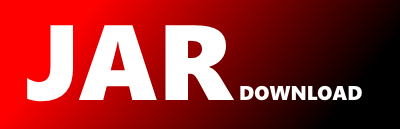
com.pulumi.azurenative.operationalinsights.SavedSearchArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.operationalinsights;
import com.pulumi.azurenative.operationalinsights.inputs.TagArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class SavedSearchArgs extends com.pulumi.resources.ResourceArgs {
public static final SavedSearchArgs Empty = new SavedSearchArgs();
/**
* The category of the saved search. This helps the user to find a saved search faster.
*
*/
@Import(name="category", required=true)
private Output category;
/**
* @return The category of the saved search. This helps the user to find a saved search faster.
*
*/
public Output category() {
return this.category;
}
/**
* Saved search display name.
*
*/
@Import(name="displayName", required=true)
private Output displayName;
/**
* @return Saved search display name.
*
*/
public Output displayName() {
return this.displayName;
}
/**
* The function alias if query serves as a function.
*
*/
@Import(name="functionAlias")
private @Nullable Output functionAlias;
/**
* @return The function alias if query serves as a function.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy