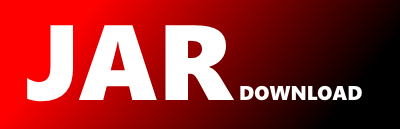
com.pulumi.azurenative.orbital.inputs.ContactProfileLinkChannelArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.orbital.inputs;
import com.pulumi.azurenative.orbital.inputs.EndPointArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Contact Profile Link Channel.
*
*/
public final class ContactProfileLinkChannelArgs extends com.pulumi.resources.ResourceArgs {
public static final ContactProfileLinkChannelArgs Empty = new ContactProfileLinkChannelArgs();
/**
* Bandwidth in MHz.
*
*/
@Import(name="bandwidthMHz", required=true)
private Output bandwidthMHz;
/**
* @return Bandwidth in MHz.
*
*/
public Output bandwidthMHz() {
return this.bandwidthMHz;
}
/**
* Center Frequency in MHz.
*
*/
@Import(name="centerFrequencyMHz", required=true)
private Output centerFrequencyMHz;
/**
* @return Center Frequency in MHz.
*
*/
public Output centerFrequencyMHz() {
return this.centerFrequencyMHz;
}
/**
* Currently unused.
*
*/
@Import(name="decodingConfiguration")
private @Nullable Output decodingConfiguration;
/**
* @return Currently unused.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy