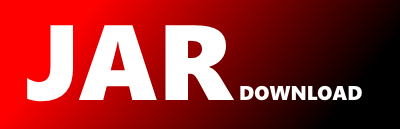
com.pulumi.azurenative.operationalinsights.outputs.GetWorkspaceResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.operationalinsights.outputs;
import com.pulumi.azurenative.operationalinsights.outputs.IdentityResponse;
import com.pulumi.azurenative.operationalinsights.outputs.PrivateLinkScopedResourceResponse;
import com.pulumi.azurenative.operationalinsights.outputs.SystemDataResponse;
import com.pulumi.azurenative.operationalinsights.outputs.WorkspaceCappingResponse;
import com.pulumi.azurenative.operationalinsights.outputs.WorkspaceFeaturesResponse;
import com.pulumi.azurenative.operationalinsights.outputs.WorkspaceSkuResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetWorkspaceResult {
/**
* @return Workspace creation date.
*
*/
private String createdDate;
/**
* @return This is a read-only property. Represents the ID associated with the workspace.
*
*/
private String customerId;
/**
* @return The resource ID of the default Data Collection Rule to use for this workspace. Expected format is - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Insights/dataCollectionRules/{dcrName}.
*
*/
private @Nullable String defaultDataCollectionRuleResourceId;
/**
* @return The etag of the workspace.
*
*/
private @Nullable String etag;
/**
* @return Workspace features.
*
*/
private @Nullable WorkspaceFeaturesResponse features;
/**
* @return Indicates whether customer managed storage is mandatory for query management.
*
*/
private @Nullable Boolean forceCmkForQuery;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The identity of the resource.
*
*/
private @Nullable IdentityResponse identity;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return Workspace modification date.
*
*/
private String modifiedDate;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return List of linked private link scope resources.
*
*/
private List privateLinkScopedResources;
/**
* @return The provisioning state of the workspace.
*
*/
private String provisioningState;
/**
* @return The network access type for accessing Log Analytics ingestion.
*
*/
private @Nullable String publicNetworkAccessForIngestion;
/**
* @return The network access type for accessing Log Analytics query.
*
*/
private @Nullable String publicNetworkAccessForQuery;
/**
* @return The workspace data retention in days. Allowed values are per pricing plan. See pricing tiers documentation for details.
*
*/
private @Nullable Integer retentionInDays;
/**
* @return The SKU of the workspace.
*
*/
private @Nullable WorkspaceSkuResponse sku;
/**
* @return Metadata pertaining to creation and last modification of the resource.
*
*/
private SystemDataResponse systemData;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
/**
* @return The daily volume cap for ingestion.
*
*/
private @Nullable WorkspaceCappingResponse workspaceCapping;
private GetWorkspaceResult() {}
/**
* @return Workspace creation date.
*
*/
public String createdDate() {
return this.createdDate;
}
/**
* @return This is a read-only property. Represents the ID associated with the workspace.
*
*/
public String customerId() {
return this.customerId;
}
/**
* @return The resource ID of the default Data Collection Rule to use for this workspace. Expected format is - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Insights/dataCollectionRules/{dcrName}.
*
*/
public Optional defaultDataCollectionRuleResourceId() {
return Optional.ofNullable(this.defaultDataCollectionRuleResourceId);
}
/**
* @return The etag of the workspace.
*
*/
public Optional etag() {
return Optional.ofNullable(this.etag);
}
/**
* @return Workspace features.
*
*/
public Optional features() {
return Optional.ofNullable(this.features);
}
/**
* @return Indicates whether customer managed storage is mandatory for query management.
*
*/
public Optional forceCmkForQuery() {
return Optional.ofNullable(this.forceCmkForQuery);
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The identity of the resource.
*
*/
public Optional identity() {
return Optional.ofNullable(this.identity);
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return Workspace modification date.
*
*/
public String modifiedDate() {
return this.modifiedDate;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return List of linked private link scope resources.
*
*/
public List privateLinkScopedResources() {
return this.privateLinkScopedResources;
}
/**
* @return The provisioning state of the workspace.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The network access type for accessing Log Analytics ingestion.
*
*/
public Optional publicNetworkAccessForIngestion() {
return Optional.ofNullable(this.publicNetworkAccessForIngestion);
}
/**
* @return The network access type for accessing Log Analytics query.
*
*/
public Optional publicNetworkAccessForQuery() {
return Optional.ofNullable(this.publicNetworkAccessForQuery);
}
/**
* @return The workspace data retention in days. Allowed values are per pricing plan. See pricing tiers documentation for details.
*
*/
public Optional retentionInDays() {
return Optional.ofNullable(this.retentionInDays);
}
/**
* @return The SKU of the workspace.
*
*/
public Optional sku() {
return Optional.ofNullable(this.sku);
}
/**
* @return Metadata pertaining to creation and last modification of the resource.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
/**
* @return The daily volume cap for ingestion.
*
*/
public Optional workspaceCapping() {
return Optional.ofNullable(this.workspaceCapping);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetWorkspaceResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String createdDate;
private String customerId;
private @Nullable String defaultDataCollectionRuleResourceId;
private @Nullable String etag;
private @Nullable WorkspaceFeaturesResponse features;
private @Nullable Boolean forceCmkForQuery;
private String id;
private @Nullable IdentityResponse identity;
private String location;
private String modifiedDate;
private String name;
private List privateLinkScopedResources;
private String provisioningState;
private @Nullable String publicNetworkAccessForIngestion;
private @Nullable String publicNetworkAccessForQuery;
private @Nullable Integer retentionInDays;
private @Nullable WorkspaceSkuResponse sku;
private SystemDataResponse systemData;
private @Nullable Map tags;
private String type;
private @Nullable WorkspaceCappingResponse workspaceCapping;
public Builder() {}
public Builder(GetWorkspaceResult defaults) {
Objects.requireNonNull(defaults);
this.createdDate = defaults.createdDate;
this.customerId = defaults.customerId;
this.defaultDataCollectionRuleResourceId = defaults.defaultDataCollectionRuleResourceId;
this.etag = defaults.etag;
this.features = defaults.features;
this.forceCmkForQuery = defaults.forceCmkForQuery;
this.id = defaults.id;
this.identity = defaults.identity;
this.location = defaults.location;
this.modifiedDate = defaults.modifiedDate;
this.name = defaults.name;
this.privateLinkScopedResources = defaults.privateLinkScopedResources;
this.provisioningState = defaults.provisioningState;
this.publicNetworkAccessForIngestion = defaults.publicNetworkAccessForIngestion;
this.publicNetworkAccessForQuery = defaults.publicNetworkAccessForQuery;
this.retentionInDays = defaults.retentionInDays;
this.sku = defaults.sku;
this.systemData = defaults.systemData;
this.tags = defaults.tags;
this.type = defaults.type;
this.workspaceCapping = defaults.workspaceCapping;
}
@CustomType.Setter
public Builder createdDate(String createdDate) {
if (createdDate == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "createdDate");
}
this.createdDate = createdDate;
return this;
}
@CustomType.Setter
public Builder customerId(String customerId) {
if (customerId == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "customerId");
}
this.customerId = customerId;
return this;
}
@CustomType.Setter
public Builder defaultDataCollectionRuleResourceId(@Nullable String defaultDataCollectionRuleResourceId) {
this.defaultDataCollectionRuleResourceId = defaultDataCollectionRuleResourceId;
return this;
}
@CustomType.Setter
public Builder etag(@Nullable String etag) {
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder features(@Nullable WorkspaceFeaturesResponse features) {
this.features = features;
return this;
}
@CustomType.Setter
public Builder forceCmkForQuery(@Nullable Boolean forceCmkForQuery) {
this.forceCmkForQuery = forceCmkForQuery;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder identity(@Nullable IdentityResponse identity) {
this.identity = identity;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder modifiedDate(String modifiedDate) {
if (modifiedDate == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "modifiedDate");
}
this.modifiedDate = modifiedDate;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder privateLinkScopedResources(List privateLinkScopedResources) {
if (privateLinkScopedResources == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "privateLinkScopedResources");
}
this.privateLinkScopedResources = privateLinkScopedResources;
return this;
}
public Builder privateLinkScopedResources(PrivateLinkScopedResourceResponse... privateLinkScopedResources) {
return privateLinkScopedResources(List.of(privateLinkScopedResources));
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder publicNetworkAccessForIngestion(@Nullable String publicNetworkAccessForIngestion) {
this.publicNetworkAccessForIngestion = publicNetworkAccessForIngestion;
return this;
}
@CustomType.Setter
public Builder publicNetworkAccessForQuery(@Nullable String publicNetworkAccessForQuery) {
this.publicNetworkAccessForQuery = publicNetworkAccessForQuery;
return this;
}
@CustomType.Setter
public Builder retentionInDays(@Nullable Integer retentionInDays) {
this.retentionInDays = retentionInDays;
return this;
}
@CustomType.Setter
public Builder sku(@Nullable WorkspaceSkuResponse sku) {
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder workspaceCapping(@Nullable WorkspaceCappingResponse workspaceCapping) {
this.workspaceCapping = workspaceCapping;
return this;
}
public GetWorkspaceResult build() {
final var _resultValue = new GetWorkspaceResult();
_resultValue.createdDate = createdDate;
_resultValue.customerId = customerId;
_resultValue.defaultDataCollectionRuleResourceId = defaultDataCollectionRuleResourceId;
_resultValue.etag = etag;
_resultValue.features = features;
_resultValue.forceCmkForQuery = forceCmkForQuery;
_resultValue.id = id;
_resultValue.identity = identity;
_resultValue.location = location;
_resultValue.modifiedDate = modifiedDate;
_resultValue.name = name;
_resultValue.privateLinkScopedResources = privateLinkScopedResources;
_resultValue.provisioningState = provisioningState;
_resultValue.publicNetworkAccessForIngestion = publicNetworkAccessForIngestion;
_resultValue.publicNetworkAccessForQuery = publicNetworkAccessForQuery;
_resultValue.retentionInDays = retentionInDays;
_resultValue.sku = sku;
_resultValue.systemData = systemData;
_resultValue.tags = tags;
_resultValue.type = type;
_resultValue.workspaceCapping = workspaceCapping;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy