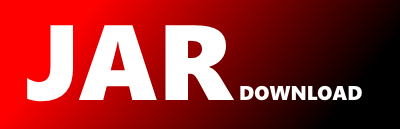
com.pulumi.azurenative.operationalinsights.Cluster Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.operationalinsights;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.operationalinsights.ClusterArgs;
import com.pulumi.azurenative.operationalinsights.outputs.AssociatedWorkspaceResponse;
import com.pulumi.azurenative.operationalinsights.outputs.CapacityReservationPropertiesResponse;
import com.pulumi.azurenative.operationalinsights.outputs.ClusterSkuResponse;
import com.pulumi.azurenative.operationalinsights.outputs.IdentityResponse;
import com.pulumi.azurenative.operationalinsights.outputs.KeyVaultPropertiesResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The top level Log Analytics cluster resource container.
* Azure REST API version: 2021-06-01. Prior API version in Azure Native 1.x: 2020-10-01.
*
* Other available API versions: 2019-08-01-preview, 2020-08-01, 2022-10-01.
*
* ## Example Usage
* ### ClustersCreate
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.operationalinsights.Cluster;
* import com.pulumi.azurenative.operationalinsights.ClusterArgs;
* import com.pulumi.azurenative.operationalinsights.inputs.ClusterSkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var cluster = new Cluster("cluster", ClusterArgs.builder()
* .clusterName("oiautorest6685")
* .location("australiasoutheast")
* .resourceGroupName("oiautorest6685")
* .sku(ClusterSkuArgs.builder()
* .capacity(1000)
* .name("CapacityReservation")
* .build())
* .tags(Map.of("tag1", "val1"))
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:operationalinsights:Cluster oiautorest6685 /subscriptions/{subscriptionId}/resourcegroups/{resourceGroupName}/providers/Microsoft.OperationalInsights/clusters/{clusterName}
* ```
*
*/
@ResourceType(type="azure-native:operationalinsights:Cluster")
public class Cluster extends com.pulumi.resources.CustomResource {
/**
* The list of Log Analytics workspaces associated with the cluster
*
*/
@Export(name="associatedWorkspaces", refs={List.class,AssociatedWorkspaceResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> associatedWorkspaces;
/**
* @return The list of Log Analytics workspaces associated with the cluster
*
*/
public Output>> associatedWorkspaces() {
return Codegen.optional(this.associatedWorkspaces);
}
/**
* The cluster's billing type.
*
*/
@Export(name="billingType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> billingType;
/**
* @return The cluster's billing type.
*
*/
public Output> billingType() {
return Codegen.optional(this.billingType);
}
/**
* Additional properties for capacity reservation
*
*/
@Export(name="capacityReservationProperties", refs={CapacityReservationPropertiesResponse.class}, tree="[0]")
private Output* @Nullable */ CapacityReservationPropertiesResponse> capacityReservationProperties;
/**
* @return Additional properties for capacity reservation
*
*/
public Output> capacityReservationProperties() {
return Codegen.optional(this.capacityReservationProperties);
}
/**
* The ID associated with the cluster.
*
*/
@Export(name="clusterId", refs={String.class}, tree="[0]")
private Output clusterId;
/**
* @return The ID associated with the cluster.
*
*/
public Output clusterId() {
return this.clusterId;
}
/**
* The cluster creation time
*
*/
@Export(name="createdDate", refs={String.class}, tree="[0]")
private Output createdDate;
/**
* @return The cluster creation time
*
*/
public Output createdDate() {
return this.createdDate;
}
/**
* The identity of the resource.
*
*/
@Export(name="identity", refs={IdentityResponse.class}, tree="[0]")
private Output* @Nullable */ IdentityResponse> identity;
/**
* @return The identity of the resource.
*
*/
public Output> identity() {
return Codegen.optional(this.identity);
}
/**
* Sets whether the cluster will support availability zones. This can be set as true only in regions where Azure Data Explorer support Availability Zones. This Property can not be modified after cluster creation. Default value is 'true' if region supports Availability Zones.
*
*/
@Export(name="isAvailabilityZonesEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> isAvailabilityZonesEnabled;
/**
* @return Sets whether the cluster will support availability zones. This can be set as true only in regions where Azure Data Explorer support Availability Zones. This Property can not be modified after cluster creation. Default value is 'true' if region supports Availability Zones.
*
*/
public Output> isAvailabilityZonesEnabled() {
return Codegen.optional(this.isAvailabilityZonesEnabled);
}
/**
* Configures whether cluster will use double encryption. This Property can not be modified after cluster creation. Default value is 'true'
*
*/
@Export(name="isDoubleEncryptionEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> isDoubleEncryptionEnabled;
/**
* @return Configures whether cluster will use double encryption. This Property can not be modified after cluster creation. Default value is 'true'
*
*/
public Output> isDoubleEncryptionEnabled() {
return Codegen.optional(this.isDoubleEncryptionEnabled);
}
/**
* The associated key properties.
*
*/
@Export(name="keyVaultProperties", refs={KeyVaultPropertiesResponse.class}, tree="[0]")
private Output* @Nullable */ KeyVaultPropertiesResponse> keyVaultProperties;
/**
* @return The associated key properties.
*
*/
public Output> keyVaultProperties() {
return Codegen.optional(this.keyVaultProperties);
}
/**
* The last time the cluster was updated.
*
*/
@Export(name="lastModifiedDate", refs={String.class}, tree="[0]")
private Output lastModifiedDate;
/**
* @return The last time the cluster was updated.
*
*/
public Output lastModifiedDate() {
return this.lastModifiedDate;
}
/**
* The geo-location where the resource lives
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return The geo-location where the resource lives
*
*/
public Output location() {
return this.location;
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* The provisioning state of the cluster.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The provisioning state of the cluster.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* The sku properties.
*
*/
@Export(name="sku", refs={ClusterSkuResponse.class}, tree="[0]")
private Output* @Nullable */ ClusterSkuResponse> sku;
/**
* @return The sku properties.
*
*/
public Output> sku() {
return Codegen.optional(this.sku);
}
/**
* Resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Cluster(java.lang.String name) {
this(name, ClusterArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Cluster(java.lang.String name, ClusterArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Cluster(java.lang.String name, ClusterArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:operationalinsights:Cluster", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Cluster(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:operationalinsights:Cluster", name, null, makeResourceOptions(options, id), false);
}
private static ClusterArgs makeArgs(ClusterArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ClusterArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:operationalinsights/v20190801preview:Cluster").build()),
Output.of(Alias.builder().type("azure-native:operationalinsights/v20200301preview:Cluster").build()),
Output.of(Alias.builder().type("azure-native:operationalinsights/v20200801:Cluster").build()),
Output.of(Alias.builder().type("azure-native:operationalinsights/v20201001:Cluster").build()),
Output.of(Alias.builder().type("azure-native:operationalinsights/v20210601:Cluster").build()),
Output.of(Alias.builder().type("azure-native:operationalinsights/v20221001:Cluster").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Cluster get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Cluster(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy