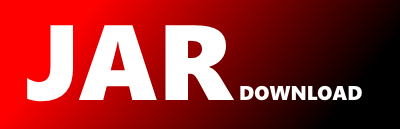
com.pulumi.azurenative.networkcloud.outputs.NetworkConfigurationResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.networkcloud.outputs;
import com.pulumi.azurenative.networkcloud.outputs.AttachedNetworkConfigurationResponse;
import com.pulumi.azurenative.networkcloud.outputs.BgpServiceLoadBalancerConfigurationResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class NetworkConfigurationResponse {
/**
* @return The configuration of networks being attached to the cluster for use by the workloads that run on this Kubernetes cluster.
*
*/
private @Nullable AttachedNetworkConfigurationResponse attachedNetworkConfiguration;
/**
* @return The configuration of the BGP service load balancer for this Kubernetes cluster.
*
*/
private @Nullable BgpServiceLoadBalancerConfigurationResponse bgpServiceLoadBalancerConfiguration;
/**
* @return The resource ID of the associated Cloud Services network.
*
*/
private String cloudServicesNetworkId;
/**
* @return The resource ID of the Layer 3 network that is used for creation of the Container Networking Interface network.
*
*/
private String cniNetworkId;
/**
* @return The IP address assigned to the Kubernetes DNS service. It must be within the Kubernetes service address range specified in service CIDR.
*
*/
private @Nullable String dnsServiceIp;
/**
* @return The CIDR notation IP ranges from which to assign pod IPs. One IPv4 CIDR is expected for single-stack networking. Two CIDRs, one for each IP family (IPv4/IPv6), is expected for dual-stack networking.
*
*/
private @Nullable List podCidrs;
/**
* @return The CIDR notation IP ranges from which to assign service IPs. One IPv4 CIDR is expected for single-stack networking. Two CIDRs, one for each IP family (IPv4/IPv6), is expected for dual-stack networking.
*
*/
private @Nullable List serviceCidrs;
private NetworkConfigurationResponse() {}
/**
* @return The configuration of networks being attached to the cluster for use by the workloads that run on this Kubernetes cluster.
*
*/
public Optional attachedNetworkConfiguration() {
return Optional.ofNullable(this.attachedNetworkConfiguration);
}
/**
* @return The configuration of the BGP service load balancer for this Kubernetes cluster.
*
*/
public Optional bgpServiceLoadBalancerConfiguration() {
return Optional.ofNullable(this.bgpServiceLoadBalancerConfiguration);
}
/**
* @return The resource ID of the associated Cloud Services network.
*
*/
public String cloudServicesNetworkId() {
return this.cloudServicesNetworkId;
}
/**
* @return The resource ID of the Layer 3 network that is used for creation of the Container Networking Interface network.
*
*/
public String cniNetworkId() {
return this.cniNetworkId;
}
/**
* @return The IP address assigned to the Kubernetes DNS service. It must be within the Kubernetes service address range specified in service CIDR.
*
*/
public Optional dnsServiceIp() {
return Optional.ofNullable(this.dnsServiceIp);
}
/**
* @return The CIDR notation IP ranges from which to assign pod IPs. One IPv4 CIDR is expected for single-stack networking. Two CIDRs, one for each IP family (IPv4/IPv6), is expected for dual-stack networking.
*
*/
public List podCidrs() {
return this.podCidrs == null ? List.of() : this.podCidrs;
}
/**
* @return The CIDR notation IP ranges from which to assign service IPs. One IPv4 CIDR is expected for single-stack networking. Two CIDRs, one for each IP family (IPv4/IPv6), is expected for dual-stack networking.
*
*/
public List serviceCidrs() {
return this.serviceCidrs == null ? List.of() : this.serviceCidrs;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(NetworkConfigurationResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable AttachedNetworkConfigurationResponse attachedNetworkConfiguration;
private @Nullable BgpServiceLoadBalancerConfigurationResponse bgpServiceLoadBalancerConfiguration;
private String cloudServicesNetworkId;
private String cniNetworkId;
private @Nullable String dnsServiceIp;
private @Nullable List podCidrs;
private @Nullable List serviceCidrs;
public Builder() {}
public Builder(NetworkConfigurationResponse defaults) {
Objects.requireNonNull(defaults);
this.attachedNetworkConfiguration = defaults.attachedNetworkConfiguration;
this.bgpServiceLoadBalancerConfiguration = defaults.bgpServiceLoadBalancerConfiguration;
this.cloudServicesNetworkId = defaults.cloudServicesNetworkId;
this.cniNetworkId = defaults.cniNetworkId;
this.dnsServiceIp = defaults.dnsServiceIp;
this.podCidrs = defaults.podCidrs;
this.serviceCidrs = defaults.serviceCidrs;
}
@CustomType.Setter
public Builder attachedNetworkConfiguration(@Nullable AttachedNetworkConfigurationResponse attachedNetworkConfiguration) {
this.attachedNetworkConfiguration = attachedNetworkConfiguration;
return this;
}
@CustomType.Setter
public Builder bgpServiceLoadBalancerConfiguration(@Nullable BgpServiceLoadBalancerConfigurationResponse bgpServiceLoadBalancerConfiguration) {
this.bgpServiceLoadBalancerConfiguration = bgpServiceLoadBalancerConfiguration;
return this;
}
@CustomType.Setter
public Builder cloudServicesNetworkId(String cloudServicesNetworkId) {
if (cloudServicesNetworkId == null) {
throw new MissingRequiredPropertyException("NetworkConfigurationResponse", "cloudServicesNetworkId");
}
this.cloudServicesNetworkId = cloudServicesNetworkId;
return this;
}
@CustomType.Setter
public Builder cniNetworkId(String cniNetworkId) {
if (cniNetworkId == null) {
throw new MissingRequiredPropertyException("NetworkConfigurationResponse", "cniNetworkId");
}
this.cniNetworkId = cniNetworkId;
return this;
}
@CustomType.Setter
public Builder dnsServiceIp(@Nullable String dnsServiceIp) {
this.dnsServiceIp = dnsServiceIp;
return this;
}
@CustomType.Setter
public Builder podCidrs(@Nullable List podCidrs) {
this.podCidrs = podCidrs;
return this;
}
public Builder podCidrs(String... podCidrs) {
return podCidrs(List.of(podCidrs));
}
@CustomType.Setter
public Builder serviceCidrs(@Nullable List serviceCidrs) {
this.serviceCidrs = serviceCidrs;
return this;
}
public Builder serviceCidrs(String... serviceCidrs) {
return serviceCidrs(List.of(serviceCidrs));
}
public NetworkConfigurationResponse build() {
final var _resultValue = new NetworkConfigurationResponse();
_resultValue.attachedNetworkConfiguration = attachedNetworkConfiguration;
_resultValue.bgpServiceLoadBalancerConfiguration = bgpServiceLoadBalancerConfiguration;
_resultValue.cloudServicesNetworkId = cloudServicesNetworkId;
_resultValue.cniNetworkId = cniNetworkId;
_resultValue.dnsServiceIp = dnsServiceIp;
_resultValue.podCidrs = podCidrs;
_resultValue.serviceCidrs = serviceCidrs;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy