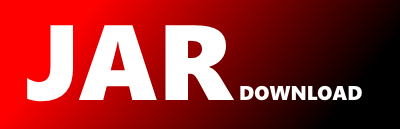
com.pulumi.azurenative.orbital.SpacecraftArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.orbital;
import com.pulumi.azurenative.orbital.inputs.SpacecraftLinkArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class SpacecraftArgs extends com.pulumi.resources.ResourceArgs {
public static final SpacecraftArgs Empty = new SpacecraftArgs();
/**
* Immutable list of Spacecraft links.
*
*/
@Import(name="links", required=true)
private Output> links;
/**
* @return Immutable list of Spacecraft links.
*
*/
public Output> links() {
return this.links;
}
/**
* The geo-location where the resource lives
*
*/
@Import(name="location")
private @Nullable Output location;
/**
* @return The geo-location where the resource lives
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy