialsResponse;
import com.pulumi.azurenative.networkcloud.outputs.ExtendedLocationResponse;
import com.pulumi.azurenative.networkcloud.outputs.HardwareInventoryResponse;
import com.pulumi.azurenative.networkcloud.outputs.HardwareValidationStatusResponse;
import com.pulumi.azurenative.networkcloud.outputs.RuntimeProtectionStatusResponse;
import com.pulumi.azurenative.networkcloud.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import javax.annotation.Nullable;
@CustomType
public final class GetBareMetalMachineResult {
/**
* @return The list of resource IDs for the other Microsoft.NetworkCloud resources that have attached this network.
*
*/
private List associatedResourceIds;
/**
* @return The connection string for the baseboard management controller including IP address and protocol.
*
*/
private String bmcConnectionString;
/**
* @return The credentials of the baseboard management controller on this bare metal machine.
*
*/
private AdministrativeCredentialsResponse bmcCredentials;
/**
* @return The MAC address of the BMC device.
*
*/
private String bmcMacAddress;
/**
* @return The MAC address of a NIC connected to the PXE network.
*
*/
private String bootMacAddress;
/**
* @return The resource ID of the cluster this bare metal machine is associated with.
*
*/
private String clusterId;
/**
* @return The cordon status of the bare metal machine.
*
*/
private String cordonStatus;
/**
* @return The more detailed status of the bare metal machine.
*
*/
private String detailedStatus;
/**
* @return The descriptive message about the current detailed status.
*
*/
private String detailedStatusMessage;
/**
* @return The extended location of the cluster associated with the resource.
*
*/
private ExtendedLocationResponse extendedLocation;
/**
* @return The hardware inventory, including information acquired from the model/sku information and from the ironic inspector.
*
*/
private HardwareInventoryResponse hardwareInventory;
/**
* @return The details of the latest hardware validation performed for this bare metal machine.
*
*/
private HardwareValidationStatusResponse hardwareValidationStatus;
/**
* @return Field Deprecated. These fields will be empty/omitted. The list of the resource IDs for the HybridAksClusters that have nodes hosted on this bare metal machine.
*
*/
private List hybridAksClustersAssociatedIds;
/**
* @return Fully qualified resource ID for the resource. E.g. "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}"
*
*/
private String id;
/**
* @return The name of this machine represented by the host object in the Cluster's Kubernetes control plane.
*
*/
private String kubernetesNodeName;
/**
* @return The version of Kubernetes running on this machine.
*
*/
private String kubernetesVersion;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return The custom details provided by the customer.
*
*/
private String machineDetails;
/**
* @return The OS-level hostname assigned to this machine.
*
*/
private String machineName;
/**
* @return The list of roles that are assigned to the cluster node running on this machine.
*
*/
private List machineRoles;
/**
* @return The unique internal identifier of the bare metal machine SKU.
*
*/
private String machineSkuId;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return The IPv4 address that is assigned to the bare metal machine during the cluster deployment.
*
*/
private String oamIpv4Address;
/**
* @return The IPv6 address that is assigned to the bare metal machine during the cluster deployment.
*
*/
private String oamIpv6Address;
/**
* @return The image that is currently provisioned to the OS disk.
*
*/
private String osImage;
/**
* @return The power state derived from the baseboard management controller.
*
*/
private String powerState;
/**
* @return The provisioning state of the bare metal machine.
*
*/
private String provisioningState;
/**
* @return The resource ID of the rack where this bare metal machine resides.
*
*/
private String rackId;
/**
* @return The rack slot in which this bare metal machine is located, ordered from the bottom up i.e. the lowest slot is 1.
*
*/
private Double rackSlot;
/**
* @return The indicator of whether the bare metal machine is ready to receive workloads.
*
*/
private String readyState;
/**
* @return The runtime protection status of the bare metal machine.
*
*/
private RuntimeProtectionStatusResponse runtimeProtectionStatus;
/**
* @return The serial number of the bare metal machine.
*
*/
private String serialNumber;
/**
* @return The discovered value of the machine's service tag.
*
*/
private String serviceTag;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
/**
* @return Field Deprecated. These fields will be empty/omitted. The list of the resource IDs for the VirtualMachines that are hosted on this bare metal machine.
*
*/
private List virtualMachinesAssociatedIds;
private GetBareMetalMachineResult() {}
/**
* @return The list of resource IDs for the other Microsoft.NetworkCloud resources that have attached this network.
*
*/
public List associatedResourceIds() {
return this.associatedResourceIds;
}
/**
* @return The connection string for the baseboard management controller including IP address and protocol.
*
*/
public String bmcConnectionString() {
return this.bmcConnectionString;
}
/**
* @return The credentials of the baseboard management controller on this bare metal machine.
*
*/
public AdministrativeCredentialsResponse bmcCredentials() {
return this.bmcCredentials;
}
/**
* @return The MAC address of the BMC device.
*
*/
public String bmcMacAddress() {
return this.bmcMacAddress;
}
/**
* @return The MAC address of a NIC connected to the PXE network.
*
*/
public String bootMacAddress() {
return this.bootMacAddress;
}
/**
* @return The resource ID of the cluster this bare metal machine is associated with.
*
*/
public String clusterId() {
return this.clusterId;
}
/**
* @return The cordon status of the bare metal machine.
*
*/
public String cordonStatus() {
return this.cordonStatus;
}
/**
* @return The more detailed status of the bare metal machine.
*
*/
public String detailedStatus() {
return this.detailedStatus;
}
/**
* @return The descriptive message about the current detailed status.
*
*/
public String detailedStatusMessage() {
return this.detailedStatusMessage;
}
/**
* @return The extended location of the cluster associated with the resource.
*
*/
public ExtendedLocationResponse extendedLocation() {
return this.extendedLocation;
}
/**
* @return The hardware inventory, including information acquired from the model/sku information and from the ironic inspector.
*
*/
public HardwareInventoryResponse hardwareInventory() {
return this.hardwareInventory;
}
/**
* @return The details of the latest hardware validation performed for this bare metal machine.
*
*/
public HardwareValidationStatusResponse hardwareValidationStatus() {
return this.hardwareValidationStatus;
}
/**
* @return Field Deprecated. These fields will be empty/omitted. The list of the resource IDs for the HybridAksClusters that have nodes hosted on this bare metal machine.
*
*/
public List hybridAksClustersAssociatedIds() {
return this.hybridAksClustersAssociatedIds;
}
/**
* @return Fully qualified resource ID for the resource. E.g. "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}"
*
*/
public String id() {
return this.id;
}
/**
* @return The name of this machine represented by the host object in the Cluster's Kubernetes control plane.
*
*/
public String kubernetesNodeName() {
return this.kubernetesNodeName;
}
/**
* @return The version of Kubernetes running on this machine.
*
*/
public String kubernetesVersion() {
return this.kubernetesVersion;
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return The custom details provided by the customer.
*
*/
public String machineDetails() {
return this.machineDetails;
}
/**
* @return The OS-level hostname assigned to this machine.
*
*/
public String machineName() {
return this.machineName;
}
/**
* @return The list of roles that are assigned to the cluster node running on this machine.
*
*/
public List machineRoles() {
return this.machineRoles;
}
/**
* @return The unique internal identifier of the bare metal machine SKU.
*
*/
public String machineSkuId() {
return this.machineSkuId;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return The IPv4 address that is assigned to the bare metal machine during the cluster deployment.
*
*/
public String oamIpv4Address() {
return this.oamIpv4Address;
}
/**
* @return The IPv6 address that is assigned to the bare metal machine during the cluster deployment.
*
*/
public String oamIpv6Address() {
return this.oamIpv6Address;
}
/**
* @return The image that is currently provisioned to the OS disk.
*
*/
public String osImage() {
return this.osImage;
}
/**
* @return The power state derived from the baseboard management controller.
*
*/
public String powerState() {
return this.powerState;
}
/**
* @return The provisioning state of the bare metal machine.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The resource ID of the rack where this bare metal machine resides.
*
*/
public String rackId() {
return this.rackId;
}
/**
* @return The rack slot in which this bare metal machine is located, ordered from the bottom up i.e. the lowest slot is 1.
*
*/
public Double rackSlot() {
return this.rackSlot;
}
/**
* @return The indicator of whether the bare metal machine is ready to receive workloads.
*
*/
public String readyState() {
return this.readyState;
}
/**
* @return The runtime protection status of the bare metal machine.
*
*/
public RuntimeProtectionStatusResponse runtimeProtectionStatus() {
return this.runtimeProtectionStatus;
}
/**
* @return The serial number of the bare metal machine.
*
*/
public String serialNumber() {
return this.serialNumber;
}
/**
* @return The discovered value of the machine's service tag.
*
*/
public String serviceTag() {
return this.serviceTag;
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
/**
* @return Field Deprecated. These fields will be empty/omitted. The list of the resource IDs for the VirtualMachines that are hosted on this bare metal machine.
*
*/
public List virtualMachinesAssociatedIds() {
return this.virtualMachinesAssociatedIds;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetBareMetalMachineResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List associatedResourceIds;
private String bmcConnectionString;
private AdministrativeCredentialsResponse bmcCredentials;
private String bmcMacAddress;
private String bootMacAddress;
private String clusterId;
private String cordonStatus;
private String detailedStatus;
private String detailedStatusMessage;
private ExtendedLocationResponse extendedLocation;
private HardwareInventoryResponse hardwareInventory;
private HardwareValidationStatusResponse hardwareValidationStatus;
private List hybridAksClustersAssociatedIds;
private String id;
private String kubernetesNodeName;
private String kubernetesVersion;
private String location;
private String machineDetails;
private String machineName;
private List machineRoles;
private String machineSkuId;
private String name;
private String oamIpv4Address;
private String oamIpv6Address;
private String osImage;
private String powerState;
private String provisioningState;
private String rackId;
private Double rackSlot;
private String readyState;
private RuntimeProtectionStatusResponse runtimeProtectionStatus;
private String serialNumber;
private String serviceTag;
private SystemDataResponse systemData;
private @Nullable Map tags;
private String type;
private List virtualMachinesAssociatedIds;
public Builder() {}
public Builder(GetBareMetalMachineResult defaults) {
Objects.requireNonNull(defaults);
this.associatedResourceIds = defaults.associatedResourceIds;
this.bmcConnectionString = defaults.bmcConnectionString;
this.bmcCredentials = defaults.bmcCredentials;
this.bmcMacAddress = defaults.bmcMacAddress;
this.bootMacAddress = defaults.bootMacAddress;
this.clusterId = defaults.clusterId;
this.cordonStatus = defaults.cordonStatus;
this.detailedStatus = defaults.detailedStatus;
this.detailedStatusMessage = defaults.detailedStatusMessage;
this.extendedLocation = defaults.extendedLocation;
this.hardwareInventory = defaults.hardwareInventory;
this.hardwareValidationStatus = defaults.hardwareValidationStatus;
this.hybridAksClustersAssociatedIds = defaults.hybridAksClustersAssociatedIds;
this.id = defaults.id;
this.kubernetesNodeName = defaults.kubernetesNodeName;
this.kubernetesVersion = defaults.kubernetesVersion;
this.location = defaults.location;
this.machineDetails = defaults.machineDetails;
this.machineName = defaults.machineName;
this.machineRoles = defaults.machineRoles;
this.machineSkuId = defaults.machineSkuId;
this.name = defaults.name;
this.oamIpv4Address = defaults.oamIpv4Address;
this.oamIpv6Address = defaults.oamIpv6Address;
this.osImage = defaults.osImage;
this.powerState = defaults.powerState;
this.provisioningState = defaults.provisioningState;
this.rackId = defaults.rackId;
this.rackSlot = defaults.rackSlot;
this.readyState = defaults.readyState;
this.runtimeProtectionStatus = defaults.runtimeProtectionStatus;
this.serialNumber = defaults.serialNumber;
this.serviceTag = defaults.serviceTag;
this.systemData = defaults.systemData;
this.tags = defaults.tags;
this.type = defaults.type;
this.virtualMachinesAssociatedIds = defaults.virtualMachinesAssociatedIds;
}
@CustomType.Setter
public Builder associatedResourceIds(List associatedResourceIds) {
if (associatedResourceIds == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "associatedResourceIds");
}
this.associatedResourceIds = associatedResourceIds;
return this;
}
public Builder associatedResourceIds(String... associatedResourceIds) {
return associatedResourceIds(List.of(associatedResourceIds));
}
@CustomType.Setter
public Builder bmcConnectionString(String bmcConnectionString) {
if (bmcConnectionString == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "bmcConnectionString");
}
this.bmcConnectionString = bmcConnectionString;
return this;
}
@CustomType.Setter
public Builder bmcCredentials(AdministrativeCredentialsResponse bmcCredentials) {
if (bmcCredentials == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "bmcCredentials");
}
this.bmcCredentials = bmcCredentials;
return this;
}
@CustomType.Setter
public Builder bmcMacAddress(String bmcMacAddress) {
if (bmcMacAddress == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "bmcMacAddress");
}
this.bmcMacAddress = bmcMacAddress;
return this;
}
@CustomType.Setter
public Builder bootMacAddress(String bootMacAddress) {
if (bootMacAddress == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "bootMacAddress");
}
this.bootMacAddress = bootMacAddress;
return this;
}
@CustomType.Setter
public Builder clusterId(String clusterId) {
if (clusterId == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "clusterId");
}
this.clusterId = clusterId;
return this;
}
@CustomType.Setter
public Builder cordonStatus(String cordonStatus) {
if (cordonStatus == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "cordonStatus");
}
this.cordonStatus = cordonStatus;
return this;
}
@CustomType.Setter
public Builder detailedStatus(String detailedStatus) {
if (detailedStatus == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "detailedStatus");
}
this.detailedStatus = detailedStatus;
return this;
}
@CustomType.Setter
public Builder detailedStatusMessage(String detailedStatusMessage) {
if (detailedStatusMessage == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "detailedStatusMessage");
}
this.detailedStatusMessage = detailedStatusMessage;
return this;
}
@CustomType.Setter
public Builder extendedLocation(ExtendedLocationResponse extendedLocation) {
if (extendedLocation == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "extendedLocation");
}
this.extendedLocation = extendedLocation;
return this;
}
@CustomType.Setter
public Builder hardwareInventory(HardwareInventoryResponse hardwareInventory) {
if (hardwareInventory == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "hardwareInventory");
}
this.hardwareInventory = hardwareInventory;
return this;
}
@CustomType.Setter
public Builder hardwareValidationStatus(HardwareValidationStatusResponse hardwareValidationStatus) {
if (hardwareValidationStatus == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "hardwareValidationStatus");
}
this.hardwareValidationStatus = hardwareValidationStatus;
return this;
}
@CustomType.Setter
public Builder hybridAksClustersAssociatedIds(List hybridAksClustersAssociatedIds) {
if (hybridAksClustersAssociatedIds == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "hybridAksClustersAssociatedIds");
}
this.hybridAksClustersAssociatedIds = hybridAksClustersAssociatedIds;
return this;
}
public Builder hybridAksClustersAssociatedIds(String... hybridAksClustersAssociatedIds) {
return hybridAksClustersAssociatedIds(List.of(hybridAksClustersAssociatedIds));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder kubernetesNodeName(String kubernetesNodeName) {
if (kubernetesNodeName == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "kubernetesNodeName");
}
this.kubernetesNodeName = kubernetesNodeName;
return this;
}
@CustomType.Setter
public Builder kubernetesVersion(String kubernetesVersion) {
if (kubernetesVersion == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "kubernetesVersion");
}
this.kubernetesVersion = kubernetesVersion;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder machineDetails(String machineDetails) {
if (machineDetails == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "machineDetails");
}
this.machineDetails = machineDetails;
return this;
}
@CustomType.Setter
public Builder machineName(String machineName) {
if (machineName == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "machineName");
}
this.machineName = machineName;
return this;
}
@CustomType.Setter
public Builder machineRoles(List machineRoles) {
if (machineRoles == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "machineRoles");
}
this.machineRoles = machineRoles;
return this;
}
public Builder machineRoles(String... machineRoles) {
return machineRoles(List.of(machineRoles));
}
@CustomType.Setter
public Builder machineSkuId(String machineSkuId) {
if (machineSkuId == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "machineSkuId");
}
this.machineSkuId = machineSkuId;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder oamIpv4Address(String oamIpv4Address) {
if (oamIpv4Address == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "oamIpv4Address");
}
this.oamIpv4Address = oamIpv4Address;
return this;
}
@CustomType.Setter
public Builder oamIpv6Address(String oamIpv6Address) {
if (oamIpv6Address == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "oamIpv6Address");
}
this.oamIpv6Address = oamIpv6Address;
return this;
}
@CustomType.Setter
public Builder osImage(String osImage) {
if (osImage == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "osImage");
}
this.osImage = osImage;
return this;
}
@CustomType.Setter
public Builder powerState(String powerState) {
if (powerState == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "powerState");
}
this.powerState = powerState;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder rackId(String rackId) {
if (rackId == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "rackId");
}
this.rackId = rackId;
return this;
}
@CustomType.Setter
public Builder rackSlot(Double rackSlot) {
if (rackSlot == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "rackSlot");
}
this.rackSlot = rackSlot;
return this;
}
@CustomType.Setter
public Builder readyState(String readyState) {
if (readyState == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "readyState");
}
this.readyState = readyState;
return this;
}
@CustomType.Setter
public Builder runtimeProtectionStatus(RuntimeProtectionStatusResponse runtimeProtectionStatus) {
if (runtimeProtectionStatus == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "runtimeProtectionStatus");
}
this.runtimeProtectionStatus = runtimeProtectionStatus;
return this;
}
@CustomType.Setter
public Builder serialNumber(String serialNumber) {
if (serialNumber == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "serialNumber");
}
this.serialNumber = serialNumber;
return this;
}
@CustomType.Setter
public Builder serviceTag(String serviceTag) {
if (serviceTag == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "serviceTag");
}
this.serviceTag = serviceTag;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder virtualMachinesAssociatedIds(List virtualMachinesAssociatedIds) {
if (virtualMachinesAssociatedIds == null) {
throw new MissingRequiredPropertyException("GetBareMetalMachineResult", "virtualMachinesAssociatedIds");
}
this.virtualMachinesAssociatedIds = virtualMachinesAssociatedIds;
return this;
}
public Builder virtualMachinesAssociatedIds(String... virtualMachinesAssociatedIds) {
return virtualMachinesAssociatedIds(List.of(virtualMachinesAssociatedIds));
}
public GetBareMetalMachineResult build() {
final var _resultValue = new GetBareMetalMachineResult();
_resultValue.associatedResourceIds = associatedResourceIds;
_resultValue.bmcConnectionString = bmcConnectionString;
_resultValue.bmcCredentials = bmcCredentials;
_resultValue.bmcMacAddress = bmcMacAddress;
_resultValue.bootMacAddress = bootMacAddress;
_resultValue.clusterId = clusterId;
_resultValue.cordonStatus = cordonStatus;
_resultValue.detailedStatus = detailedStatus;
_resultValue.detailedStatusMessage = detailedStatusMessage;
_resultValue.extendedLocation = extendedLocation;
_resultValue.hardwareInventory = hardwareInventory;
_resultValue.hardwareValidationStatus = hardwareValidationStatus;
_resultValue.hybridAksClustersAssociatedIds = hybridAksClustersAssociatedIds;
_resultValue.id = id;
_resultValue.kubernetesNodeName = kubernetesNodeName;
_resultValue.kubernetesVersion = kubernetesVersion;
_resultValue.location = location;
_resultValue.machineDetails = machineDetails;
_resultValue.machineName = machineName;
_resultValue.machineRoles = machineRoles;
_resultValue.machineSkuId = machineSkuId;
_resultValue.name = name;
_resultValue.oamIpv4Address = oamIpv4Address;
_resultValue.oamIpv6Address = oamIpv6Address;
_resultValue.osImage = osImage;
_resultValue.powerState = powerState;
_resultValue.provisioningState = provisioningState;
_resultValue.rackId = rackId;
_resultValue.rackSlot = rackSlot;
_resultValue.readyState = readyState;
_resultValue.runtimeProtectionStatus = runtimeProtectionStatus;
_resultValue.serialNumber = serialNumber;
_resultValue.serviceTag = serviceTag;
_resultValue.systemData = systemData;
_resultValue.tags = tags;
_resultValue.type = type;
_resultValue.virtualMachinesAssociatedIds = virtualMachinesAssociatedIds;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy