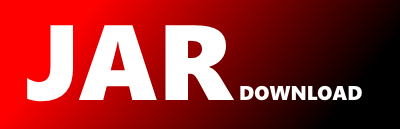
com.pulumi.azurenative.orbital.outputs.GetContactResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.orbital.outputs;
import com.pulumi.azurenative.orbital.outputs.ContactsPropertiesResponseAntennaConfiguration;
import com.pulumi.azurenative.orbital.outputs.ContactsPropertiesResponseContactProfile;
import com.pulumi.azurenative.orbital.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class GetContactResult {
/**
* @return The configuration associated with the allocated antenna.
*
*/
private ContactsPropertiesResponseAntennaConfiguration antennaConfiguration;
/**
* @return The reference to the contact profile resource.
*
*/
private ContactsPropertiesResponseContactProfile contactProfile;
/**
* @return Azimuth of the antenna at the end of the contact in decimal degrees.
*
*/
private Double endAzimuthDegrees;
/**
* @return Spacecraft elevation above the horizon at contact end.
*
*/
private Double endElevationDegrees;
/**
* @return Any error message while scheduling a contact.
*
*/
private String errorMessage;
/**
* @return Azure Ground Station name.
*
*/
private String groundStationName;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return Maximum elevation of the antenna during the contact in decimal degrees.
*
*/
private Double maximumElevationDegrees;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Reservation end time of a contact (ISO 8601 UTC standard).
*
*/
private String reservationEndTime;
/**
* @return Reservation start time of a contact (ISO 8601 UTC standard).
*
*/
private String reservationStartTime;
/**
* @return Receive end time of a contact (ISO 8601 UTC standard).
*
*/
private String rxEndTime;
/**
* @return Receive start time of a contact (ISO 8601 UTC standard).
*
*/
private String rxStartTime;
/**
* @return Azimuth of the antenna at the start of the contact in decimal degrees.
*
*/
private Double startAzimuthDegrees;
/**
* @return Spacecraft elevation above the horizon at contact start.
*
*/
private Double startElevationDegrees;
/**
* @return Status of a contact.
*
*/
private String status;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return Transmit end time of a contact (ISO 8601 UTC standard).
*
*/
private String txEndTime;
/**
* @return Transmit start time of a contact (ISO 8601 UTC standard).
*
*/
private String txStartTime;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetContactResult() {}
/**
* @return The configuration associated with the allocated antenna.
*
*/
public ContactsPropertiesResponseAntennaConfiguration antennaConfiguration() {
return this.antennaConfiguration;
}
/**
* @return The reference to the contact profile resource.
*
*/
public ContactsPropertiesResponseContactProfile contactProfile() {
return this.contactProfile;
}
/**
* @return Azimuth of the antenna at the end of the contact in decimal degrees.
*
*/
public Double endAzimuthDegrees() {
return this.endAzimuthDegrees;
}
/**
* @return Spacecraft elevation above the horizon at contact end.
*
*/
public Double endElevationDegrees() {
return this.endElevationDegrees;
}
/**
* @return Any error message while scheduling a contact.
*
*/
public String errorMessage() {
return this.errorMessage;
}
/**
* @return Azure Ground Station name.
*
*/
public String groundStationName() {
return this.groundStationName;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return Maximum elevation of the antenna during the contact in decimal degrees.
*
*/
public Double maximumElevationDegrees() {
return this.maximumElevationDegrees;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Reservation end time of a contact (ISO 8601 UTC standard).
*
*/
public String reservationEndTime() {
return this.reservationEndTime;
}
/**
* @return Reservation start time of a contact (ISO 8601 UTC standard).
*
*/
public String reservationStartTime() {
return this.reservationStartTime;
}
/**
* @return Receive end time of a contact (ISO 8601 UTC standard).
*
*/
public String rxEndTime() {
return this.rxEndTime;
}
/**
* @return Receive start time of a contact (ISO 8601 UTC standard).
*
*/
public String rxStartTime() {
return this.rxStartTime;
}
/**
* @return Azimuth of the antenna at the start of the contact in decimal degrees.
*
*/
public Double startAzimuthDegrees() {
return this.startAzimuthDegrees;
}
/**
* @return Spacecraft elevation above the horizon at contact start.
*
*/
public Double startElevationDegrees() {
return this.startElevationDegrees;
}
/**
* @return Status of a contact.
*
*/
public String status() {
return this.status;
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Transmit end time of a contact (ISO 8601 UTC standard).
*
*/
public String txEndTime() {
return this.txEndTime;
}
/**
* @return Transmit start time of a contact (ISO 8601 UTC standard).
*
*/
public String txStartTime() {
return this.txStartTime;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetContactResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private ContactsPropertiesResponseAntennaConfiguration antennaConfiguration;
private ContactsPropertiesResponseContactProfile contactProfile;
private Double endAzimuthDegrees;
private Double endElevationDegrees;
private String errorMessage;
private String groundStationName;
private String id;
private Double maximumElevationDegrees;
private String name;
private String reservationEndTime;
private String reservationStartTime;
private String rxEndTime;
private String rxStartTime;
private Double startAzimuthDegrees;
private Double startElevationDegrees;
private String status;
private SystemDataResponse systemData;
private String txEndTime;
private String txStartTime;
private String type;
public Builder() {}
public Builder(GetContactResult defaults) {
Objects.requireNonNull(defaults);
this.antennaConfiguration = defaults.antennaConfiguration;
this.contactProfile = defaults.contactProfile;
this.endAzimuthDegrees = defaults.endAzimuthDegrees;
this.endElevationDegrees = defaults.endElevationDegrees;
this.errorMessage = defaults.errorMessage;
this.groundStationName = defaults.groundStationName;
this.id = defaults.id;
this.maximumElevationDegrees = defaults.maximumElevationDegrees;
this.name = defaults.name;
this.reservationEndTime = defaults.reservationEndTime;
this.reservationStartTime = defaults.reservationStartTime;
this.rxEndTime = defaults.rxEndTime;
this.rxStartTime = defaults.rxStartTime;
this.startAzimuthDegrees = defaults.startAzimuthDegrees;
this.startElevationDegrees = defaults.startElevationDegrees;
this.status = defaults.status;
this.systemData = defaults.systemData;
this.txEndTime = defaults.txEndTime;
this.txStartTime = defaults.txStartTime;
this.type = defaults.type;
}
@CustomType.Setter
public Builder antennaConfiguration(ContactsPropertiesResponseAntennaConfiguration antennaConfiguration) {
if (antennaConfiguration == null) {
throw new MissingRequiredPropertyException("GetContactResult", "antennaConfiguration");
}
this.antennaConfiguration = antennaConfiguration;
return this;
}
@CustomType.Setter
public Builder contactProfile(ContactsPropertiesResponseContactProfile contactProfile) {
if (contactProfile == null) {
throw new MissingRequiredPropertyException("GetContactResult", "contactProfile");
}
this.contactProfile = contactProfile;
return this;
}
@CustomType.Setter
public Builder endAzimuthDegrees(Double endAzimuthDegrees) {
if (endAzimuthDegrees == null) {
throw new MissingRequiredPropertyException("GetContactResult", "endAzimuthDegrees");
}
this.endAzimuthDegrees = endAzimuthDegrees;
return this;
}
@CustomType.Setter
public Builder endElevationDegrees(Double endElevationDegrees) {
if (endElevationDegrees == null) {
throw new MissingRequiredPropertyException("GetContactResult", "endElevationDegrees");
}
this.endElevationDegrees = endElevationDegrees;
return this;
}
@CustomType.Setter
public Builder errorMessage(String errorMessage) {
if (errorMessage == null) {
throw new MissingRequiredPropertyException("GetContactResult", "errorMessage");
}
this.errorMessage = errorMessage;
return this;
}
@CustomType.Setter
public Builder groundStationName(String groundStationName) {
if (groundStationName == null) {
throw new MissingRequiredPropertyException("GetContactResult", "groundStationName");
}
this.groundStationName = groundStationName;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetContactResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder maximumElevationDegrees(Double maximumElevationDegrees) {
if (maximumElevationDegrees == null) {
throw new MissingRequiredPropertyException("GetContactResult", "maximumElevationDegrees");
}
this.maximumElevationDegrees = maximumElevationDegrees;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetContactResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder reservationEndTime(String reservationEndTime) {
if (reservationEndTime == null) {
throw new MissingRequiredPropertyException("GetContactResult", "reservationEndTime");
}
this.reservationEndTime = reservationEndTime;
return this;
}
@CustomType.Setter
public Builder reservationStartTime(String reservationStartTime) {
if (reservationStartTime == null) {
throw new MissingRequiredPropertyException("GetContactResult", "reservationStartTime");
}
this.reservationStartTime = reservationStartTime;
return this;
}
@CustomType.Setter
public Builder rxEndTime(String rxEndTime) {
if (rxEndTime == null) {
throw new MissingRequiredPropertyException("GetContactResult", "rxEndTime");
}
this.rxEndTime = rxEndTime;
return this;
}
@CustomType.Setter
public Builder rxStartTime(String rxStartTime) {
if (rxStartTime == null) {
throw new MissingRequiredPropertyException("GetContactResult", "rxStartTime");
}
this.rxStartTime = rxStartTime;
return this;
}
@CustomType.Setter
public Builder startAzimuthDegrees(Double startAzimuthDegrees) {
if (startAzimuthDegrees == null) {
throw new MissingRequiredPropertyException("GetContactResult", "startAzimuthDegrees");
}
this.startAzimuthDegrees = startAzimuthDegrees;
return this;
}
@CustomType.Setter
public Builder startElevationDegrees(Double startElevationDegrees) {
if (startElevationDegrees == null) {
throw new MissingRequiredPropertyException("GetContactResult", "startElevationDegrees");
}
this.startElevationDegrees = startElevationDegrees;
return this;
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("GetContactResult", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetContactResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder txEndTime(String txEndTime) {
if (txEndTime == null) {
throw new MissingRequiredPropertyException("GetContactResult", "txEndTime");
}
this.txEndTime = txEndTime;
return this;
}
@CustomType.Setter
public Builder txStartTime(String txStartTime) {
if (txStartTime == null) {
throw new MissingRequiredPropertyException("GetContactResult", "txStartTime");
}
this.txStartTime = txStartTime;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetContactResult", "type");
}
this.type = type;
return this;
}
public GetContactResult build() {
final var _resultValue = new GetContactResult();
_resultValue.antennaConfiguration = antennaConfiguration;
_resultValue.contactProfile = contactProfile;
_resultValue.endAzimuthDegrees = endAzimuthDegrees;
_resultValue.endElevationDegrees = endElevationDegrees;
_resultValue.errorMessage = errorMessage;
_resultValue.groundStationName = groundStationName;
_resultValue.id = id;
_resultValue.maximumElevationDegrees = maximumElevationDegrees;
_resultValue.name = name;
_resultValue.reservationEndTime = reservationEndTime;
_resultValue.reservationStartTime = reservationStartTime;
_resultValue.rxEndTime = rxEndTime;
_resultValue.rxStartTime = rxStartTime;
_resultValue.startAzimuthDegrees = startAzimuthDegrees;
_resultValue.startElevationDegrees = startElevationDegrees;
_resultValue.status = status;
_resultValue.systemData = systemData;
_resultValue.txEndTime = txEndTime;
_resultValue.txStartTime = txStartTime;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy