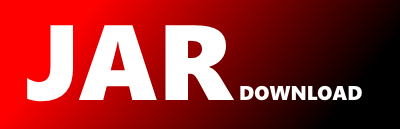
com.pulumi.azurenative.offazure.outputs.SiteHealthSummaryResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.offazure.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class SiteHealthSummaryResponse {
/**
* @return Gets or sets the count of affected objects.
*
*/
private @Nullable Double affectedObjectsCount;
/**
* @return Gets the affected resource type.
*
*/
private String affectedResourceType;
/**
* @return Gets or sets the affected resources.
*
*/
private @Nullable List affectedResources;
/**
* @return Gets the appliance name.
*
*/
private String applianceName;
/**
* @return Gets the error code.
*
*/
private String errorCode;
/**
* @return Gets the error Id.
*
*/
private Double errorId;
/**
* @return Gets the error message.
*
*/
private String errorMessage;
/**
* @return Gets or sets sources of the exception.
*
*/
private @Nullable List fabricLayoutUpdateSources;
/**
* @return Gets or sets the hit count of the error.
*
*/
private @Nullable Double hitCount;
/**
* @return Gets the remediation guidance.
*
*/
private String remediationGuidance;
/**
* @return Gets the severity of error.
*
*/
private String severity;
/**
* @return Gets the summary message.
*
*/
private String summaryMessage;
private SiteHealthSummaryResponse() {}
/**
* @return Gets or sets the count of affected objects.
*
*/
public Optional affectedObjectsCount() {
return Optional.ofNullable(this.affectedObjectsCount);
}
/**
* @return Gets the affected resource type.
*
*/
public String affectedResourceType() {
return this.affectedResourceType;
}
/**
* @return Gets or sets the affected resources.
*
*/
public List affectedResources() {
return this.affectedResources == null ? List.of() : this.affectedResources;
}
/**
* @return Gets the appliance name.
*
*/
public String applianceName() {
return this.applianceName;
}
/**
* @return Gets the error code.
*
*/
public String errorCode() {
return this.errorCode;
}
/**
* @return Gets the error Id.
*
*/
public Double errorId() {
return this.errorId;
}
/**
* @return Gets the error message.
*
*/
public String errorMessage() {
return this.errorMessage;
}
/**
* @return Gets or sets sources of the exception.
*
*/
public List fabricLayoutUpdateSources() {
return this.fabricLayoutUpdateSources == null ? List.of() : this.fabricLayoutUpdateSources;
}
/**
* @return Gets or sets the hit count of the error.
*
*/
public Optional hitCount() {
return Optional.ofNullable(this.hitCount);
}
/**
* @return Gets the remediation guidance.
*
*/
public String remediationGuidance() {
return this.remediationGuidance;
}
/**
* @return Gets the severity of error.
*
*/
public String severity() {
return this.severity;
}
/**
* @return Gets the summary message.
*
*/
public String summaryMessage() {
return this.summaryMessage;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(SiteHealthSummaryResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Double affectedObjectsCount;
private String affectedResourceType;
private @Nullable List affectedResources;
private String applianceName;
private String errorCode;
private Double errorId;
private String errorMessage;
private @Nullable List fabricLayoutUpdateSources;
private @Nullable Double hitCount;
private String remediationGuidance;
private String severity;
private String summaryMessage;
public Builder() {}
public Builder(SiteHealthSummaryResponse defaults) {
Objects.requireNonNull(defaults);
this.affectedObjectsCount = defaults.affectedObjectsCount;
this.affectedResourceType = defaults.affectedResourceType;
this.affectedResources = defaults.affectedResources;
this.applianceName = defaults.applianceName;
this.errorCode = defaults.errorCode;
this.errorId = defaults.errorId;
this.errorMessage = defaults.errorMessage;
this.fabricLayoutUpdateSources = defaults.fabricLayoutUpdateSources;
this.hitCount = defaults.hitCount;
this.remediationGuidance = defaults.remediationGuidance;
this.severity = defaults.severity;
this.summaryMessage = defaults.summaryMessage;
}
@CustomType.Setter
public Builder affectedObjectsCount(@Nullable Double affectedObjectsCount) {
this.affectedObjectsCount = affectedObjectsCount;
return this;
}
@CustomType.Setter
public Builder affectedResourceType(String affectedResourceType) {
if (affectedResourceType == null) {
throw new MissingRequiredPropertyException("SiteHealthSummaryResponse", "affectedResourceType");
}
this.affectedResourceType = affectedResourceType;
return this;
}
@CustomType.Setter
public Builder affectedResources(@Nullable List affectedResources) {
this.affectedResources = affectedResources;
return this;
}
public Builder affectedResources(String... affectedResources) {
return affectedResources(List.of(affectedResources));
}
@CustomType.Setter
public Builder applianceName(String applianceName) {
if (applianceName == null) {
throw new MissingRequiredPropertyException("SiteHealthSummaryResponse", "applianceName");
}
this.applianceName = applianceName;
return this;
}
@CustomType.Setter
public Builder errorCode(String errorCode) {
if (errorCode == null) {
throw new MissingRequiredPropertyException("SiteHealthSummaryResponse", "errorCode");
}
this.errorCode = errorCode;
return this;
}
@CustomType.Setter
public Builder errorId(Double errorId) {
if (errorId == null) {
throw new MissingRequiredPropertyException("SiteHealthSummaryResponse", "errorId");
}
this.errorId = errorId;
return this;
}
@CustomType.Setter
public Builder errorMessage(String errorMessage) {
if (errorMessage == null) {
throw new MissingRequiredPropertyException("SiteHealthSummaryResponse", "errorMessage");
}
this.errorMessage = errorMessage;
return this;
}
@CustomType.Setter
public Builder fabricLayoutUpdateSources(@Nullable List fabricLayoutUpdateSources) {
this.fabricLayoutUpdateSources = fabricLayoutUpdateSources;
return this;
}
public Builder fabricLayoutUpdateSources(String... fabricLayoutUpdateSources) {
return fabricLayoutUpdateSources(List.of(fabricLayoutUpdateSources));
}
@CustomType.Setter
public Builder hitCount(@Nullable Double hitCount) {
this.hitCount = hitCount;
return this;
}
@CustomType.Setter
public Builder remediationGuidance(String remediationGuidance) {
if (remediationGuidance == null) {
throw new MissingRequiredPropertyException("SiteHealthSummaryResponse", "remediationGuidance");
}
this.remediationGuidance = remediationGuidance;
return this;
}
@CustomType.Setter
public Builder severity(String severity) {
if (severity == null) {
throw new MissingRequiredPropertyException("SiteHealthSummaryResponse", "severity");
}
this.severity = severity;
return this;
}
@CustomType.Setter
public Builder summaryMessage(String summaryMessage) {
if (summaryMessage == null) {
throw new MissingRequiredPropertyException("SiteHealthSummaryResponse", "summaryMessage");
}
this.summaryMessage = summaryMessage;
return this;
}
public SiteHealthSummaryResponse build() {
final var _resultValue = new SiteHealthSummaryResponse();
_resultValue.affectedObjectsCount = affectedObjectsCount;
_resultValue.affectedResourceType = affectedResourceType;
_resultValue.affectedResources = affectedResources;
_resultValue.applianceName = applianceName;
_resultValue.errorCode = errorCode;
_resultValue.errorId = errorId;
_resultValue.errorMessage = errorMessage;
_resultValue.fabricLayoutUpdateSources = fabricLayoutUpdateSources;
_resultValue.hitCount = hitCount;
_resultValue.remediationGuidance = remediationGuidance;
_resultValue.severity = severity;
_resultValue.summaryMessage = summaryMessage;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy