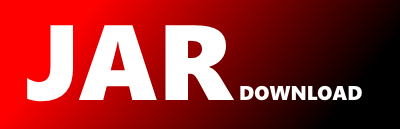
com.pulumi.azurenative.networkcloud.outputs.InitialAgentPoolConfigurationResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.networkcloud.outputs;
import com.pulumi.azurenative.networkcloud.outputs.AdministratorConfigurationResponse;
import com.pulumi.azurenative.networkcloud.outputs.AgentOptionsResponse;
import com.pulumi.azurenative.networkcloud.outputs.AgentPoolUpgradeSettingsResponse;
import com.pulumi.azurenative.networkcloud.outputs.AttachedNetworkConfigurationResponse;
import com.pulumi.azurenative.networkcloud.outputs.KubernetesLabelResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class InitialAgentPoolConfigurationResponse {
/**
* @return The administrator credentials to be used for the nodes in this agent pool.
*
*/
private @Nullable AdministratorConfigurationResponse administratorConfiguration;
/**
* @return The configurations that will be applied to each agent in this agent pool.
*
*/
private @Nullable AgentOptionsResponse agentOptions;
/**
* @return The configuration of networks being attached to the agent pool for use by the workloads that run on this Kubernetes cluster.
*
*/
private @Nullable AttachedNetworkConfigurationResponse attachedNetworkConfiguration;
/**
* @return The list of availability zones of the Network Cloud cluster used for the provisioning of nodes in this agent pool. If not specified, all availability zones will be used.
*
*/
private @Nullable List availabilityZones;
/**
* @return The number of virtual machines that use this configuration.
*
*/
private Double count;
/**
* @return The labels applied to the nodes in this agent pool.
*
*/
private @Nullable List labels;
/**
* @return The selection of how this agent pool is utilized, either as a system pool or a user pool. System pools run the features and critical services for the Kubernetes Cluster, while user pools are dedicated to user workloads. Every Kubernetes cluster must contain at least one system node pool with at least one node.
*
*/
private String mode;
/**
* @return The name that will be used for the agent pool resource representing this agent pool.
*
*/
private String name;
/**
* @return The taints applied to the nodes in this agent pool.
*
*/
private @Nullable List taints;
/**
* @return The configuration of the agent pool.
*
*/
private @Nullable AgentPoolUpgradeSettingsResponse upgradeSettings;
/**
* @return The name of the VM SKU that determines the size of resources allocated for node VMs.
*
*/
private String vmSkuName;
private InitialAgentPoolConfigurationResponse() {}
/**
* @return The administrator credentials to be used for the nodes in this agent pool.
*
*/
public Optional administratorConfiguration() {
return Optional.ofNullable(this.administratorConfiguration);
}
/**
* @return The configurations that will be applied to each agent in this agent pool.
*
*/
public Optional agentOptions() {
return Optional.ofNullable(this.agentOptions);
}
/**
* @return The configuration of networks being attached to the agent pool for use by the workloads that run on this Kubernetes cluster.
*
*/
public Optional attachedNetworkConfiguration() {
return Optional.ofNullable(this.attachedNetworkConfiguration);
}
/**
* @return The list of availability zones of the Network Cloud cluster used for the provisioning of nodes in this agent pool. If not specified, all availability zones will be used.
*
*/
public List availabilityZones() {
return this.availabilityZones == null ? List.of() : this.availabilityZones;
}
/**
* @return The number of virtual machines that use this configuration.
*
*/
public Double count() {
return this.count;
}
/**
* @return The labels applied to the nodes in this agent pool.
*
*/
public List labels() {
return this.labels == null ? List.of() : this.labels;
}
/**
* @return The selection of how this agent pool is utilized, either as a system pool or a user pool. System pools run the features and critical services for the Kubernetes Cluster, while user pools are dedicated to user workloads. Every Kubernetes cluster must contain at least one system node pool with at least one node.
*
*/
public String mode() {
return this.mode;
}
/**
* @return The name that will be used for the agent pool resource representing this agent pool.
*
*/
public String name() {
return this.name;
}
/**
* @return The taints applied to the nodes in this agent pool.
*
*/
public List taints() {
return this.taints == null ? List.of() : this.taints;
}
/**
* @return The configuration of the agent pool.
*
*/
public Optional upgradeSettings() {
return Optional.ofNullable(this.upgradeSettings);
}
/**
* @return The name of the VM SKU that determines the size of resources allocated for node VMs.
*
*/
public String vmSkuName() {
return this.vmSkuName;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(InitialAgentPoolConfigurationResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable AdministratorConfigurationResponse administratorConfiguration;
private @Nullable AgentOptionsResponse agentOptions;
private @Nullable AttachedNetworkConfigurationResponse attachedNetworkConfiguration;
private @Nullable List availabilityZones;
private Double count;
private @Nullable List labels;
private String mode;
private String name;
private @Nullable List taints;
private @Nullable AgentPoolUpgradeSettingsResponse upgradeSettings;
private String vmSkuName;
public Builder() {}
public Builder(InitialAgentPoolConfigurationResponse defaults) {
Objects.requireNonNull(defaults);
this.administratorConfiguration = defaults.administratorConfiguration;
this.agentOptions = defaults.agentOptions;
this.attachedNetworkConfiguration = defaults.attachedNetworkConfiguration;
this.availabilityZones = defaults.availabilityZones;
this.count = defaults.count;
this.labels = defaults.labels;
this.mode = defaults.mode;
this.name = defaults.name;
this.taints = defaults.taints;
this.upgradeSettings = defaults.upgradeSettings;
this.vmSkuName = defaults.vmSkuName;
}
@CustomType.Setter
public Builder administratorConfiguration(@Nullable AdministratorConfigurationResponse administratorConfiguration) {
this.administratorConfiguration = administratorConfiguration;
return this;
}
@CustomType.Setter
public Builder agentOptions(@Nullable AgentOptionsResponse agentOptions) {
this.agentOptions = agentOptions;
return this;
}
@CustomType.Setter
public Builder attachedNetworkConfiguration(@Nullable AttachedNetworkConfigurationResponse attachedNetworkConfiguration) {
this.attachedNetworkConfiguration = attachedNetworkConfiguration;
return this;
}
@CustomType.Setter
public Builder availabilityZones(@Nullable List availabilityZones) {
this.availabilityZones = availabilityZones;
return this;
}
public Builder availabilityZones(String... availabilityZones) {
return availabilityZones(List.of(availabilityZones));
}
@CustomType.Setter
public Builder count(Double count) {
if (count == null) {
throw new MissingRequiredPropertyException("InitialAgentPoolConfigurationResponse", "count");
}
this.count = count;
return this;
}
@CustomType.Setter
public Builder labels(@Nullable List labels) {
this.labels = labels;
return this;
}
public Builder labels(KubernetesLabelResponse... labels) {
return labels(List.of(labels));
}
@CustomType.Setter
public Builder mode(String mode) {
if (mode == null) {
throw new MissingRequiredPropertyException("InitialAgentPoolConfigurationResponse", "mode");
}
this.mode = mode;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("InitialAgentPoolConfigurationResponse", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder taints(@Nullable List taints) {
this.taints = taints;
return this;
}
public Builder taints(KubernetesLabelResponse... taints) {
return taints(List.of(taints));
}
@CustomType.Setter
public Builder upgradeSettings(@Nullable AgentPoolUpgradeSettingsResponse upgradeSettings) {
this.upgradeSettings = upgradeSettings;
return this;
}
@CustomType.Setter
public Builder vmSkuName(String vmSkuName) {
if (vmSkuName == null) {
throw new MissingRequiredPropertyException("InitialAgentPoolConfigurationResponse", "vmSkuName");
}
this.vmSkuName = vmSkuName;
return this;
}
public InitialAgentPoolConfigurationResponse build() {
final var _resultValue = new InitialAgentPoolConfigurationResponse();
_resultValue.administratorConfiguration = administratorConfiguration;
_resultValue.agentOptions = agentOptions;
_resultValue.attachedNetworkConfiguration = attachedNetworkConfiguration;
_resultValue.availabilityZones = availabilityZones;
_resultValue.count = count;
_resultValue.labels = labels;
_resultValue.mode = mode;
_resultValue.name = name;
_resultValue.taints = taints;
_resultValue.upgradeSettings = upgradeSettings;
_resultValue.vmSkuName = vmSkuName;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy