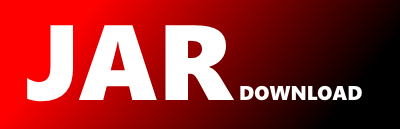
com.pulumi.azurenative.notificationhubs.outputs.ApnsCredentialPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.notificationhubs.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ApnsCredentialPropertiesResponse {
/**
* @return Gets or sets the APNS certificate.
*
*/
private @Nullable String apnsCertificate;
/**
* @return Gets or sets the issuer (iss) registered claim key, whose value is
* your 10-character Team ID, obtained from your developer account
*
*/
private @Nullable String appId;
/**
* @return Gets or sets the name of the application
*
*/
private @Nullable String appName;
/**
* @return Gets or sets the certificate key.
*
*/
private @Nullable String certificateKey;
/**
* @return Gets or sets the endpoint of this credential.
*
*/
private String endpoint;
/**
* @return Gets or sets a 10-character key identifier (kid) key, obtained from
* your developer account
*
*/
private @Nullable String keyId;
/**
* @return Gets or sets the APNS certificate Thumbprint
*
*/
private @Nullable String thumbprint;
/**
* @return Gets or sets provider Authentication Token, obtained through your
* developer account
*
*/
private @Nullable String token;
private ApnsCredentialPropertiesResponse() {}
/**
* @return Gets or sets the APNS certificate.
*
*/
public Optional apnsCertificate() {
return Optional.ofNullable(this.apnsCertificate);
}
/**
* @return Gets or sets the issuer (iss) registered claim key, whose value is
* your 10-character Team ID, obtained from your developer account
*
*/
public Optional appId() {
return Optional.ofNullable(this.appId);
}
/**
* @return Gets or sets the name of the application
*
*/
public Optional appName() {
return Optional.ofNullable(this.appName);
}
/**
* @return Gets or sets the certificate key.
*
*/
public Optional certificateKey() {
return Optional.ofNullable(this.certificateKey);
}
/**
* @return Gets or sets the endpoint of this credential.
*
*/
public String endpoint() {
return this.endpoint;
}
/**
* @return Gets or sets a 10-character key identifier (kid) key, obtained from
* your developer account
*
*/
public Optional keyId() {
return Optional.ofNullable(this.keyId);
}
/**
* @return Gets or sets the APNS certificate Thumbprint
*
*/
public Optional thumbprint() {
return Optional.ofNullable(this.thumbprint);
}
/**
* @return Gets or sets provider Authentication Token, obtained through your
* developer account
*
*/
public Optional token() {
return Optional.ofNullable(this.token);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ApnsCredentialPropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String apnsCertificate;
private @Nullable String appId;
private @Nullable String appName;
private @Nullable String certificateKey;
private String endpoint;
private @Nullable String keyId;
private @Nullable String thumbprint;
private @Nullable String token;
public Builder() {}
public Builder(ApnsCredentialPropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.apnsCertificate = defaults.apnsCertificate;
this.appId = defaults.appId;
this.appName = defaults.appName;
this.certificateKey = defaults.certificateKey;
this.endpoint = defaults.endpoint;
this.keyId = defaults.keyId;
this.thumbprint = defaults.thumbprint;
this.token = defaults.token;
}
@CustomType.Setter
public Builder apnsCertificate(@Nullable String apnsCertificate) {
this.apnsCertificate = apnsCertificate;
return this;
}
@CustomType.Setter
public Builder appId(@Nullable String appId) {
this.appId = appId;
return this;
}
@CustomType.Setter
public Builder appName(@Nullable String appName) {
this.appName = appName;
return this;
}
@CustomType.Setter
public Builder certificateKey(@Nullable String certificateKey) {
this.certificateKey = certificateKey;
return this;
}
@CustomType.Setter
public Builder endpoint(String endpoint) {
if (endpoint == null) {
throw new MissingRequiredPropertyException("ApnsCredentialPropertiesResponse", "endpoint");
}
this.endpoint = endpoint;
return this;
}
@CustomType.Setter
public Builder keyId(@Nullable String keyId) {
this.keyId = keyId;
return this;
}
@CustomType.Setter
public Builder thumbprint(@Nullable String thumbprint) {
this.thumbprint = thumbprint;
return this;
}
@CustomType.Setter
public Builder token(@Nullable String token) {
this.token = token;
return this;
}
public ApnsCredentialPropertiesResponse build() {
final var _resultValue = new ApnsCredentialPropertiesResponse();
_resultValue.apnsCertificate = apnsCertificate;
_resultValue.appId = appId;
_resultValue.appName = appName;
_resultValue.certificateKey = certificateKey;
_resultValue.endpoint = endpoint;
_resultValue.keyId = keyId;
_resultValue.thumbprint = thumbprint;
_resultValue.token = token;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy