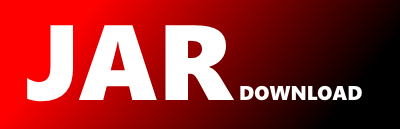
com.pulumi.azurenative.offazurespringboot.outputs.SpringbootappsPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.offazurespringboot.outputs;
import com.pulumi.azurenative.offazurespringboot.outputs.ErrorResponse;
import com.pulumi.azurenative.offazurespringboot.outputs.SpringbootappsPropertiesResponseApplicationConfigurations;
import com.pulumi.azurenative.offazurespringboot.outputs.SpringbootappsPropertiesResponseInstances;
import com.pulumi.azurenative.offazurespringboot.outputs.SpringbootappsPropertiesResponseMiscs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class SpringbootappsPropertiesResponse {
/**
* @return The name of SpringBootApp.
*
*/
private @Nullable String appName;
/**
* @return The application port.
*
*/
private @Nullable Integer appPort;
/**
* @return The application type, whether it is a SpringBoot app.
*
*/
private @Nullable String appType;
/**
* @return The application configuration file list.
*
*/
private @Nullable List applicationConfigurations;
/**
* @return The artifact name of SpringBootApp.
*
*/
private @Nullable String artifactName;
/**
* @return The application binding port list.
*
*/
private @Nullable List bindingPorts;
/**
* @return The jdk version in build.
*
*/
private @Nullable String buildJdkVersion;
/**
* @return The certificate file list.
*
*/
private @Nullable List certificates;
/**
* @return The checksum of jar file.
*
*/
private @Nullable String checksum;
/**
* @return The connection string list.
*
*/
private @Nullable List connectionStrings;
/**
* @return The dependency list.
*
*/
private @Nullable List dependencies;
/**
* @return The environment variable list.
*
*/
private @Nullable List environments;
/**
* @return The list of errors.
*
*/
private @Nullable List errors;
/**
* @return The total instance count the app deployed.
*
*/
private @Nullable Integer instanceCount;
/**
* @return The breakdown info for app instances on all the servers
*
*/
private @Nullable List instances;
/**
* @return The jar file location on the server.
*
*/
private @Nullable String jarFileLocation;
/**
* @return The jvm heap memory allocated.
*
*/
private @Nullable Integer jvmMemoryInMB;
/**
* @return The jvm options.
*
*/
private @Nullable List jvmOptions;
/**
* @return Resource labels
*
*/
private @Nullable Map labels;
/**
* @return Time when this springbootapps jar file was last modified.
*
*/
private @Nullable String lastModifiedTime;
/**
* @return Time when this springbootapps instance was last refreshed.
*
*/
private @Nullable String lastUpdatedTime;
/**
* @return The machine ARM id list the app belongs to.
*
*/
private @Nullable List machineArmIds;
/**
* @return The other types of date collected.
*
*/
private @Nullable List miscs;
/**
* @return The resource provisioning state.
*
*/
private String provisioningState;
/**
* @return The jdk version installed on server
*
*/
private @Nullable String runtimeJdkVersion;
/**
* @return The server list the app installed
*
*/
private @Nullable List servers;
/**
* @return The spring boot version.
*
*/
private @Nullable String springBootVersion;
/**
* @return The static content location list.
*
*/
private @Nullable List staticContentLocations;
private SpringbootappsPropertiesResponse() {}
/**
* @return The name of SpringBootApp.
*
*/
public Optional appName() {
return Optional.ofNullable(this.appName);
}
/**
* @return The application port.
*
*/
public Optional appPort() {
return Optional.ofNullable(this.appPort);
}
/**
* @return The application type, whether it is a SpringBoot app.
*
*/
public Optional appType() {
return Optional.ofNullable(this.appType);
}
/**
* @return The application configuration file list.
*
*/
public List applicationConfigurations() {
return this.applicationConfigurations == null ? List.of() : this.applicationConfigurations;
}
/**
* @return The artifact name of SpringBootApp.
*
*/
public Optional artifactName() {
return Optional.ofNullable(this.artifactName);
}
/**
* @return The application binding port list.
*
*/
public List bindingPorts() {
return this.bindingPorts == null ? List.of() : this.bindingPorts;
}
/**
* @return The jdk version in build.
*
*/
public Optional buildJdkVersion() {
return Optional.ofNullable(this.buildJdkVersion);
}
/**
* @return The certificate file list.
*
*/
public List certificates() {
return this.certificates == null ? List.of() : this.certificates;
}
/**
* @return The checksum of jar file.
*
*/
public Optional checksum() {
return Optional.ofNullable(this.checksum);
}
/**
* @return The connection string list.
*
*/
public List connectionStrings() {
return this.connectionStrings == null ? List.of() : this.connectionStrings;
}
/**
* @return The dependency list.
*
*/
public List dependencies() {
return this.dependencies == null ? List.of() : this.dependencies;
}
/**
* @return The environment variable list.
*
*/
public List environments() {
return this.environments == null ? List.of() : this.environments;
}
/**
* @return The list of errors.
*
*/
public List errors() {
return this.errors == null ? List.of() : this.errors;
}
/**
* @return The total instance count the app deployed.
*
*/
public Optional instanceCount() {
return Optional.ofNullable(this.instanceCount);
}
/**
* @return The breakdown info for app instances on all the servers
*
*/
public List instances() {
return this.instances == null ? List.of() : this.instances;
}
/**
* @return The jar file location on the server.
*
*/
public Optional jarFileLocation() {
return Optional.ofNullable(this.jarFileLocation);
}
/**
* @return The jvm heap memory allocated.
*
*/
public Optional jvmMemoryInMB() {
return Optional.ofNullable(this.jvmMemoryInMB);
}
/**
* @return The jvm options.
*
*/
public List jvmOptions() {
return this.jvmOptions == null ? List.of() : this.jvmOptions;
}
/**
* @return Resource labels
*
*/
public Map labels() {
return this.labels == null ? Map.of() : this.labels;
}
/**
* @return Time when this springbootapps jar file was last modified.
*
*/
public Optional lastModifiedTime() {
return Optional.ofNullable(this.lastModifiedTime);
}
/**
* @return Time when this springbootapps instance was last refreshed.
*
*/
public Optional lastUpdatedTime() {
return Optional.ofNullable(this.lastUpdatedTime);
}
/**
* @return The machine ARM id list the app belongs to.
*
*/
public List machineArmIds() {
return this.machineArmIds == null ? List.of() : this.machineArmIds;
}
/**
* @return The other types of date collected.
*
*/
public List miscs() {
return this.miscs == null ? List.of() : this.miscs;
}
/**
* @return The resource provisioning state.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The jdk version installed on server
*
*/
public Optional runtimeJdkVersion() {
return Optional.ofNullable(this.runtimeJdkVersion);
}
/**
* @return The server list the app installed
*
*/
public List servers() {
return this.servers == null ? List.of() : this.servers;
}
/**
* @return The spring boot version.
*
*/
public Optional springBootVersion() {
return Optional.ofNullable(this.springBootVersion);
}
/**
* @return The static content location list.
*
*/
public List staticContentLocations() {
return this.staticContentLocations == null ? List.of() : this.staticContentLocations;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(SpringbootappsPropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String appName;
private @Nullable Integer appPort;
private @Nullable String appType;
private @Nullable List applicationConfigurations;
private @Nullable String artifactName;
private @Nullable List bindingPorts;
private @Nullable String buildJdkVersion;
private @Nullable List certificates;
private @Nullable String checksum;
private @Nullable List connectionStrings;
private @Nullable List dependencies;
private @Nullable List environments;
private @Nullable List errors;
private @Nullable Integer instanceCount;
private @Nullable List instances;
private @Nullable String jarFileLocation;
private @Nullable Integer jvmMemoryInMB;
private @Nullable List jvmOptions;
private @Nullable Map labels;
private @Nullable String lastModifiedTime;
private @Nullable String lastUpdatedTime;
private @Nullable List machineArmIds;
private @Nullable List miscs;
private String provisioningState;
private @Nullable String runtimeJdkVersion;
private @Nullable List servers;
private @Nullable String springBootVersion;
private @Nullable List staticContentLocations;
public Builder() {}
public Builder(SpringbootappsPropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.appName = defaults.appName;
this.appPort = defaults.appPort;
this.appType = defaults.appType;
this.applicationConfigurations = defaults.applicationConfigurations;
this.artifactName = defaults.artifactName;
this.bindingPorts = defaults.bindingPorts;
this.buildJdkVersion = defaults.buildJdkVersion;
this.certificates = defaults.certificates;
this.checksum = defaults.checksum;
this.connectionStrings = defaults.connectionStrings;
this.dependencies = defaults.dependencies;
this.environments = defaults.environments;
this.errors = defaults.errors;
this.instanceCount = defaults.instanceCount;
this.instances = defaults.instances;
this.jarFileLocation = defaults.jarFileLocation;
this.jvmMemoryInMB = defaults.jvmMemoryInMB;
this.jvmOptions = defaults.jvmOptions;
this.labels = defaults.labels;
this.lastModifiedTime = defaults.lastModifiedTime;
this.lastUpdatedTime = defaults.lastUpdatedTime;
this.machineArmIds = defaults.machineArmIds;
this.miscs = defaults.miscs;
this.provisioningState = defaults.provisioningState;
this.runtimeJdkVersion = defaults.runtimeJdkVersion;
this.servers = defaults.servers;
this.springBootVersion = defaults.springBootVersion;
this.staticContentLocations = defaults.staticContentLocations;
}
@CustomType.Setter
public Builder appName(@Nullable String appName) {
this.appName = appName;
return this;
}
@CustomType.Setter
public Builder appPort(@Nullable Integer appPort) {
this.appPort = appPort;
return this;
}
@CustomType.Setter
public Builder appType(@Nullable String appType) {
this.appType = appType;
return this;
}
@CustomType.Setter
public Builder applicationConfigurations(@Nullable List applicationConfigurations) {
this.applicationConfigurations = applicationConfigurations;
return this;
}
public Builder applicationConfigurations(SpringbootappsPropertiesResponseApplicationConfigurations... applicationConfigurations) {
return applicationConfigurations(List.of(applicationConfigurations));
}
@CustomType.Setter
public Builder artifactName(@Nullable String artifactName) {
this.artifactName = artifactName;
return this;
}
@CustomType.Setter
public Builder bindingPorts(@Nullable List bindingPorts) {
this.bindingPorts = bindingPorts;
return this;
}
public Builder bindingPorts(Integer... bindingPorts) {
return bindingPorts(List.of(bindingPorts));
}
@CustomType.Setter
public Builder buildJdkVersion(@Nullable String buildJdkVersion) {
this.buildJdkVersion = buildJdkVersion;
return this;
}
@CustomType.Setter
public Builder certificates(@Nullable List certificates) {
this.certificates = certificates;
return this;
}
public Builder certificates(String... certificates) {
return certificates(List.of(certificates));
}
@CustomType.Setter
public Builder checksum(@Nullable String checksum) {
this.checksum = checksum;
return this;
}
@CustomType.Setter
public Builder connectionStrings(@Nullable List connectionStrings) {
this.connectionStrings = connectionStrings;
return this;
}
public Builder connectionStrings(String... connectionStrings) {
return connectionStrings(List.of(connectionStrings));
}
@CustomType.Setter
public Builder dependencies(@Nullable List dependencies) {
this.dependencies = dependencies;
return this;
}
public Builder dependencies(String... dependencies) {
return dependencies(List.of(dependencies));
}
@CustomType.Setter
public Builder environments(@Nullable List environments) {
this.environments = environments;
return this;
}
public Builder environments(String... environments) {
return environments(List.of(environments));
}
@CustomType.Setter
public Builder errors(@Nullable List errors) {
this.errors = errors;
return this;
}
public Builder errors(ErrorResponse... errors) {
return errors(List.of(errors));
}
@CustomType.Setter
public Builder instanceCount(@Nullable Integer instanceCount) {
this.instanceCount = instanceCount;
return this;
}
@CustomType.Setter
public Builder instances(@Nullable List instances) {
this.instances = instances;
return this;
}
public Builder instances(SpringbootappsPropertiesResponseInstances... instances) {
return instances(List.of(instances));
}
@CustomType.Setter
public Builder jarFileLocation(@Nullable String jarFileLocation) {
this.jarFileLocation = jarFileLocation;
return this;
}
@CustomType.Setter
public Builder jvmMemoryInMB(@Nullable Integer jvmMemoryInMB) {
this.jvmMemoryInMB = jvmMemoryInMB;
return this;
}
@CustomType.Setter
public Builder jvmOptions(@Nullable List jvmOptions) {
this.jvmOptions = jvmOptions;
return this;
}
public Builder jvmOptions(String... jvmOptions) {
return jvmOptions(List.of(jvmOptions));
}
@CustomType.Setter
public Builder labels(@Nullable Map labels) {
this.labels = labels;
return this;
}
@CustomType.Setter
public Builder lastModifiedTime(@Nullable String lastModifiedTime) {
this.lastModifiedTime = lastModifiedTime;
return this;
}
@CustomType.Setter
public Builder lastUpdatedTime(@Nullable String lastUpdatedTime) {
this.lastUpdatedTime = lastUpdatedTime;
return this;
}
@CustomType.Setter
public Builder machineArmIds(@Nullable List machineArmIds) {
this.machineArmIds = machineArmIds;
return this;
}
public Builder machineArmIds(String... machineArmIds) {
return machineArmIds(List.of(machineArmIds));
}
@CustomType.Setter
public Builder miscs(@Nullable List miscs) {
this.miscs = miscs;
return this;
}
public Builder miscs(SpringbootappsPropertiesResponseMiscs... miscs) {
return miscs(List.of(miscs));
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("SpringbootappsPropertiesResponse", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder runtimeJdkVersion(@Nullable String runtimeJdkVersion) {
this.runtimeJdkVersion = runtimeJdkVersion;
return this;
}
@CustomType.Setter
public Builder servers(@Nullable List servers) {
this.servers = servers;
return this;
}
public Builder servers(String... servers) {
return servers(List.of(servers));
}
@CustomType.Setter
public Builder springBootVersion(@Nullable String springBootVersion) {
this.springBootVersion = springBootVersion;
return this;
}
@CustomType.Setter
public Builder staticContentLocations(@Nullable List staticContentLocations) {
this.staticContentLocations = staticContentLocations;
return this;
}
public Builder staticContentLocations(String... staticContentLocations) {
return staticContentLocations(List.of(staticContentLocations));
}
public SpringbootappsPropertiesResponse build() {
final var _resultValue = new SpringbootappsPropertiesResponse();
_resultValue.appName = appName;
_resultValue.appPort = appPort;
_resultValue.appType = appType;
_resultValue.applicationConfigurations = applicationConfigurations;
_resultValue.artifactName = artifactName;
_resultValue.bindingPorts = bindingPorts;
_resultValue.buildJdkVersion = buildJdkVersion;
_resultValue.certificates = certificates;
_resultValue.checksum = checksum;
_resultValue.connectionStrings = connectionStrings;
_resultValue.dependencies = dependencies;
_resultValue.environments = environments;
_resultValue.errors = errors;
_resultValue.instanceCount = instanceCount;
_resultValue.instances = instances;
_resultValue.jarFileLocation = jarFileLocation;
_resultValue.jvmMemoryInMB = jvmMemoryInMB;
_resultValue.jvmOptions = jvmOptions;
_resultValue.labels = labels;
_resultValue.lastModifiedTime = lastModifiedTime;
_resultValue.lastUpdatedTime = lastUpdatedTime;
_resultValue.machineArmIds = machineArmIds;
_resultValue.miscs = miscs;
_resultValue.provisioningState = provisioningState;
_resultValue.runtimeJdkVersion = runtimeJdkVersion;
_resultValue.servers = servers;
_resultValue.springBootVersion = springBootVersion;
_resultValue.staticContentLocations = staticContentLocations;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy